Python: Count Number of Characters in a String (Length) : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Before diving into the solutions, let’s clearly define the problem. We want to count the number of characters in a string. This includes letters, digits, punctuation, and all other special characters. We assume we are given a string
s
, and we need to find the total number of characters in s
.
Method 1: Using the len() Function
The simplest way to count characters in a python string is to use the built-in len()
function, which returns the length of an object.
s = "Hello, World!" count = len(s) # Result: count = 13

In this code example, len(s)
calculates the number of characters in the string s
and assigns the result to the variable count
.
Method 2: Using a for Loop
Another way to count characters is by iterating over the string using a for
loop and incrementing a counter at each iteration.
s = "Hello, World!" count = 0 for character in s: count += 1
Here, we initialize count
to 0 and then increment count
by 1 for each character in s
.
Method 3: Using the Collections Module
We can also use the Counter
class from the collections
module to count characters, which is particularly useful when you need the count of each character.
from collections import Counter s = "Hello, World!" char_count = Counter(s) total_characters = sum(char_count.values())
Counter(s)
creates a dictionary where keys are the characters and values are their respective counts. sum(char_count.values())
calculates the total characters.
Method 4: Using List Comprehension
List comprehension combined with the len()
function is a less Pythonic way to count characters.
s = "Hello, World!" count = len([character for character in s])
This code creates a list of all characters in s
and then calculates the length of this list. However, this is very clunky and I wouldn’t recommend it. Just use len()
on the string itself!
Method 5: Using map() and sum()
You can use the map()
function with sum()
to achieve the same result in a functional programming style.
s = "Hello, World!" count = sum(map(lambda x: 1, s))
Here, map()
applies a function that returns 1 for each character, and sum()
adds all the returned values together.
Bonus One-Liner Method 6: Using the operator module
For those who love one-liners, Python’s operator
module provides the length_hint()
function, a fast and direct way to get the length of an iterable.
import operator s = "Hello, World!" count = operator.length_hint(s)
length_hint()
returns an estimation of the number of elements in s
, which, for a string, is the exact length.
Summary/Discussion
These methods represent different ways to count characters in a string in Python, ranging from the straightforward len()
function to more complex iterations and functional programming approaches. In 99% of cases, you’d be advised to use the simple, most straightforward Method 1: the len()
function.
February 04, 2024 at 08:29PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
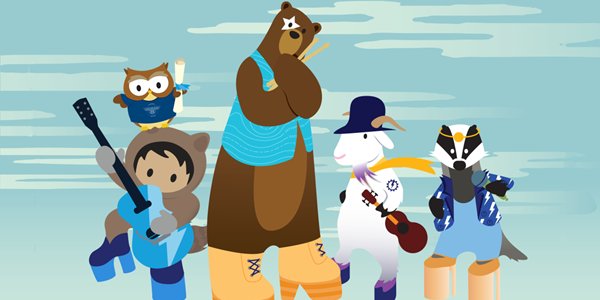
Post a Comment