5 Best Ways to Create Numpy Arrays of Tuples in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post

When working with large datasets in Python, it’s common to need to create Numpy arrays that hold tuples as their elements. This problem revolves around transforming a collection of tuples, representing multidimensional data points, into a structured Numpy array where each tuple becomes an array element. Suppose the input is a list of tuples like [(1, 2), (3, 4)]
, the desired output would be a Numpy array, potentially for further vectorized operations.
Method 1: Using numpy.array
This method involves the direct use of the numpy.array()
function to transform a list of tuples into a Numpy array. The function delineates the structure of the resultant array, allowing for multidimensional array creation that can be tailored through data types and order parameters.
Here’s an example:
import numpy as np tuples_list = [(1, 2), (3, 4), (5, 6)] np_array_of_tuples = np.array(tuples_list) print(np_array_of_tuples)
Output:
[[1 2] [3 4] [5 6]]
This code snippet first defines a list of tuples. It then uses the numpy.array()
function to convert this list into a Numpy array, preserving the tuple structure as the elements of the array.
Method 2: Using numpy.asarray
The numpy.asarray()
function is an alternative that converts an input sequence into an array. It’s similar to numpy.array()
, but does not copy the object if it is already an ndarray with matching dtype and order. It is efficient when you’re dealing with sequences already in a numpy-like structure.
Here’s an example:
import numpy as np tuples_list = [(7, 8), (9, 10), (11, 12)] np_array_of_tuples = np.asarray(tuples_list) print(np_array_of_tuples)
Output:
[[ 7 8] [ 9 10] [11 12]]
This example starts with a list of tuples and uses numpy.asarray()
to create a numpy array. If the provided list of tuples were already an array, asarray()
would not needlessly make a copy of the data.
Method 3: Using numpy.fromiter
The numpy.fromiter()
function creates a new one-dimensional array from an iterable object, such as a generator expression or iterator. To create an array of tuples, the iterable should yield tuples. This method can be more memory-efficient than creating a list first.
Here’s an example:
import numpy as np tuples_iterator = iter([(13, 14), (15, 16), (17, 18)]) np_array_of_tuples = np.fromiter(tuples_iterator, dtype='i,i') print(np_array_of_tuples)
Output:
[(13, 14) (15, 16) (17, 18)]
Here, we create an iterator from a list of tuples and pass it to numpy.fromiter()
, specifying the data type as a pair of integers (i,i
). The output is a one-dimensional array of tuple elements, making it ideal for sequence conversions.
Method 4: Using numpy.zeros with a structured dtype
NumPy’s numpy.zeros()
can create an array filled with zeros. By specifying a structured data type (dtype), you can form an array of zeros where each zero element is replaced with a tuple structure.
Here’s an example:
import numpy as np tuple_shape = (3,) # the desired shape of the array dtype = [('x', 'int32'), ('y', 'int32')] # specifying tuple structure as a list of pairs np_array_of_tuples = np.zeros(tuple_shape, dtype=dtype) print(np_array_of_tuples)
Output:
[(0, 0) (0, 0) (0, 0)]
In this code snippet, we use numpy.zeros()
with a specified structured dtype to create a structured array. While the initial array is filled with zeros, it can be subsequently populated with the actual tuple data.
Bonus One-Liner Method 5: List Comprehension with numpy.array
A one-liner method involves using a list comprehension inside the numpy.array()
function call. This method is great for cases where the tuples need to be generated or transformed before creating the array.
Here’s an example:
import numpy as np np_array_of_tuples = np.array([(x, x+1) for x in range(3)]) print(np_array_of_tuples)
Output:
[[0 1] [1 2] [2 3]]
This one-liner uses list comprehension to create tuples on the fly and immediately converts them into a Numpy array using numpy.array()
.
Summary/Discussion
- Method 1: numpy.array. Straightforward. Ideal for lists. Can be memory intensive.
- Method 2: numpy.asarray. Efficient for existing sequences. Less intuitive for tuple creation.
- Method 3: numpy.fromiter. Memory-efficient for large sequences. Limited to one-dimensional arrays.
- Method 4: numpy.zeros with structured dtype. Allows preset array structure. Initial values will be zeros.
- Method 5: List Comprehension with numpy.array. Compact and convenient for on-the-fly transformations. Less readable with complex transformations.
February 20, 2024 at 06:50PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
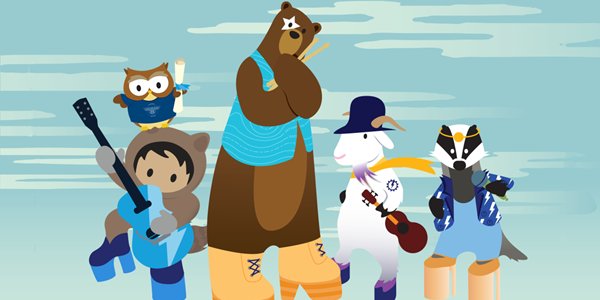
Post a Comment