How to Solve Python Tuple Index Error : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
To tackle the IndexError: tuple index out of range
in Python, ensure that you access tuple elements within their valid index range, which starts from 0 up to one less than the length of the tuple. Utilize the len()
function to dynamically determine the tuple’s length and guide your access patterns, or employ error handling with try
and except
blocks to gracefully manage attempts to access non-existent indices.
Broken Example
Consider a tuple example_tuple = (10, 20, 30)
. Attempting to access example_tuple[3]
triggers an IndexError
because the indices here range from 0 to 2, making 3 out of bounds.
example_tuple = (10, 20, 30 print(example_tuple[3]) # This will raise an IndexError: tuple index out of range
Fixed Example
To access the last element safely, either use the correct index directly, if known, or calculate it dynamically using len(example_tuple) - 1
.
example_tuple = (10, 20, 30) print(example_tuple[2]) # Correctly accesses the last element, 30 # Or dynamically: print(example_tuple[len(example_tuple) - 1]) # Also accesses the last element, 30
FAQ on Tuple Index Out of Range Error
Q: What if I’m not sure about the tuple’s length?
A: Use the len()
function to find out the tuple’s length. This way, you can dynamically adjust your code to access elements without going out of bounds.
Q: How can I access the last element of a tuple without knowing its length?
A: Use the index -1
. Python supports negative indexing, where -1
refers to the last element, -2
to the second last, and so on.
example_tuple = (10, 20, 30) print(example_tuple[-1]) # Outputs 30, the last element
Q: What’s the best way to avoid the IndexError
when iterating over a tuple?
A: Use a for
loop that iterates directly over the tuple elements, or use range(len(tuple))
to ensure your indices are within bounds.
example_tuple = (10, 20, 30) for item in example_tuple: print(item) # Safely iterates over each element
Q: Can I use error handling to manage out-of-range errors?
A: Yes, wrapping your access attempts in a try
block with an except IndexError
clause allows your program to handle the error gracefully.
example_tuple = (10, 20, 30) try: print(example_tuple[3]) except IndexError: print("Attempted to access an element outside the tuple's range.")
Q: Is there a way to safely access elements within a tuple without risking an IndexError
?
A: Besides using correct indices and error handling, you can also use the get()
method from the operator
module, which allows safe access with a default value if the index is out of range. However, remember that tuples are meant to be accessed directly via indices or iterated over; if you find yourself needing get()
frequently, consider whether a different data structure, like a list or a dictionary, might suit your needs better.
5 Common Reasons For The Python Tuple Index Out of Range Error
- Reason 1: Attempting to access an element beyond the tuple’s length, e.g.,
my_tuple = (1, 2, 3); print(my_tuple[3])
. - Reason 2: Miscounting the tuple’s starting index, forgetting that Python indices start at 0, not 1.
- Reason 3: Dynamically accessing tuple elements without checking the tuple’s current size with
len(my_tuple)
. - Reason 4: Using a variable as an index without ensuring it falls within the valid range of the tuple’s indices.
- Reason 5: Iterating over a range that exceeds the tuple’s length instead of directly iterating over the tuple itself.
Related article: Python Tuple Index Out of Range Error – How to Fix?
April 05, 2024 at 09:12PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
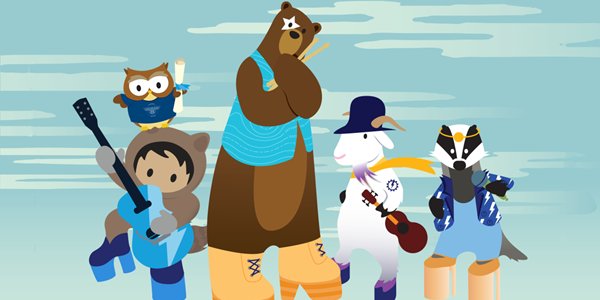
Post a Comment