Python Read Text File Into a List of Lists (5 Easy Ways) : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Read a text file and structure its contents into a list of lists, where each inner list represents a line or a set of values from the file. For instance, given a text file with tabulated data, the goal is to transform each line of data into an individual list so that the entire file becomes a list of these lists.
Method 1: Using a for loop with split()
This method involves reading a file line by line using a for
loop and then splitting each line into a list using the split()
function. This approach is simple and very flexible, as it allows you to specify a delimiter if your data isn’t separated by whitespace.
Here’s an example:
list_of_lists = [] with open('data.txt', 'r') as file: for line in file: list_of_lists.append(line.strip().split('\t')) # Assuming tab-delimited data
The code snippet opens a text file named 'data.txt'
and reads it line by line. Each line is stripped of leading and trailing whitespaces, then split on tabs (\t
), forming an individual list that’s appended to list_of_lists
.
How to Read a Text File Into a List or an Array with Python?
Method 2: List Comprehension with split()
List comprehension is a concise way to create lists in Python. It can be used to read a text file and convert it into a list of lists in a single line of code.
Here’s an example:
with open('data.txt', 'r') as file: list_of_lists = [line.strip().split('\t') for line in file]
This snippet uses list comprehension to iterate over each line in ‘data.txt’, strip it of whitespaces, split each line on tabs, and compile the results into list_of_lists
.
7 Best Ways to Initialize a List of Lists in Python
Method 3: Using the csv.reader module
The csv
module in Python is designed to work with delimited files. The csv.reader
function can be utilized to read a file and automatically split each line into a list, thereby producing a list of lists.
Here’s an example:
import csv with open('data.txt', 'r') as file: list_of_lists = list(csv.reader(file, delimiter='\t'))
In this code, csv.reader
is fed the file object and the delimiter ('\t'
for tab-delimited data). The reader object is converted to a list, assigning it to list_of_lists
.
Method 4: Using numpy.loadtxt
For those dealing with numeric data, numpy
offers a function called loadtxt
which loads data from a text file, with each row in the file becoming a sub-array.
Here’s an example:
import numpy as np list_of_lists = np.loadtxt('data.txt', delimiter='\t').tolist()
This code reads ‘data.txt’ as an array using numpy.loadtxt
, with each line split at tabs. It then converts the array to list_of_lists
using the tolist()
method.
Bonus One-Liner Method 5: Using map() and split()
A combination of map()
and split()
within a list comprehension can offer a quick one-liner solution.
Here’s an example:
with open('data.txt', 'r') as file: list_of_lists = list(map(lambda line: line.strip().split('\t'), file))
This one-liner uses map()
to apply a lambda function that strips and splits each line on tabs, then converts the map object into a list of lists.
Summary/Discussion
- Method 1: For loop with
split()
- Strength: Highly customizable to different file structures and delimiters.
- Weakness: More verbose compared to other methods.
- Method 2: List Comprehension with
split()
- Strength: Offers shorter and cleaner code than a traditional for loop.
- Weakness: Less readable for beginners or complex operations.
- Method 3: Using
csv.reader
- Strength: Efficient and built specifically for delimited files, facilitating error handling.
- Weakness: Overhead of importing an additional module when not already using
csv
.
- Method 4: Using
numpy.loadtxt
- Strength: Ideal for numerical data and integrates well with the
numpy
array operations. - Weakness: Not suitable for non-numeric data and adds dependency on
numpy
.
- Strength: Ideal for numerical data and integrates well with the
- Bonus Method 5: One-liner using
map()
andsplit()
- Strength: Concise one-liner that keeps code compact.
- Weakness: Can be less intuitive and harder to debug compared to list comprehensions or loops.
Python – Convert CSV to List of Lists
February 12, 2024 at 10:50PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
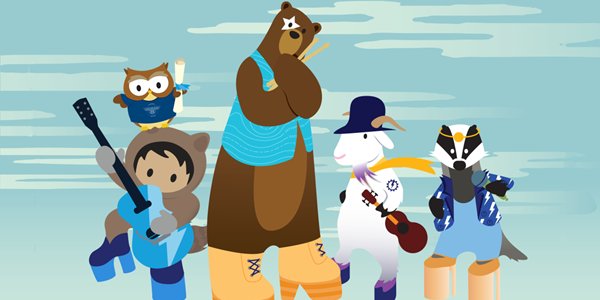
Post a Comment