The No-Code Guide to Advanced Data Structures in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Understanding Python’s Built-In Data Structures

Before diving into specifics, you must understand that Python provides a variety of built-in data structures that offer flexibility and powerful ways to handle data. They include lists, tuples, dictionaries, and sets, each with its own special attributes for different use cases.
Lists as Mutable Sequences
A List in Python is a mutable sequence, allowing you to store a collection of items, be they integers, strings, or other lists. You can modify lists, meaning items can be changed, added, or removed.
For instance, appending to a list is as simple as your_list.append(item)
.
Tuples as Immutable Sequences
On the other hand, a Tuple is Python’s approach to an immutable sequence. Once you create a tuple, such as your_tuple = (1, 'a', 3.14)
, you cannot change its elements. This implies that tuples are a preferred choice when you require data integrity or want to use the structure as a dictionary key.
Dictionaries for Mapping Operations
Dictionaries are incredibly flexible in Python, allowing you to store data in a key-value pairing. Keys are unique within a dictionary and are used for accessing values. To add an item, you simply assign a value to a key like your_dict['new_key'] = 'new_value'
. Dictionary operations are fast and efficient for mapping data.
Sets for Handling Unique Elements
Lastly, Sets offer a way to store unique elements, useful for operations like unions or intersections. A set in Python can be created simply with your_set = {1, 2, 3}
and supports methods like your_set.add(4)
to add more elements, ensuring no duplicates are present.
Efficiency in Data Handling and Access Patterns
Before diving into specific types of data structures, it’s vital you understand how each is integral for speeding up data handling and access. Whether it’s managing data sequences or optimizing lookups, the performance of your Python program can hinge on these structures.
Performance of Array Types
In Python, arrays are excellent when you need to work with elements sequentially because they allow fast access patterns. If you’re handling numerical data, using arrays can lead to significant performance gains, specifically with search and insertion operations at the end of the sequence.
However, insertion or removal at random locations can be costly as it requires shifting elements.
- Search: The time to search for an element is mostly dependent on the size of the array.
- Insertion/Removal: Appending or popping elements at the end of an array is fast, whereas, for other positions, it can be slower due to the need for element shifting.
Optimizing Data Access with Hash Tables
Hash tables—often realized through Python’s native dictionaries—enable you to achieve very fast lookup times. By optimizing data access using hash tables, you ensure that insertions, searches, and removal actions are much more efficient, often close to O(1) time complexity in the best-case scenario.
- Fast Lookup: Accessing an item through a key in a hash table is swift because it doesn’t depend on the size of the hash table.
- Access Efficiency: Efficient access is guaranteed as long as the hash function distributes the entries uniformly across the underlying array.
Understanding Queues and Stacks
With queues, you’re working with a FIFO (First In, First Out) structure which is perfect for scenarios where the order of tasks matters, such as task scheduling. Stacks, on the other hand, adhere to a LIFO (Last In, First Out) principle, ideal for undo mechanisms or parsing expressions.
- Queue:
- Insertion: Adding to the rear (enqueue) is typically done in constant time.
- Removal: Removing from the front (dequeue) is also a quick operation.
- Stack:
- Insertion: Pushing an item onto the stack is efficient and simple to manage.
- Removal: Popping off the top of the stack is equally prompt, making it conducive for certain types of data access patterns.
In both queues and stacks, optimization comes from knowing when to use each structure as they excel in different access patterns. Whether you need fast lookups, sequential access, or efficient insertions and deletions, incorporating the right data structure is key to enhancing efficiency in your Python applications.
Advanced Data Structures: Trees, Heaps, and Graphs
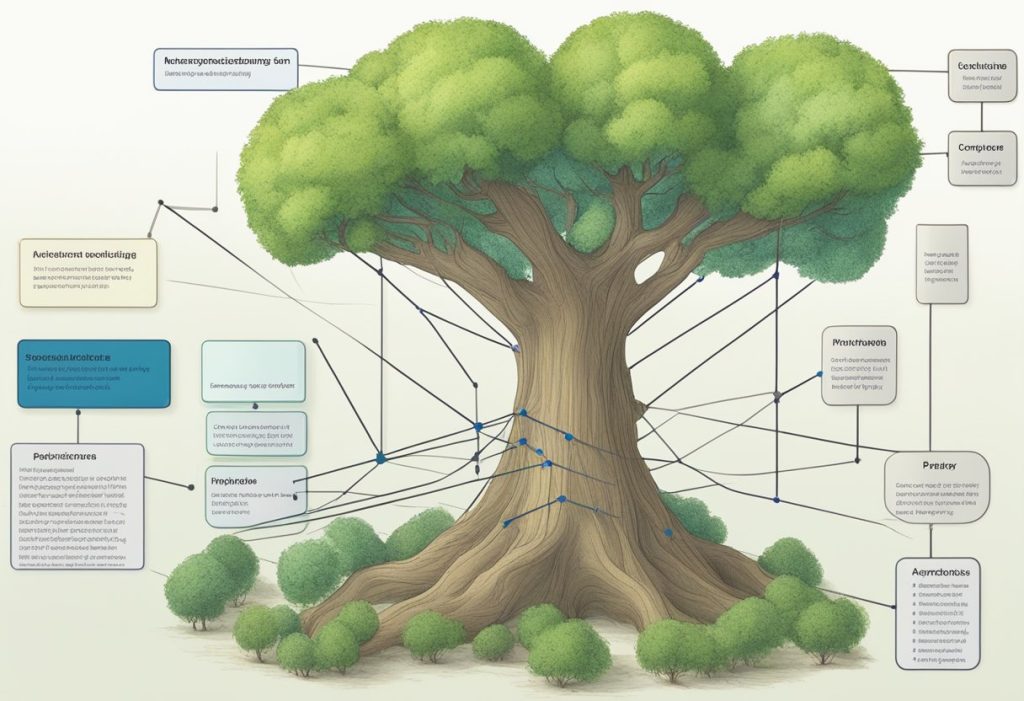
Diving into Python’s advanced data structures, you’ll discover how trees, heaps, and graphs optimize your programs, especially when dealing with complex data sets.
These structures are key in areas like data science and efficient programming due to their ability to handle hierarchical data, prioritize tasks, and model intricate relationships.
Tree Structures for Hierarchical Data
Trees are indispensable when you’re working with data that naturally forms a hierarchy. A prime example is the binary search tree (BST), where each node has at most two children; this setup allows for efficient searching and sorting of data. You may encounter variations like red-black trees, which maintain balance after insertions and deletions to ensure operations stay efficient.
For data science applications involving decisions, don’t overlook the utility of decision trees, an algorithm that discriminates data points based on features.
- Common Tree Operations:
- Insert: Add a new node
- Delete: Remove a node
- Search: Find a node
Using trees, you establish a structure conducive to advanced algorithms that can navigate and manipulate entries memory-efficiently.
Heaps for Priority Queue Implementations
For implementing prioritized tasks, heaps are your go-to. A binary heap ensures that the parent node is either greater than or equal to (in a max heap) or less than or equal to (in a min heap) its child nodes.
Heaps excel in applications requiring regular access to the ‘largest’ or ‘smallest’ element, such as in heap sort or priority queues used in advanced scheduling algorithms.
- Key Heap Invariants:
- Max Heap: Parent ≥ Children
- Min Heap: Parent ≤ Children
By utilizing heaps, you’re not only facilitating efficiency in program execution but also optimizing memory usage.
Graphs for Complex Relationship Modeling
Graphs enable you to represent and analyze complex relationships with nodes, also known as vertices, connected by edges. For something like social network analysis or route navigation, you’ll likely use a directed graph, where edges have a direction. In contrast, an undirected graph allows for two-way relationships, as seen in undirected networks.
When performance is critical, algorithms such as Dijkstra’s for shortest path or A search* come into play, providing you pathways to solve complex data sets efficiently.
- Types of Graphs & Their Applications:
- Directed Graphs: For one-way relationships
- Undirected Graphs: For mutual associations
- Weighted Graphs: Includes cost of transition between nodes
Incorporating graphs into your applications bolsters your ability to manage and interpret relationships within sizeable datasets effectively.
Data Structures in Practical Applications
Data structures are your toolkit for managing complexity. They help you optimize data interactions specific to your application’s needs, making them crucial in various fields like web development and machine learning.
Web Development and Data Structures
In web development, the choice of data structures significantly impacts your site’s performance and efficiency.
Consider a social media platform where dictionaries and hash tables are used to store user profiles with quick lookup times. When dealing with real-time data, like in chat applications, queues support the FIFO (First In, First Out) protocol, ensuring that messages are received and sent in the correct order.
Stacks are handy in undo features within text editors or browsers, as they allow users to revert actions in a LIFO (Last In, First Out) manner.
Meanwhile, implementing graphs can help model complex relationships between entities, such as the network of friends in a social media app.
Machine Learning’s Data Needs
Machine learning algorithms rely heavily on data structures for data pre-processing, analysis, and model building. Arrays and matrices are the backbone for numerical computations, often used to store feature data and labels in a structured form. Efficient manipulation of these structures is key, as operations on high-dimensional data can become costly.
Complex algorithms require trees or graphs to model decisions and hierarchies, like in decision trees or neural networks.
The use of priority queues or heaps is crucial in optimization problems and algorithms that need to regularly access the smallest or largest element, like in the case of algorithm classifiers.
Improving Code with Data Structures

Incorporating advanced data structures into your code not only boosts its efficiency but also enhances readability and maintainability. As you work through more complex algorithms, your choice of data structures can greatly influence the performance and quality of your applications.
Readability and Maintainability
Selecting the right data structures is pivotal to writing code that you and others can understand and alter without an excessive headache. It’s like choosing a toolbox for a job—pick the right tools and you’ll work smarter, not harder.
For instance, while implementing a feature that requires frequent item lookups, using a dictionary can be a far more readable and efficient choice than a list, due to its constant-time lookup capabilities.
Another key to maintaining your code is making it reusable. Generic data structures such as queues and stacks enable this by abstracting complexity, allowing you to apply the same concepts across different problems.
These structures, when well-implemented, become plug-and-play components that can significantly cut down on the time you spend debugging and reworking your code.
Frequently Asked Questions

In this section, you’ll find answers to some common inquiries regarding advanced data structures in Python that can enhance your programming efficiency.
What are some examples of advanced data structures used for efficiency in Python?
Advanced data structures such as B-Trees, Red-Black Trees, Heaps, and Graphs are used in Python to manage data more efficiently. These structures can handle large amounts of data with operations that allow for faster retrieval, insertion, and sorting compared to basic structures like lists or dictionaries.
How can implementing advanced data structures improve program efficiency?
Using advanced data structures can significantly reduce the time complexity of operations. For example, structures like Heaps provide efficient priority queue implementation, enabling quick access to the highest or lowest elements.
What is considered the most efficient data structure for handling large datasets in Python?
The efficiency of data structures can depend on the type of operations required. However, for handling large datasets, Hash Tables or Dictionaries in Python are often considered efficient in terms of average time complexity for search, insert, and delete operations.
Can you provide a comparison of efficiency between standard and advanced data structures in Python?
Advanced data structures often offer more efficient algorithms than standard data structures for specific tasks. For example, while a list might offer O(n) search time complexity, a Binary Search Tree can offer O(log n) complexity, making it significantly faster for search operations in sorted data.
Where can I find practical examples of complex data structures implemented in Python?
You can find practical examples of complex data structures implemented in Python on various programming platforms. Explore articles like “Exploring Advanced Data Structures in Python: A Comprehensive Guide” on Medium for use cases and code snippets.
What resources or books would you recommend for learning about advanced data structures in Python?
For those looking to deepen their understanding of advanced data structures in Python, resources such as “Python Data Structures Explained: Unlock the Secrets to Efficient Programming” and the book “Problem Solving with Algorithms and Data Structures using Python” are highly recommended.
December 22, 2023 at 10:41PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
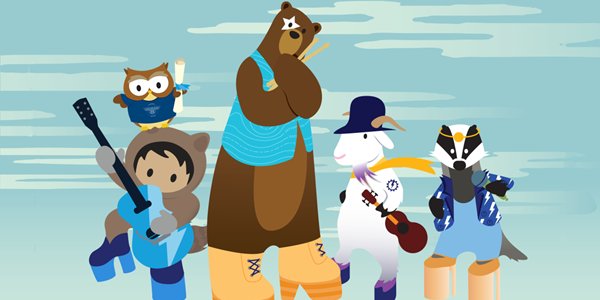
Post a Comment