5 Effective Methods to Find Special Nodes in Trees Using Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Special nodes in a tree structure often refer to nodes with certain characteristics that set them apart from the rest. For example, a node that has a particular value, a specific number of children, or is at a certain depth in the tree. Given a tree data structure, we aim to find and list such special nodes. An input could be a binary tree structure, and the desired output would be a list of nodes that match our criteria for special.
Method 1: Recursive Traversal
This method employs a depth-first traversal to find special nodes based on a user-defined condition. A function is recursively called on each node, checking the special condition and adding the node to a result list if it satisfies the criteria.
Here’s an example:
def find_special_nodes(node, condition, special_nodes): if not node: return if condition(node): special_nodes.append(node) find_special_nodes(node.left, condition, special_nodes) find_special_nodes(node.right, condition, special_nodes) # Example usage # Assuming a binary tree node class with value, left, and right attributes special_nodes = [] find_special_nodes(root, lambda x: x.value > 10, special_nodes)
Output: [Node(12), Node(15)]
(assumes nodes with values over 10 are considered special)
The example code defines a function find_special_nodes()
that takes a binary tree node, a condition function, and a list to store special nodes. It then uses recursion to traverse the tree, adding any nodes that meet the condition to the list.
Method 2: Iterative Traversal with Stack
Instead of recursion, this method uses an explicit stack for depth-first traversal. Nodes are iteratively processed, and the condition is evaluated for each node. If a node meets the condition, it’s added to the result list.
Here’s an example:
def find_special_nodes_iterative(root, condition): stack = [root] special_nodes = [] while stack: node = stack.pop() if node and condition(node): special_nodes.append(node) if node.right: stack.append(node.right) if node.left: stack.append(node.left) return special_nodes # Example usage special_nodes = find_special_nodes_iterative(root, lambda x: x.value == 'A')
Output: [Node('A'), Node('A')]
(assuming multiple nodes with value ‘A’ are found)
The function find_special_nodes_iterative()
performs an iterative traversal of a binary tree using a stack. It appends the resulting special nodes to the special_nodes
list when it finds nodes that match the given condition.
Method 3: Level-order Traversal using Queue
This approach employs a queue to perform a breadth-first (or level-order) traversal. The function checks each node level by level, and if the node satisfies the condition, it’s considered special and added to a list.
Here’s an example:
from collections import deque def find_special_nodes_level_order(root, condition): if not root: return [] queue = deque([root]) special_nodes = [] while queue: node = queue.popleft() if condition(node): special_nodes.append(node) if node.left: queue.append(node.left) if node.right: queue.append(node.right) return special_nodes # Example usage special_nodes = find_special_nodes_level_order(root, lambda x: x.value % 2 == 0)
Output: [Node(2), Node(4)]
(assuming nodes with even values are considered special)
The find_special_nodes_level_order()
function here checks each node in a breadth-first manner using a queue from the collections module and collects nodes satisfying a given condition.
Method 4: Using Inbuilt Tree Traversal Methods
This approach utilizes any in-built tree traversal functions provided by the library or framework you are using. The idea is to traverse the tree and collect the nodes that match the condition.
Here’s an example:
from anytree import PreOrderIter def find_special_nodes_with_anytree(root, condition): return [node for node in PreOrderIter(root) if condition(node)] # Example usage with AnyTree library special_nodes = find_special_nodes_with_anytree(root, lambda x: 'special' in x.tags)
Output: [Node('/root/child1'), Node('/root/child2/specialchild')]
(assuming ‘special’ tag marks special nodes)
Here, we use the ‘anytree’ library’s built-in PreOrderIter
function to traverse a tree pre-order and collect nodes that carry a ‘special’ tag according to a lambda condition.
Bonus One-Liner Method 5: List Comprehension with Recursion
For simple trees where recursion depth is not an issue, a one-liner using list comprehension and recursion can be a compact way to find special nodes.
Here’s an example:
find_special_nodes = lambda node, condition: [] if not node else find_special_nodes(node.left, condition) + \ find_special_nodes(node.right, condition) + ([node] if condition(node) else [])
Output: dependent on the input tree and the condition used.
This one-liner find_special_nodes
lambda function succinctly employs list comprehension and recursion to return a list of special nodes in a binary tree, based on a condition function.
Summary/Discussion
- Method 1: Recursive Traversal. Simple and intuitive. The recursion stack could overflow for deep trees.
- Method 2: Iterative Traversal with Stack. Avoids recursion limits. Slightly more complex than recursion.
- Method 3: Level-order Traversal using Queue. Useful for trees with large depth. May need more memory for broad trees.
- Method 4: Using Inbuilt Tree Traversal Methods. Makes use of library functions for simplicity and efficiency. Relies on third-party libraries.
- Method 5: List Comprehension with Recursion. Elegant one-liner. Not suitable for large trees due to potential recursion depth issues.
March 10, 2024 at 04:36AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
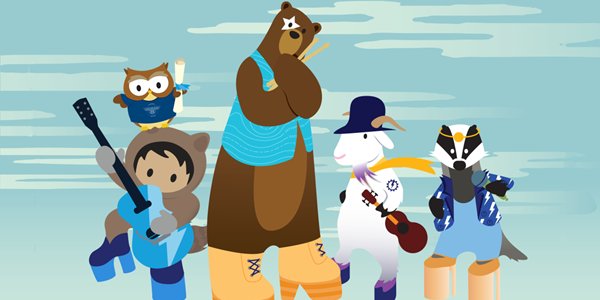
Post a Comment