5 Ways to Edit Remote Text Files with PowerShell : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: You’re managing multiple servers or systems, possibly in a mixed environment (e.g., some hosts on-premises, others in the cloud), and need to update configuration or text files remotely. The challenge is to do this efficiently and reliably using PowerShell, with a prime example being the need to change a specific line in a configuration file on multiple remote Windows servers. The desired output would adjust the target line’s content across all servers without manually logging into each machine.
Method 1: Using Invoke-Command with Set-Content
Invoke-Command
is a PowerShell cmdlet that allows scripts to be run on remote sessions or on a local machine. When paired with Set-Content
, it provides a straightforward method to edit text files remotely. This method requires that the remote server has PowerShell Remoting enabled and that the user has the necessary permissions.
Here’s an example:
Invoke-Command -ComputerName Server01, Server02 -ScriptBlock { Set-Content -Path C:\configs\example.txt -Value "New Content" -Force }
Output:
// The content of example.txt on Server01 and Server02 has been replaced with "New Content"
This code snippet remotely changes the entire content of the file example.txt
to "New Content"
on both Server01
and Server02
. The -Force
parameter is used to ensure that the changes are made even if the file is in use.
How to Edit a Text File in Windows PowerShell?
Method 2: Using New-PSDrive
New-PSDrive
lets you create a temporary PowerShell Drive that is connected to a remote file system. You can use this to edit files as if they were on your local system. The New-PSDrive
cmdlet is particularly useful when you have ongoing editing tasks on a remote machine.
Here’s an example:
New-PSDrive -Name RemoteServer -PSProvider FileSystem -Root "\\Server01\c$" -Credential (Get-Credential) Set-Content -Path RemoteServer:\configs\example.txt -Value "Updated Content" Remove-PSDrive -Name RemoteServer
Output:
// A prompt for credentials appears, and after providing them, the content of example.txt on Server01 will be changed to "Updated Content"
The script creates a drive named RemoteServer that maps to the C$ administrative share on Server01. Once the credentials are provided, Set-Content is used to edit example.txt. Afterward, the temporary drive is removed with Remove-PSDrive.
Method 3: Using Copy-Item and Invoke-Command
This method combines the use of local editing and remote updating. You first edit the file locally, then use Copy-Item
within an Invoke-Command
script block to replace the remote file with the updated local version.
Here’s an example:
# Edit the file locally Set-Content -Path .\localExample.txt -Value "Locally Updated Content" # Copy the updated file to the remote server Invoke-Command -ComputerName Server01 -ScriptBlock { Copy-Item -Path C:\temp\localExample.txt -Destination C:\configs\example.txt -Force } -ArgumentList (Get-Item .\localExample.txt)
Output:
// The example.txt file contents on Server01 are replaced by the contents of localExample.txt from your local machine
The localExample.txt
file is edited locally with Set-Content
. The updated file is then copied over to the remote Server01
by using Copy-Item
inside an Invoke-Command
block.
5 Easy Ways to Edit a Text File From Command Line (Windows)
Method 4: Using Enter-PSSession and Set-Content
Enter-PSSession is used for interactive sessions with a remote system. However, it could be scripted to automate text editing tasks. This should be used carefully, as it’s intended for manual operations.
Here’s an example:
$session = New-PSSession -ComputerName Server01 -Credential (Get-Credential) Enter-PSSession -Session $session Set-Content -Path C:\configs\example.txt -Value "Interactively Changed Content" Exit-PSSession Remove-PSSession -Session $session
Output:
// After credentials are accepted, the content of example.txt on Server01 will now say "Interactively Changed Content"
In this code, New-PSSession creates a remote session to Server01 which is then entered interactively using Enter-PSSession. Set-Content is used to change the file’s content. The remote session is exited and then removed.
Bonus One-Liner Method 5: Using SCP with PowerShell Core
If you’re using PowerShell Core (available on macOS, Linux, and Windows), you can use the Secure Copy Protocol (SCP) to edit text files remotely in a single line of PowerShell.
Here’s an example:
scp ./localExample.txt user@server01:/configs/example.txt
Output:
// The file localExample.txt from the local machine is copied to example.txt on server01, replacing its content
With this approach, SCP is used to securely transfer the locally edited file to overwrite the remote file. This is a quick and secure method for systems with SCP enabled.
Summary/Discussion
- Method 1: Invoke-Command with Set-Content. Strengths: Direct and simple for remote execution of scripts. Weaknesses: Fully replaces file content; requires PowerShell Remoting.
- Method 2: New-PSDrive. Strengths: Allows for seamless mapping of remote drives; interactive editing is possible. Weaknesses: May have permission complexities with remote drive access.
- Method 3: Copy-Item and Invoke-Command. Strengths: Ideal for bulk updates with a local editing preference. Weaknesses: Requires two steps: local editing and remote copying.
- Method 4: Enter-PSSession and Set-Content. Strengths: Great for interactive management and editing. Weaknesses: Not ideal for scripting; intended for manual session management.
- Method 5: SCP with PowerShell Core. Strengths: Quick and secure; doesn’t require PowerShell Remoting. Weaknesses: Only available in PowerShell Core; requires SCP setup on both ends.
February 13, 2024 at 05:24PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
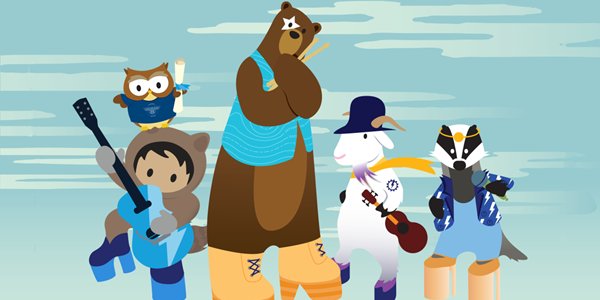
Post a Comment