5 Best Ways to Remove the Index Column in Pandas DataFrame : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When dealing with data in pandas DataFrames, a common requirement is to remove the index column when exporting the data to a file. The default index can be repetitive or unnecessary, especially if the data already contains a unique identifier. Users seek techniques to remove or ignore the index to prevent it from becoming an unwanted column in their output file. For instance, given a DataFrame with the default index, a user may wish to save it to a CSV without the index column being present.
Method 1: Use to_csv
without the Index
The to_csv
method in the pandas library can save a DataFrame to a CSV file. It has the index
parameter, which you can set to False
to suppress writing the index column to the CSV file. This method is straightforward and often used when the only target is to save to a CSV without the index.
Here’s an example:
import pandas as pd # Creating a simple DataFrame df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) # Saving to CSV without the index df.to_csv('output.csv', index=False)
The output will be a CSV file containing:
A,B 1,3 2,4
This code snippet shows how to create a simple DataFrame and then save it to a CSV file called “output.csv” using the to_csv
method with index=False
to exclude the index from the output.
Method 2: Disabling the Index Upon DataFrame Creation
You can create a DataFrame without an index by setting the index
parameter to None
in the DataFrame constructor. This way, the DataFrame is generated without an explicit index, and there will be nothing to remove before exporting or using the data.
Here’s an example:
import pandas as pd # Creating a DataFrame without an index df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}, index=[None]*2) # Displaying the DataFrame print(df)
The output will display:
A B None 1 3 None 2 4
In this example, by setting the index
parameter to a list of None
values that matches the number of rows, we create a DataFrame without a standard numeric index. This DataFrame can then be used directly without the need for index manipulation.
Method 3: Resetting the Index
Resetting the index of a DataFrame involves creating a new default integer index and transforming the old index into a column. If you further set the drop
parameter to True
, the original index gets removed.
Here’s an example:
import pandas as pd # Suppose we have a DataFrame with a custom index df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}, index=['x', 'y']) # Resetting the index and dropping the old one df_reset = df.reset_index(drop=True) print(df_reset)
Output:
A B 0 1 3 1 2 4
The code snippet resets the index of the DataFrame by dropping the current index and replacing it with the default integer index. No additional index column is added to the DataFrame.
Method 4: Dropping the Index Column Directly
If your index has a name and has been converted into a column already (for example, by a previous reset of the index without dropping), you can drop it using the drop
method by specifying the index’s name.
Here’s an example:
import pandas as pd # DataFrame with the index turned into a column named 'Index' df = pd.DataFrame({'Index': ['x', 'y'], 'A': [1, 2], 'B': [3, 4]}).set_index('Index') # Dropping the 'Index' column df_dropped = df.reset_index().drop('Index', axis=1) print(df_dropped)
The output will show:
A B 0 1 3 1 2 4
This code snippet demonstrates the removal of a named index that was previously turned into a column in the DataFrame. Using reset_index()
brings the index into the frame as a column, and drop()
with the axis set to 1 (columns) removes it altogether.
Bonus One-Liner Method 5: Use to_string
or to_html
without the Index
In situations where the output format is a string or HTML, such as when displaying a DataFrame in a web application, pandas provides to_string()
and to_html()
methods which have the index
parameter to exclude the index.
Here’s an example:
import pandas as pd # A simple DataFrame df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) # Convert the DataFrame to HTML without the index html_output = df.to_html(index=False) print(html_output)
This command outputs the DataFrame as an HTML table without including the index:
<table border="1" class="dataframe"> <thead> <tr style="text-align: right;"> <th>A</th> <th>B</th> </tr> </thead> <tbody> <tr> <td>1</td> <td>3</td> </tr> <tr> <td>2</td> <td>4</td> </tr> </tbody> </table>
The code snippet converts the DataFrame to an HTML table, omitting the index by using the to_html
method with index=False
.
Summary/Discussion
- Method 1:
to_csv
without Index. Straightforward for CSV export. Limited to one file format. - Method 2: Disabling the Index Upon DataFrame Creation. Prevents initial index. May require external control of input data structure.
- Method 3: Resetting the Index. Versatile in resetting to default. The original index gets lost unless saved beforehand.
- Method 4: Dropping the Index Column Directly. Direct when index already in column form. Requires the index to be named.
- Bonus Method 5:
to_string
orto_html
without Index. Useful for representations. Not suitable for data storage practices.
February 19, 2024 at 02:53AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
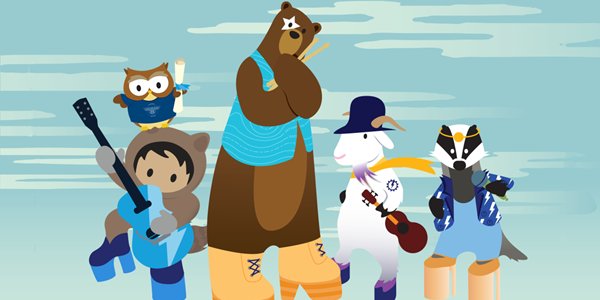
Post a Comment