5 Best Ways to Plot Google Maps Using gmplot Package in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: You need to display geographic data on a Google Map in a Python application. How can you do so programmatically while still having the ability to customize markers, lines, and other features? Imagine you’re provided with latitudes and longitudes and wish to visualize these points on a map for analysis or presentation.
Method 1: Plotting Single Location Marker
This method simply places a marker on a Google Map using the gmplot package. The GoogleMapPlotter
class is used to create a map object and the marker()
method places a marker at specified latitude and longitude. This is perfect for pinpointing a single location on the map.
Here’s an example:
from gmplot import GoogleMapPlotter # Create a map object at a specific location gmap = GoogleMapPlotter(37.428, -122.145, 16) # Place a marker gmap.marker(37.428, -122.145, 'cornflowerblue') # Generate HTML map file gmap.draw("my_map.html")
This code generates an HTML file named “my_map.html” with a Google Map showing a single marker at the defined coordinates.
In the given snippet, we’ve instantiated a GoogleMapPlotter
object for a specific zoom and coordinates, added a single ‘cornflowerblue’ marker, and drawn it out to an HTML file. This way, the marker appears when the HTML file is opened in a browser.
Method 2: Drawing a Line Between Points
To draw a line between multiple points, use plot()
method on the map object. The method takes two array-like sequences of latitudes and longitudes, alongside a color and edge width. This is great for tracing routes or paths.
Here’s an example:
from gmplot import GoogleMapPlotter # Create map object gmap = GoogleMapPlotter(37.428, -122.145, 16) # Latitude and longitude points latitudes = [37.428, 37.429] longitudes = [-122.145, -122.146] # Draw a line between given points gmap.plot(latitudes, longitudes, 'cornflowerblue', edge_width=10) # Generate the map gmap.draw("my_map.html")
This code results in “my_map.html” where a line is drawn between the defined latitude and longitude points.
Here, we’ve created an overlay line on the map using the plot()
method, which draws the line in ‘cornflowerblue’ and an edge width of 10. This visualizes the connection or path between given geographic points.
Method 3: Adding Polygons
The gmplot
package allows drawing of polygons with the polygon()
method. The parameters are similar to plot()
, but it fills the area enclosed by lines. This is ideal for highlighting areas on a map.
Here’s an example:
from gmplot import GoogleMapPlotter # New map instance gmap = GoogleMapPlotter(37.428, -122.145, 16) # Define polygon points polygon_latitudes = [37.428, 37.428, 37.429, 37.429] polygon_longitudes = [-122.145, -122.146, -122.146, -122.145] # Draw the polygon gmap.polygon(polygon_latitudes, polygon_longitudes, color='cornflowerblue') # Output the map gmap.draw("my_polygon_map.html")
“my_polygon_map.html” includes a highlighted polygon covering the area defined by the points in the lists.
This code outlines the usage of the polygon()
method to create filled-in areas on the map, using an array of latitude and longitude points which mark the vertices of the polygon.
Method 4: Heatmaps
The gmplot package also supports plotting heatmaps using the heatmap()
method. It takes latitude and longitude points to show density or intensity in different areas, perfect for visualizing data concentration.
Here’s an example:
from gmplot import GoogleMapPlotter # Start a new map gmap = GoogleMapPlotter(37.428, -122.145, 13) # Coordinates for the heatmap lats = [37.428 + i * 0.001 for i in range(10)] lons = [-122.145 + i * 0.001 for i in range(10)] # Create heatmap gmap.heatmap(lats, lons) # Save to HTML gmap.draw("my_heatmap.html")
The produced “my_heatmap.html” will show a heatmap based on the density of the provided points.
By employing heatmap()
, we’ve injected a visual representation of data density into the map, which can tell a deeper story about the distribution of a dataset across a geographic area.
Bonus One-Liner Method 5: Quick Scatter Plot
For a swift scatter plot to represent numerous points, the scatter()
method can be used. It’s ideal for quickly placing multiple markers without much customization.
Here’s an example:
from gmplot import GoogleMapPlotter # Setup map gmap = GoogleMapPlotter(37.428, -122.145, 13) # Scatter points gmap.scatter([37.428, 37.429], [-122.145, -122.146], '#3B0B39', size=40, marker=False) # Save to file gmap.draw("my_scatter.html")
Execution results in an HTML file “my_scatter.html” displaying scattered points on the map.
This one-liner lets you quickly visualize multiple data points on your map. By using scatter()
, we bypass the need for markers, instead opting for simple, colored dots on the map, sized as specified.
Summary/Discussion
- Method 1: Single Location Marker. Simple and straightforward. Cannot represent multiple points without additional code.
- Method 2: Drawing Lines Between Points. Connects multiple locations creating a path. Not suitable for non-linear or complex data representations.
- Method 3: Adding Polygons. Useful for highlighting areas. Requires knowing the coordinates of all vertices, which might not be trivial for complex shapes.
- Method 4: Heatmaps. Excellent for visualizing intensity or density information. The resulting visual can become confusing if too many data points overlap.
- Method 5: Quick Scatter Plot. Speedy data points plotting. Does not involve detailed marker customization, hence more basic in its representation.
March 12, 2024 at 03:56AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
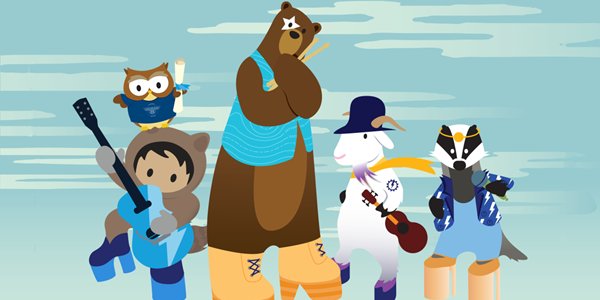
Post a Comment