5 Best Ways to Convert Python CSV Bytes to JSON : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Developers often encounter the need to convert CSV data retrieved in byte format to a JSON structure. This conversion can be critical for tasks such as data processing in web services or applications that require JSON format for interoperability. Suppose we have CSV data in bytes, for example,
b'Name,Age\\nAlice,30\\nBob,25'
and we want to convert it to a JSON format like [{"Name": "Alice", "Age": "30"}, {"Name": "Bob", "Age": "25"}]
.
Method 1: Using the csv and json Modules
The csv and json modules in Python provide a straightforward way to read CSV bytes, parse them, and then serialize the parsed data to JSON. This method involves reading the bytes using a StringIO
object, parsing the CSV data with csv.DictReader
, and finally converting it to a list of dictionaries that can be easily serialized to JSON with json.dumps()
.
Here’s an example:
import csv import json from io import StringIO # CSV data in bytes csv_bytes = b'Name,Age\\nAlice,30\\nBob,25' # Convert bytes to string and read into DictReader reader = csv.DictReader(StringIO(csv_bytes.decode('utf-8'))) # Convert to list of dictionaries dict_list = [row for row in reader] # Serialize list of dictionaries to JSON json_data = json.dumps(dict_list, indent=2) print(json_data)
The output of this code snippet is:
[ { "Name": "Alice", "Age": "30" }, { "Name": "Bob", "Age": "25" } ]
This code snippet converts CSV bytes to a string, reads the data into a DictReader
which parses each row into a dictionary, and finally dumps the list of dictionaries into a pretty-printed JSON string.
Method 2: Using pandas with BytesIO
The pandas library is a powerful data manipulation tool that can read CSV data from bytes and convert it to a DataFrame. Once you have the data in a DataFrame, pandas can directly output it to a JSON format using the to_json()
method. Utilizing BytesIO
allows pandas to read the byte stream directly.
Here’s an example:
import pandas as pd from io import BytesIO # CSV data in bytes csv_bytes = b'Name,Age\\nAlice,30\\nBob,25' # Use BytesIO to read the byte stream dataframe = pd.read_csv(BytesIO(csv_bytes)) # Convert DataFrame to JSON json_data = dataframe.to_json(orient='records', indent=2) print(json_data)
The output of this code snippet is:
[ { "Name": "Alice", "Age": 30 }, { "Name": "Bob", "Age": 25 } ]
This code snippet uses pandas to read CSV bytes into a DataFrame using BytesIO
and directly converts it to a JSON string representation with the to_json()
method. This method is very concise and powerful but requires the pandas library, which can be heavy for small tasks.
Method 3: Using Openpyxl for Excel Files
If the CSV bytes represent an Excel file, the openpyxl module can be used to convert Excel binary data to JSON. This is particularly useful when dealing with CSV data from .xlsx files. The module reads the Excel file into a workbook object, iterates over the rows, and then constructs a list of dictionaries that is converted to JSON.
Here’s an example:
import json from openpyxl import load_workbook from io import BytesIO # Excel file in bytes (represents CSV data) xlsx_bytes = b'excel-binary-data' # Read Excel file wb = load_workbook(filename=BytesIO(xlsx_bytes)) sheet = wb.active # Extract data and convert to list of dictionaries data = [] for row in sheet.iter_rows(min_row=2, values_only=True): # Assuming first row is the header data.append({'Name': row[0], 'Age': row[1]}) # Convert to JSON json_data = json.dumps(data, indent=2) print(json_data)
The output would be similar to JSON data presented in previous methods, depending on the actual content of the Excel file represented by xlsx_bytes
.
This snippet relies on openpyxl to handle Excel files, reading the binary content with BytesIO
, extracting the relevant data and converting it to JSON. However, this method specifically applies to Excel formats, not plain CSV files.
Method 4: Custom Parsing Function
When libraries are not available or you need a customized parsing approach, writing your own function to parse CSV bytes can do the trick. This method involves manual parsing of bytes for CSV data, including handling line breaks and splitting on the delimiter to create a list of dictionaries.
Here’s an example:
import json # CSV data in bytes csv_bytes = b'Name,Age\\nAlice,30\\nBob,25' # Custom parser def parse_csv_bytes(csv_bytes): lines = csv_bytes.decode('utf-8').split('\\n') header = lines[0].split(',') data = [dict(zip(header, line.split(','))) for line in lines[1:] if line] return data # Convert to JSON json_data = json.dumps(parse_csv_bytes(csv_bytes), indent=2) print(json_data)
The output of this code snippet will match the JSON output shown in earlier methods, based on the input format specified.
This snippet demonstrates how a function parse_csv_bytes
efficiently breaks down the byte string into lines, extracts headers, and constructs a list of dictionaries which is then converted to JSON format. It’s a more hands-on approach and can be modified to fit very specific parsing needs.
Bonus One-Liner Method 5: Using List Comprehension with StringIO
If the CSV is simple and doesn’t require the robustness of csv.DictReader, a one-liner using StringIO
and list comprehension can convert the bytes to JSON. However, this method assumes the first line contains the headers and the rest are data entries.
Here’s an example:
import json from io import StringIO # CSV data in bytes csv_bytes = b'Name,Age\\nAlice,30\\nBob,25' # One-liner conversion json_data = json.dumps([dict(zip(*(line.split(',') for line in StringIO(csv_bytes.decode('utf-8')).read().split('\\n'))))] , indent=2) print(json_data)
The output would be the JSON array of objects as demonstrated in previous examples.
This one-liner unpacks the CSV into a list of headers and corresponding data rows, then maps each row to a dictionary creating a JSON struct. It’s succinct but not as readable or flexible when dealing with complex CSV data.
Summary/Discussion
- Method 1: Using the csv and json Modules. Strengths: Part of the Python standard library, robust parsing. Weaknesses: More verbose than other methods.
- Method 2: Using pandas with BytesIO. Strengths: Concise and utilizes powerful data handling capabilities of pandas. Weaknesses: Requires external library, not ideal for lightweight applications.
- Method 3: Using Openpyxl for Excel Files. Strengths: Handles Excel formatted binary CSV data well. Weaknesses: Inapplicable for non-Excel CSV files and requires an external library.
- Method 4: Custom Parsing Function. Strengths: Fully customizable and does not depend on external libraries. Weaknesses: Potentially error-prone with complex CSV data.
- Method 5: Bonus One-Liner. Strengths: Extremely succinct. Weaknesses: Not very readable and limited in application for more complicated CSV structures.
March 02, 2024 at 03:41AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
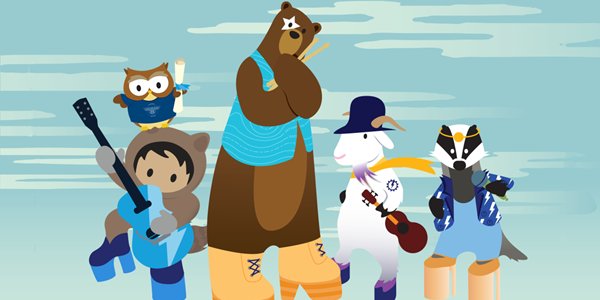
Post a Comment