The Basics of Text File Editing Commands in PowerShell : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with text files in a Windows environment, it’s often necessary to perform tasks like creating, reading, appending, and deleting text content programmatically.
For example, you might want to create a script that logs events to a text file, or modify configuration files for software deployment.
PowerShell, the robust command-line shell and scripting language, presents a variety of commands for handling such tasks effectively. This article addresses PowerShell commands that let you manipulate text files efficiently. We aim to cover these commands using practical examples, providing both input scenarios and the corresponding desired outputs.
Method 1: Creating and Writing to Files with Set-Content
The Set-Content
cmdlet in PowerShell is used to write and replace the content in a specified item, such as a text file. It’s particularly handy when you want to create a new file or overwrite an existing one with new content. Its function is similar to the basic ‘write’ operation in file handling.
Here’s an example:
Set-Content -Path 'C:\example\diary.txt' -Value "Dear Diary, today PowerShell helped me automate a task."
Output:
The file 'diary.txt' will contain the single line: "Dear Diary, today PowerShell helped me automate a task."
In this snippet, Set-Content
is creating a new file called diary.txt
at the specified path and writing a string into that file. If the file already exists, Set-Content
will overwrite the contents without prompting, which makes it crucial to use it with precision.
5 Easy Ways to Edit a Text File from Terminal (macOS)
Method 2: Appending to Files with Add-Content
Where Set-Content
is about writing or overwriting files, the Add-Content
cmdlet allows you to append data to a file, keeping the existing content intact. This is ideal for log files or any scenario where you need to add information to an ongoing record without disturbing the existing entries.
Here’s an example:
Add-Content -Path 'C:\example\tech_journal.txt' -Value "Entry 1: Exploring PowerShell's capabilities."
Output:
The file 'tech_journal.txt' will have "Entry 1: Exploring PowerShell's capabilities." appended to it.
The Add-Content
command in our example appends a new line to the end of the file ‘tech_journal.txt.’ It’s great for logging because it doesn’t risk erasing what’s already there; it simply adds to it.
5 Easy Ways to Edit a Text File From Command Line (Windows)
Method 3: Reading Files with Get-Content
The Get-Content
cmdlet is the go-to command for reading and displaying the contents of a file. It’s similar to the ‘cat’ command found in Unix/Linux systems. Get-Content
is useful for quickly reviewing the contents of a file without needing to open it in a text editor. The cmdlet can also handle large files since it reads the file line by line by default, which can help with memory management.
Here’s an example:
Get-Content -Path 'C:\example\notes.txt'
Output:
All existing lines in 'notes.txt' will be displayed in the PowerShell console.
The provided code reads the content of ‘notes.txt’ and outputs it directly to the console. This is particularly useful for verifying contents or for using file content as input in a script.
Method 4: Deleting Files with Remove-Item
When the time comes to clean up and remove unnecessary files, the Remove-Item
cmdlet is your tool. You can delete any item in your file system, including text files, with this command. Be cautious as this is a permanent action and the deleted file won’t be available in the Recycle Bin.
Here’s an example:
Remove-Item -Path 'C:\example\obsolete.txt'
Output:
The file 'obsolete.txt' will be permanently deleted from the filesystem.
Using Remove-Item
, as shown in the example, will delete 'obsolete.txt'
from the path specified. There is no output displayed unless there is an error. This command helps keep your directories uncluttered by removing files that are no longer necessary.
How to Edit a Text File in Windows PowerShell?
Bonus One-Liner Method 5: Inline Editing with (Get-Content)
Sometimes you need to quickly perform a find-and-replace within a file. PowerShell can accomplish this in a one-liner by piping the Get-Content
cmdlet into Set-Content
through ForEach-Object
.
Here’s an example:
(Get-Content 'C:\example\story.txt') -replace 'sad', 'happy' | Set-Content 'C:\example\story.txt'
Output:
All instances of the word 'sad' in 'story.txt' are replaced with 'happy'.
In this succinct example, the text ‘sad’ is replaced with ‘happy’ in ‘story.txt’. This is an incredibly powerful one-liner for simple text replacement that doesn’t require opening an editor.
Summary/Discussion
- Method 1: Set-Content. Creates or overwrites a file with specified content. Its strength lies in its straightforwardness; however, caution is required as it can unintentionally overwrite important data.
- Method 2: Add-Content. Appends data to an existing file, perfect for logging or incremental file writing. It’s safe for adding content, but it’s not suitable for modifying existing content.
- Method 3: Get-Content. Reads from a file and outputs its content. It’s efficient for viewing file contents in the console and can be used for further processing in scripts. It doesn’t handle in-file editing, though.
- Method 4: Remove-Item. Deletes files from the file system. While excellent for cleanup, it’s a definitive action that must be used judiciously to avoid accidental data loss.
- Bonus Method 5: Inline Editing with
(Get-Content)
Replace. Offers a powerful and quick way to perform find-and-replace within files. It’s great for simple text manipulations but isn’t practical for complex editing tasks or large-scale file transformations.
February 13, 2024 at 05:29PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
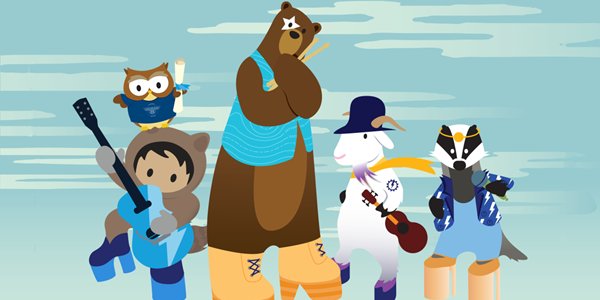
Post a Comment