: Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Python Filter List of Strings Startswith
Problem Formulation: When working with lists of strings in Python, a common task is to filter the list based on whether the strings start with a specific prefix. Suppose you have a list of names, and you want to retrieve only those that start with the letter
"J"
.
Below are several methods to accomplish this.
Method 1: Using a List Comprehension
List comprehensions are a concise way to create lists in Python and can be used for filtering. In this method, we iterate over the list and include only strings that match our criterion.
Here’s an example:
names = ['John', 'Jane', 'George', 'Jim'] j_names = [name for name in names if name.startswith('J')] print(j_names) # Output: ['John', 'Jane', 'Jim']

This code iterates through each element in the names
list, checks if the startswith
method returns True
for the prefix 'J'
, and includes the name in the new list j_names
if it does.
Method 2: Using the filter Function
The filter()
function in Python can be used to filter elements out of an iterable based on a function. In this case, a lambda function can be utilized to check the prefix.
Here’s an example:
names = ['John', 'Jane', 'George', 'Jim'] j_names = list(filter(lambda name: name.startswith('J'), names)) print(j_names) # Output: ['John', 'Jane', 'Jim']
Here, filter
applies a lambda function to each name
in names
and returns only those that start with 'J'
. We wrap the filter
object with list()
to convert it into a list.
Method 3: Using a For Loop
A traditional for loop can also be used to filter the list by appending matching elements to a new list.
Here’s an example:
names = ['John', 'Jane', 'George', 'Jim'] j_names = [] for name in names: if name.startswith('J'): j_names.append(name) print(j_names) # Output: ['John', 'Jane', 'Jim']
In this snippet, we create an empty list j_names
and loop over names
. If a name
starts with ‘J’, it gets appended to j_names
.
Method 4: Using the fnmatch Module
The fnmatch
module provides support for Unix shell-style wildcards, which can be used for string matching. This can be handy for more complex patterns.
Here’s an example:
import fnmatch names = ['John', 'Jane', 'George', 'Jim'] j_names = [name for name in names if fnmatch.fnmatch(name, 'J*')] print(j_names) # Output: ['John', 'Jane', 'Jim']
fnmatch.fnmatch(name, 'J*')
checks if the name
matches the pattern ‘J‘, where ‘‘ stands for any character(s). Matching names are added to j_names
.
Method 5: Using Regular Expressions
For pattern matching more complex than the startswith
case, regular expressions are powerful. This method uses the re
module to match strings that start with 'J'
.
Here’s an example:
import re names = ['John', 'Jane', 'George', 'Jim'] j_names = [name for name in names if re.match(r'^J', name)] print(j_names) # Output: ['John', 'Jane', 'Jim']
The regular expression r'^J'
checks for strings that start with 'J'
. We include only those matches in j_names
.
Bonus One-Liner Method 6: Using next
and Generator Expressions
When you only need the first match, a generator expression combined with next
can be efficient.
names = ['John', 'Jane', 'George', 'Jim'] j_name = next((name for name in names if name.startswith('J')), None) print(j_name) # Output: 'John'
This code creates a generator expression that yields names starting with 'J'
, and next
retrieves the first one. The second argument to next
, None
, is a default value if no match is found.
Summary/Discussion
Filtering lists in Python based on string prefixes can be done using various methods.
List comprehensions and the filter
function are both concise and expressive, while traditional loops offer clarity to beginners.
Modules like fnmatch
and re
provide pattern matching capabilities beyond simple prefixes.
The one-liner with next
is a neat trick for fetching the first matching element only, which might come in handy in scenarios where you are looking for a single, specific item.
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills. You’ll learn about advanced Python features such as list comprehension, slicing, lambda functions, regular expressions, map and reduce functions, and slice assignments.
You’ll also learn how to:
- Leverage data structures to solve real-world problems, like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array, shape, axis, type, broadcasting, advanced indexing, slicing, sorting, searching, aggregating, and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups, negative lookaheads, escaped characters, whitespaces, character sets (and negative characters sets), and greedy/nongreedy operators
- Understand a wide range of computer science topics, including anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, searching, and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined, and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
February 05, 2024 at 05:17PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
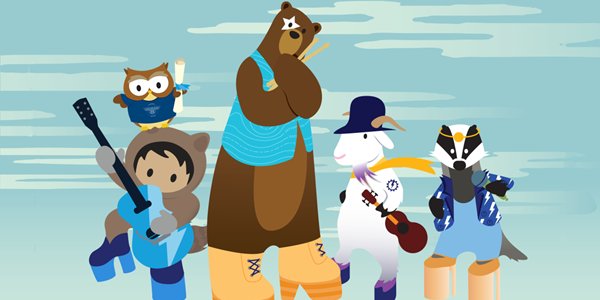
Post a Comment