5 Best Ways to Convert CSV to GPX in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Converting data from a CSV file to GPX format is a common requirement for professionals working with GPS and location data. For instance, you might need to convert a list of latitude and longitude coordinates from a CSV file to a GPX file to use with GPS software or services. This article outlines methods to achieve this conversion using Python.
Method 1: Using pandas and gpxpy Libraries
Combining the pandas
library for CSV data manipulation and the gpxpy
library for creating GPX files, this method offers a robust solution for converting between file formats. It provides a high level of customization and error-handling capabilities.
Here’s an example:
import pandas as pd import gpxpy import gpxpy.gpx # Read CSV file data = pd.read_csv('locations.csv') # Create a new GPX object gpx = gpxpy.gpx.GPX() # Create waypoints for index, row in data.iterrows(): waypoint = gpxpy.gpx.GPXWaypoint(latitude=row['latitude'], longitude=row['longitude']) gpx.waypoints.append(waypoint) # Save to a GPX file with open('output.gpx', 'w') as f: f.write(gpx.to_xml())
Output GPX file: output.gpx
with waypoints from the CSV data.
This code snippet reads a CSV file into a pandas DataFrame, iterates over its rows to create waypoints, adds them to a GPX object, and finally writes the GPX file to disk. It’s concise and leverages the power of existing libraries for data handling and format conversion.
Method 2: Using csv and lxml Libraries
For those who prefer lower-level control over the GPX file construction, the csv
and lxml.etree
libraries provide a means to manually build the GPX structure. This method requires a more in-depth understanding of the GPX XML schema.
Here’s an example:
import csv from lxml import etree as ET # Create the root GPX element gpx = ET.Element('gpx', version="1.1", creator="csv_to_gpx") # Read CSV file and create GPX waypoints with open('locations.csv', 'r') as csvfile: reader = csv.DictReader(csvfile) for row in reader: wpt_element = ET.SubElement(gpx, 'wpt', lat=row['latitude'], lon=row['longitude']) ET.SubElement(wpt_element, 'name').text = row['name'] # Write to a GPX file tree = ET.ElementTree(gpx) tree.write('output.gpx', pretty_print=True, xml_declaration=True, encoding='UTF-8')
Output GPX file: output.gpx
with waypoints and names from the CSV data.
This snippet manually creates a GPX file from a CSV using the csv
module to read input and the lxml
library to build the GPX XML. The result is a customized GPX file written precisely to the user’s specifications.
Method 3: Using Simple Template Substitution
If you don’t need extensive GPX features and your CSV file format is always the same, a simple string template substitution using Python’s string.Template
can be surprisingly efficient.
Here’s an example:
from string import Template # Template for a GPX waypoint wpt_template = Template('<wpt lat="$latitude" lon="$longitude"><name>$name</name></wpt>') # Read CSV file and substitute values into the template gpx_content = '<gpx creator="csv_to_gpx">\n' with open('locations.csv', 'r') as csvfile: next(csvfile) # Skip header line for line in csvfile: latitude, longitude, name = line.strip().split(',') gpx_content += wpt_template.substitute(latitude=latitude, longitude=longitude, name=name) + '\n' gpx_content += '</gpx>' # Write to a GPX file with open('output.gpx', 'w') as f: f.write(gpx_content)
Output GPX content: Plain text representation of a GPX file containing waypoints.
This method skips CSV and GPX parsing libraries entirely and uses pure Python templating to generate a GPX format. It’s useful for simple, one-off tasks with predictable CSV structures but lacks the robustness and flexibility of a full parser.
Method 4: Command-Line Tools via Python
Packages like gpx_csv_converter
provide command-line tools that can be invoked from Python using the subprocess
module. This is helpful when you prefer to use a tried-and-tested standalone utility.
Here’s an example:
import subprocess # Assuming 'gpx_csv_converter' is installed and added to the PATH subprocess.run(['gpx_csv_converter', 'locations.csv', 'output.gpx'])
No output in Python; check the output.gpx
file created in the working directory.
This snippet leverages the external ‘gpx_csv_converter’ tool to perform the conversion outside of the Python environment. It’s an excellent approach when such reliable tools are available and can be easily integrated into Python scripts.
Bonus One-Liner Method 5: pandas and GeoPandas
For those already using the geospatial data in pandas
, the geopandas
extension offers an even simpler one-liner conversion to save a DataFrame directly to a GPX file.
Here’s an example:
import pandas as pd import geopandas # Read CSV file as a GeoDataFrame gdf = geopandas.GeoDataFrame(pd.read_csv('locations.csv')) # Save it directly as a GPX file gdf.to_file('output.gpx', driver='GPX')
Output GPX file: output.gpx
, generated from the GeoDataFrame.
GeoPandas abstracts away the details of the file format conversion, offering a direct method for GeoDataFrame users to export their geospatial data as GPX. This method is simple, clean, and effective but requires that you’re working within the GeoPandas environment.
Summary/Discussion
- Method 1: pandas and gpxpy. Highly customizable and Pythonic. May have a learning curve for newcomers.
- Method 2: csv and lxml. Offers granular control of the GPX XML schema. Requires more code and an understanding of XML.
- Method 3: Simple Template Substitution. Quick for simple structures and small datasets. Not robust or flexible for varying schemas.
- Method 4: Command-Line Tools via Python. Utilizes proven external tools and simplifies integration. External dependencies and less control over the process.
- Method 5: pandas and GeoPandas. The simplest method for those in the GeoPandas ecosystem. Limited to users of GeoPandas.
March 02, 2024 at 03:41AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
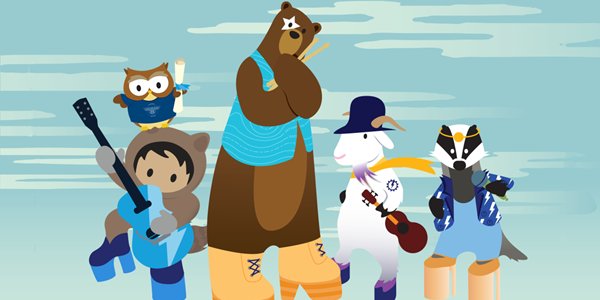
Post a Comment