What is the Fastest Way to Get a Sorted Unique List in Python? : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Understanding the Problem
When working with Python, you might often find yourself with a list of items where you need to remove duplicates and then sort the list. Let’s say you have a list of numbers, but this could be any ‘hashable‘ items – meaning items that can be compared for equality and can be used as keys in Python dictionaries, like numbers, strings, and tuples.
Imagine you have a list like this:
my_list = [5, 4, 2, 8, 4, 2, 1]
In this list, the numbers 4 and 2 appear more than once. Your goal is to:
- Remove these duplicates.
- Sort the remaining numbers in ascending order.
The result should be a list (or any iterable) that looks like this:
[1, 2, 4, 5, 8]
A typical way to do this in Python is to use set
to remove duplicates and then sorted
to sort the list:
sorted_unique_list = sorted(set(my_list))
While the above method works, you might wonder if there’s a more efficient way to do this, especially if you’re working with large lists where performance matters.
The concern is that by first converting to a set and then sorting, we might be doing unnecessary work.
Is there a way to sort and remove duplicates in one step, similar to how the Unix command sort | uniq
works, where sorting inherently helps in identifying duplicates?
You’re looking for the fastest and most memory-efficient way to achieve this in Python. This is particularly important for your use case, where the list is only used once (a ‘throwaway’ list), making an in-place solution (modifying the original list) potentially more desirable for saving memory.
It’s Still The Fastest Solution!
The best solution to create a sorted, unique list in Python, particularly focusing on efficiency, is to use a combination of Python’s built-in functions and data structures in a smart way. Here’s an approach that is both efficient and concise:
input_list = [5, 4, 2, 8, 4, 2, 1] sorted_unique_list = sorted(set(input_list))
Using set(input_list)
: This removes duplicates from the list. A set in Python is an unordered collection of unique elements. By converting the list to a set, we automatically discard any duplicates.
Using sorted()
: The sorted function then takes this set of unique elements and returns a new list that is sorted in ascending order.
Why Is This Efficient?
Time Complexity: The most time-consuming operations here are the creation of the set and the sorting. The average time complexity for creating a set is O(n), where n is the number of elements in the list. Sorting has a time complexity of O(n log n). Therefore, the overall complexity remains quite efficient.
Space Complexity: Since the list may be a throwaway (used only once), using this method is fine in terms of memory usage. The set operation creates a new set, and the sorted operation creates a new list. However, since the original list is not needed anymore, this shouldn’t be a concern in that case.
If memory efficiency is a higher priority and modifying the original list is acceptable, you could potentially sort the list first and then remove duplicates in place.
However, this method is more complex and does not necessarily offer significant performance improvements over the sorted(set(input_list))
approach.
For most practical purposes, especially with Python’s efficient handling of data structures and its built-in functions, sorted(set(input_list))
is an excellent balance between efficiency, readability, and conciseness. It is suitable for a wide range of applications, including scenarios involving large datasets.
Alternative Solutions That Are Inferior
- Using a loop to manually check and add unique items: This method is generally slower and more verbose compared to using
set
, as it involves manually iterating through each element and checking for uniqueness. - Sorting first and then removing duplicates in-place: While this can be memory efficient, it is computationally less efficient and more complex in code compared to
sorted(set(input_list))
. - Using a dictionary to track occurrences: This approach is overkill for the task, adding unnecessary complexity and memory usage compared to the simplicity of a set.
- Heapq module for sorting and deduplication: While useful for specific cases, using
heapq
is generally slower for simple sorting and deduplication tasks and adds complexity. - Using
numpy.unique()
for arrays: This is efficient for NumPy arrays but adds a dependency and is less efficient for native Python lists compared tosorted(set(input_list))
. - Using pandas’ unique and sort functionalities: While powerful for large datasets, using pandas for this task is excessive for simple lists and requires an external library.
- List comprehension with conditional checks: This can be less efficient than using
set
due to the need to manually check for duplicates and is less readable.
Advanced Tutorial: Sorting One List Based on Another

Sorting a List Based on Values From Another List
January 08, 2024 at 02:58AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
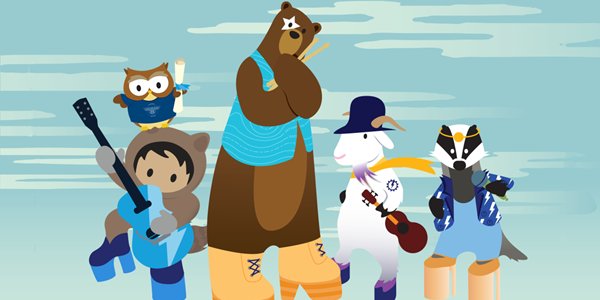
Post a Comment