Python Print String and Int: A Guide to Outputting Data in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
In Python, displaying text and numbers to the console is a fundamental operation, and the print
function is the tool you use to perform it. When you want to show both strings and integers in the output, you might encounter issues if you don’t handle data types correctly. Strings are sequences of characters wrapped in quotes, while integers are whole numbers without any decimal point. Merging these different types into a cohesive output requires knowledge of data type manipulation and string formatting.

Python offers you several methods to concatenate or format strings and integers, enabling you to create a structured and informative output. Understanding these techniques is crucial, whether you’re displaying a simple message or generating complex logs. You can convert an integer to a string to concatenate it, or you can use placeholders in a string to insert numbers and text. The newer f-string feature makes it even more straightforward, allowing you to insert variables directly into a string using curly braces.
Python Print Function Basics

The print()
function is a fundamental tool in Python for displaying output to the console. It allows you to send data of various types, including strings and integers, to the standard output stream.
Understanding Print() Function
The print()
function in Python is your go-to for writing information to the console. When you pass variables or literals as arguments to print()
, the function converts them to a string representation and displays them on your screen.
Data Types and Variables
Python supports different data types such as string
and int
(integer). Variables in Python can store these data types and are placeholders for the data that can be printed out using the print()
function.
Formatting Output in Python
To present data in a readable and aesthetically pleasing format, Python offers several methods for string formatting. Using the format()
method, f-strings, and placeholders enclosed in curly braces {}
, you can construct structured and formatted strings that include variable values.
Concatenating Strings and Integers
Concatenation in Python is the process of combining strings. To concatenate a string and an integer, you must first convert the integer to a string using str()
. Alternatively, you can use commas to separate strings and integers within the print()
function, which converts and outputs them as a single string.
Common Errors and Best Practices

In Python, effectively managing data types and ensuring code clarity are critical for success. The following tips will help you avoid common pitfalls and apply best practices when printing strings and integers.
Avoiding and Resolving TypeErrors
When working with strings and integers in Python, a frequent issue you may come across is a TypeError
. This error occurs when you try to perform an operation on a value that is not compatible with its type. Here are specific examples and how to resolve them:
- Attempting to concatenate a string and an integer directly:
- Error:
TypeError
- Fix: Use
str()
to convert an integer to a string before concatenation:age = 25 print("You are " + str(age) + " years old.")
- Error:
- Using
+
to combine strings and numbers within print statements:- Fix: Leverage formatted string literals (f-strings) available from Python 3.6 onwards for a more readable approach:
age = 25 print(f"You are {age} years old.")
- Fix: Leverage formatted string literals (f-strings) available from Python 3.6 onwards for a more readable approach:
Improving Code Readability
Code readability is about making your code as easy to understand as possible for others and for your future self. To improve the readability of print statements that involve strings and integers, consider the following:
- Use f-strings: These allow you to embed expressions inside string literals using curly braces
{}
:name = "Jamie" tasks_completed = 5 print(f"{name} has completed {tasks_completed} tasks today.")
- Apply the
format()
method: A conventional method applicable in versions prior to Python 3.6, and still useful for complex formatting:name = "Jamie" tasks_completed = 5 print("{} has completed {} tasks today.".format(name, tasks_completed))
By paying attention to error handling and readability, you improve both the functionality and maintainability of your Python programs.
Frequently Asked Questions

In this section, you’ll find concise answers to common questions you may have when working with strings and integers in Python. These are practical insights to help you effectively print and format your data.
How do you convert an integer to a string before printing in Python?
To convert an integer to a string in Python, you can use the str()
function. For example, if you have an integer age = 25
, you can convert it by calling str(age)
.
What is the syntax to concatenate a string and integer in Python?
To concatenate a string and an integer, first convert the integer to a string using str()
, and then you can concatenate it with another string using the +
operator. For instance: greeting = "Hello, you are " + str(age) + " years old."
What methods can be used to print a combination of strings and variables in Python?
You can print a combination of strings and variables by using the print()
function with comma separation, or by using string formatting methods such as the format()
method, f-strings, or %
-formatting.
How can you print multiple data types on the same line in Python?
You can print multiple data types on the same line by separating them with commas within the print()
function, which will convert them to strings and separate them with spaces, or by converting all values to strings and concatenating them.
What are the common mistakes to avoid when printing strings and integers together in Python?
Avoid directly concatenating strings with non-string data types without conversion, and ensure that you do not mistakenly use +
when you intend to output multiple items separated by a space inside print()
.
In Python, how do you format a string to include integers within the output?
You can format a string to include integers by using placeholders in the format()
method, inserting values with f-string syntax f"value: {value}"
, or using the %
operator like "value: %d" % value
. Each of these methods inserts the integer into a string.
January 17, 2024 at 12:56AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
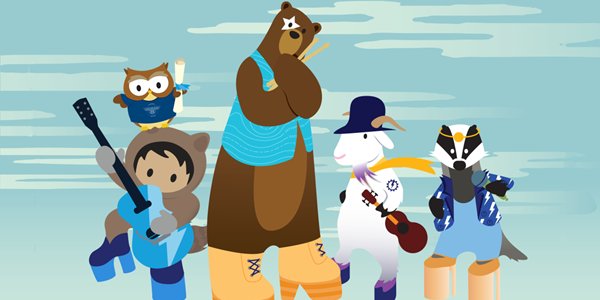
Post a Comment