Python Print String and Variable: A Guide to Output Formatting in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
When working with Python, one of the most fundamental tasks you’ll encounter is outputting data to the screen. This is where the print()
function becomes a tool you’ll use frequently. The simplicity of Python’s print function belies its importance; it’s the basic means of displaying information, a critical part of debugging, and a way to give users feedback or insights into the process of a program. Whether you’re a beginner looking to understand the basics or an experienced programmer who needs to ensure output is formatted correctly, mastering the print()
function and its capabilities to handle strings and variables is essential.

To print a string and a variable, you have various methods at your disposal, each suited to different scenarios. Concatenation and string formatting are two techniques that will allow you to combine strings and variables effectively. Use concatenation when you need to join strings directly with variables, ensuring any non-string variable is converted to a string first. For more complex outputs, string formatting using the format()
method or formatted string literals, better known as f-strings, provide powerful ways to embed variables within strings, allowing for more control and customization.
Understanding Python’s Print Function

The print()
function in Python is your primary tool for displaying output to the console. This article guides you through the essentials of using print()
, various string formatting techniques, and how to manage output of different data types efficiently.
Basics of the Print() Function
To produce output in Python, use the print()
function, which is both versatile and easy to use. You can pass multiple strings or variables, separated by commas, and print()
will convert them to a string and concatenate them with spaces by default. For example:
name = "Alice" print("Hello", name) # Output: Hello Alice
Your output appears in the console, providing a straightforward way to debug or present information.
Exploring String Formatting Methods
There are several methods for string formatting in Python that allow for cleaner and more dynamic output:
- Concatenation: Combine variables and strings using
+
:age = 30 print("You are " + str(age) + " years old.")
- Format Strings: Use the
.format()
method or f-strings for inserting values into string placeholders:- With
.format()
:temperature = 75.5 print("Current temperature is {} degrees.".format(temperature))
- With f-strings (Python 3.6+):
temperature = 75.5 print(f"Current temperature is {temperature} degrees.")
- With
Working with Variables and Data Types
The print()
function can handle any type of variable you throw at it by automatically converting it to a string:
- Integers and floats: Directly pass numeric values to
print()
. - Lists and dictionaries: Enclosed in brackets, their elements are printed as comma-separated items.
- Objects: Python calls the object’s
__str__()
method to obtain a printable string representation.
Here’s how you print different data types:
number = 42 pi = 3.14159 inventory = ['sword', 'armor', 'potion'] hero_stats = {"strength": 18, "dexterity": 12, "charisma": 15} print(number) # Output: 42 print("Value of pi:", pi) # Output: Value of pi: 3.14159 print("Inventory items:", inventory) # Output: Inventory items: ['sword', 'armor', 'potion'] print("Hero stats:", hero_stats) # Output: Hero stats: {'strength': 18, 'dexterity': 12, 'charisma': 15}
Mastering the print()
function equips you with a fundamental skill for generating readable and useful output in Python.
Advanced Printing Techniques

When working with Python’s print function, it’s important to understand how to efficiently combine strings and variables for output. This section will guide you through some of the advanced techniques that can elevate the clarity and efficiency of your code.
Utilizing F-Strings and Format Specifiers
Python 3.6 introduced f-strings, a feature that allows you to embed expressions inside string literals using curly braces {}
and a leading f
. For instance:
name = "Alice" print(f"Hello, {name}!")
F-strings are not only concise but also faster at runtime. With the use of format specifiers, they offer powerful formatting options. You can control the precision of a float or format an integer as binary with expressions like {value:.2f}
and {value:b}
respectively.
Concatenating Strings and Variables
The process of combining strings and variables, known as string concatenation, can be done using the +
assignment operator. However, this method requires all objects to be strings; non-string variables must be converted using the str()
function:
age = 30 print("You are " + str(age) + " years old.")
Although concatenation is straightforward, using ,
as a separator in the print()
function or .format() method can be more flexible, as they handle different data types without needing explicit type conversion.
Handling Errors and Best Practices
A common mistake that can result in a TypeError
is trying to concatenate non-string types without conversion. To avoid this, always ensure variables are the correct type before concatenating. For better readability and maintenance, consider using logging instead of print()
for complex programs. Always be aware of the version-specific features of Python; for example, the % operator and .format() are available in Python 2.7, whereas f-strings are only in Python 3.6 and later. Using these features appropriately will make your code cleaner and more efficient.
Remember to handle expressions and variables with care in your strings, and opt for the method that best fits the complexity of your data and the goal of your code for maximum efficiency and clarity.
Frequently Asked Questions

When working with text and variables in Python, knowing how to correctly combine and print them can greatly streamline your coding process. This section addresses common questions and provides clear answers for efficiently handling string and variable printing.
How do you concatenate a string and a variable in Python for printing?
You concatenate a string and a variable in Python by using the plus sign (+). Ensure that the variable is a string, otherwise, convert it using the str()
function before concatenation.
What syntax is used for printing multiple variables alongside strings in Python?
For printing multiple variables alongside strings, you can separate them with commas inside the print()
function, or use string formatting methods like format()
or f-strings to combine them seamlessly.
How can you format a string to include variables in Python before printing?
You can format a string to include variables by using the str.format()
method or f-strings, which allow for embedded Python expressions inside string literals, prefixed by an f
or F
.
Can you display both the name and the value of a variable in a Python print statement?
Yes, you can display both the variable name and its value by using the print()
statement with f-strings or str.format()
and including the actual variable name in the string text.
What method allows the inclusion of integer variables within a string for printing in Python?
The inclusion of integer variables within a string for printing can be achieved using f-strings or by passing them as arguments to the print()
function, which automatically converts them to strings.
Is there a way to print all variables’ values at once in Python?
To print all variables’ values at once, you may use the locals()
or globals()
function inside a print()
statement to display a dictionary of the current local and global symbol table.
January 17, 2024 at 12:56AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
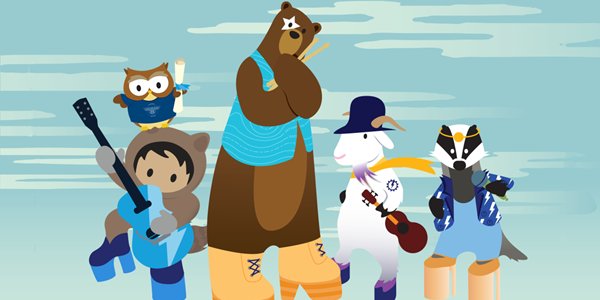
Post a Comment