5 Best Ways to Get Column Values Based on Condition in Selenium with Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Automating web data extraction can be complex, especially when dealing with HTML tables. You want to retrieve all values from a specific column in a web table when they meet certain conditions using Selenium with Python. For example, from a table of products, you might want to extract all prices that are higher than $100. This article demonstrates how to accomplish this task with different methods.
Method 1: Using Selenium WebDriver to Iterate Through Rows
This method involves using Selenium’s WebDriver in Python to iterate over each row in the HTML table and select the column value if it meets the condition. The find_elements_by_xpath()
function is typically used to locate the table elements.
Here’s an example:
from selenium import webdriver driver = webdriver.Chrome() driver.get('http://example.com/products') prices = [] for row in driver.find_elements_by_xpath('//table/tbody/tr'): price = row.find_element_by_xpath('./td[3]').text # Assuming prices are in the third column if price > 100: prices.append(price) driver.quit()
Output: A list of all prices from the third column of the table on the webpage which are greater than $100.
This code snippet sets up a Selenium WebDriver, navigates to the desired web page, and creates an empty list called prices
. It then iterates through each row in the table, checks if the price is greater than $100, and appends the price to the list if the condition is satisfied. Lastly, the browser is closed using driver.quit()
.
Method 2: Filtering With CSS Selectors
This method uses CSS selectors to target the specific column and apply the condition directly in the selector string. Selenium has the find_elements_by_css_selector()
method which is very efficient for this purpose.
Here’s an example:
from selenium import webdriver driver = webdriver.Chrome() driver.get('http://example.com/products') prices = [price.text for price in driver.find_elements_by_css_selector('table tr td:nth-child(3):not([data-price="100"])')] driver.quit()
Output: A list of prices excluding any that are exactly $100.
This snippet uses a CSS Selector to target all third column cells that do not have a data attribute equal to 100. It loops through these selected elements, gets their text content, and adds it to the list prices
. As with the previous method, it then closes the browser.
Method 3: Using XPath Functions
XPath provides a powerful way to navigate nodes in an XML document’s tree structure. When using Selenium with Python, XPath can be particularly useful to identify elements that match a specific condition by combining path expressions with functions.
Here’s an example:
from selenium import webdriver driver = webdriver.Chrome() driver.get('http://example.com/products') prices = driver.find_elements_by_xpath('//table/tbody/tr/td[3][number(.) > 100]') profits = [price.text for price in prices] driver.quit()
Output: List of prices that are numbers greater than $100.
In this example, the XPath expression //table/tbody/tr/td[3][number(.) > 100]
identifies all the third column cells where the content is a number greater than 100. It uses the number()
function to cast the cell content to a numerical value for the comparison. The resulting nodes are iterated over to create a list of text values which represent extracted profits.
Method 4: Combining Selenium with Pandas
For those who prefer working with data in tabular form, Selenium can be combined with the Pandas library to first extract the entire table into a DataFrame and then apply conditions to filter the DataFrame accordingly.
Here’s an example:
import pandas as pd from selenium import webdriver driver = webdriver.Chrome() driver.get('http://example.com/products') table_data = driver.find_element_by_xpath('//table').get_attribute('outerHTML') df = pd.read_html(table_data)[0] filtered_prices = df[df['Price'] > 100]['Price'].tolist() driver.quit()
Output: A list of prices from the ‘Price’ column in the DataFrame that are greater than $100.
After finding the table element, this script retrieves the HTML of the table and uses Pandas to read it into a DataFrame. It then applies a condition to filter the DataFrame and extracts the values of interest. The .tolist()
method is used to convert the resulting Pandas Series into a Python list.
Bonus One-Liner Method 5: List Comprehension with Conditions
A simple yet powerful one-liner in Python, list comprehension allows for terse and efficient filtering directly in the creation of a list. This is Python’s syntactic sugar and can be leveraged in conjunction with Selenium.
Here’s an example:
from selenium import webdriver driver = webdriver.Chrome() driver.get('http://example.com/products') prices = [e.text for e in driver.find_elements_by_xpath('//table/tbody/tr/td[3]') if float(e.text.replace('$', '')) > 100] driver.quit()
Output: A list of the textual content of all third-column cells with values greater than $100, with the $ symbol stripped.
This compact code utilizes a list comprehension to iterate over the elements returned by an XPath query. The if-clause inside the list comprehension processes each text value, stripping the dollar sign and converting the result to a float before comparing it to 100, all in a single line.
Summary/Discussion
Method 1: Iterating Through Rows. Straightforward. It can be slow for large tables.
Method 2: CSS Selectors. Elegant and potentially faster. May not handle complex conditions easily.
Method 3: XPath Functions. Very flexible. Can get complicated and hard to maintain.
Method 4: Selenium with Pandas. Great for complex data processing. Introduces additional dependency on Pandas.
Method 5: List Comprehension with Conditions. Compact and Pythonic. May not be as readable for those unfamiliar with list comprehensions.
March 12, 2024 at 03:57AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
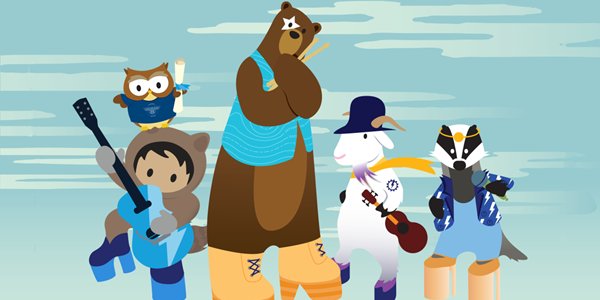
Post a Comment