Python Ternary For Loop: Simplifying Conditional Expressions in Iterations : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Python’s ternary operator, also known as the conditional operator, evaluates a condition and returns one of two values, depending on whether the condition is true or false. This useful feature enables you to write clean and concise Python code, using one line to achieve the functionality of an if-else statement.
Tip: Like a nested
if ... else
statement, you can use a nested ternary to express multiple conditions in a single line.
Python’s ternary operator is often used in conjunction with for
loops to create more compact and readable code.
When working with for
loops in Python, you might find yourself needing to apply different actions to elements in your loop based on specific conditions.
Instead of writing lengthy if-else statements within the loop, the ternary operator can streamline the process and make your code easier to understand.
Understanding Python Ternary For Loop

Basics of Ternary Operator
The ternary operator is a concise way to express a conditional expression in Python. It is essentially a one-line alternative to the traditional if-else statement. It evaluates a condition and returns one of the two possible values based on whether the condition evaluates to true or false.
Here’s a basic example:
result = "Even" if x % 2 == 0 else "Odd"
In this case, the result
variable will be assigned the value "Even"
if x
is divisible by 2 and "Odd"
otherwise.
Syntax and Expressions of Ternary For Loop
A ternary for
loop in Python combines the concepts of the ternary operator and for loop to create a compact looping structure. It is not a built-in Python feature but can be achieved using a combination of assignment statements and conditional expressions.
The general syntax for a ternary for
loop is:
[expression_if_true if condition else expression_if_false for item in iterable]
Here’s an example that squares each element in a list if it’s even and adds 1 to each odd element:
numbers = [1, 2, 3, 4, 5] result = [x**2 if x % 2 == 0 else x + 1 for x in numbers]
In this case, the result
variable will be a new list with the transformed values [2, 4, 4, 16, 6]
.
Function of Lambda with Ternary Operator
The power of the ternary operator can be further enhanced by using it in conjunction with lambda functions.
Info: Lambda functions are small, anonymous functions that can accept multiple inputs and return a single expression. They are useful for quick in-line operations where a full function definition might be undesired or unnecessary.
The ternary operator can be incorporated into a lambda function like this:
(lambda x: x**2 if x % 2 == 0 else x + 1)
You can use lambda functions with ternary operators inside a for loop or with functions like map()
and filter()
to create more complex and efficient looping structures.
Here’s an example that squares each even element and increments each odd element in a list:
numbers = [1, 2, 3, 4, 5] result = list(map(lambda x: x**2 if x % 2 == 0 else x + 1, numbers))
The result, just like before, would be the transformed list [2, 4, 4, 16, 6]
.
Keep in mind that using ternary operators and lambda functions can reduce readability, so use them judiciously.
Practical Examples with Limitations
In Python, you can use ternary for loops with list comprehensions, tuples, dictionary, and lambda functions. However, there are some limitations to consider when using ternary operators in these scenarios. Let’s explore a few examples and discuss their readability,
Example 1: List Comprehension
Utilizing a ternary operator within list comprehension can be a concise way to handle conditional statements.
Here’s a basic implementation:
numbers = [1, 2, 3, 4, 5] result = [x if x % 2 == 0 else -x for x in numbers]
In this example, the result is [-1, 2, -3, 4, -5]
and each even number will remain the same, while the odd numbers are negated.
Even though this example is pretty simple, combining multiple expressions or nested ternary operators may reduce the readability.
Example 2: Tuple Unpacking
You can use tuples to incorporate ternary operators by using index-based access. However, make sure you understand that this method is not exactly the same as using a regular ternary operator.
Nevertheless, it provides a similar functionality:
greet = True greet_person = ('Bye', 'Hi')[greet]
In this case, greet_person
will equal 'Hi'
.
While this may look like a ternary operator, it is actually using tuple indexing. The greet
boolean variable is implicitly converted to an integer (False -> 0, True -> 1) and thus indexes the tuple accordingly.
Example 3: Dictionary and Lambda Function
When handling multiple conditions, a dictionary with lambda functions may offer a cleaner solution compared to a nested ternary operator.
For instance, consider the following age-based scenario:
age = 25 category = { 1: 'Child', 2: 'Teenager', 3: 'Adult' } age_group = category.get((lambda age: 1 if age < 13 else 2 if age < 18 else 3)(age), 'Unknown')
This will assign 'Adult'
to age_group
. Although it seems complex, once you are familiar with lambda functions, it can be more readable compared to a deeply nested ternary operator.
Disclaimer: While ternary operators provide a concise way of expressing conditional statements in Python, you should be aware of the potential limitations, especially when it comes to readability. Additionally, remember that ternary operators are unique to Python and may not be directly translatable to languages like Java. Ensure that your usage of ternary operators is successful by maintaining simplicity and prioritizing clarity over brevity.
Frequently Asked Questions
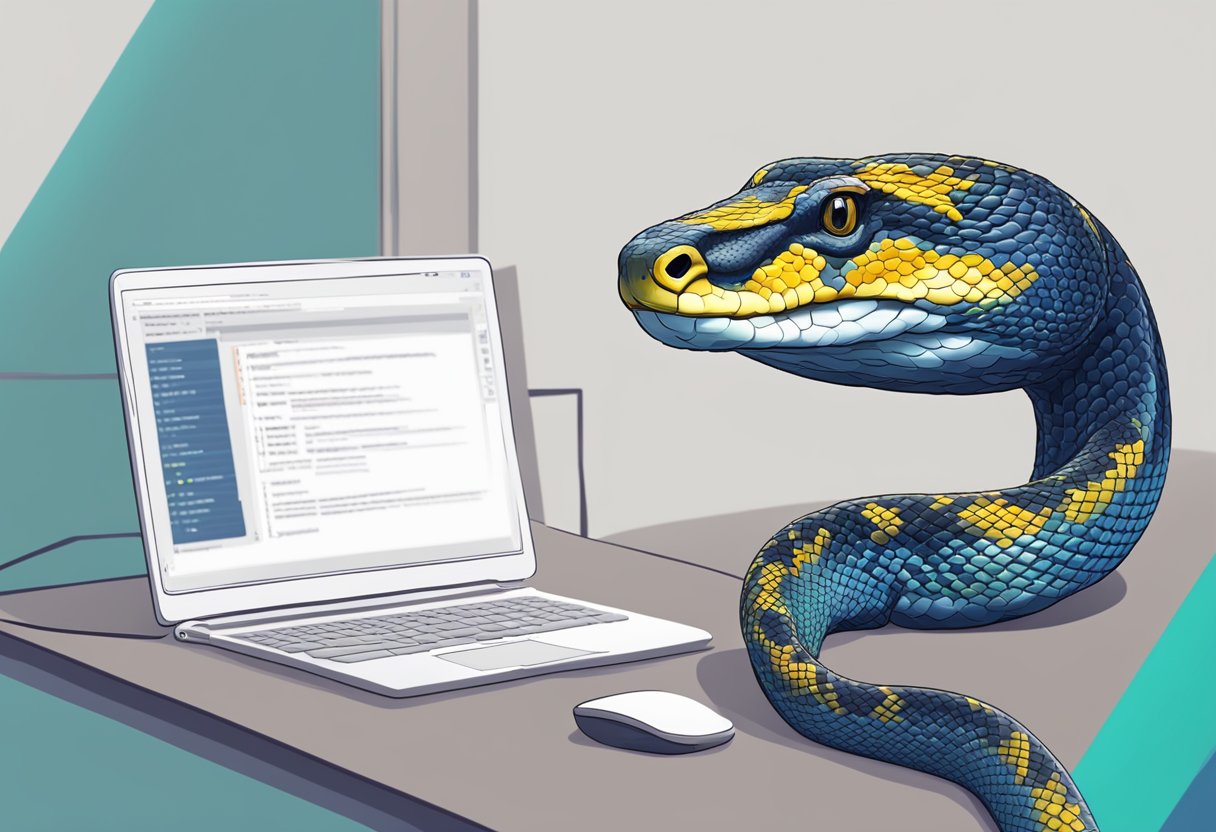
How do you use a ternary operator in Python list comprehension?
You can use a ternary operator in Python list comprehension to create a new list with elements based on a condition. Here’s the syntax:
new_list = [value_if_true if condition else value_if_false for item in old_list]
An example of using a ternary operator in list comprehension:
numbers = [1, 2, 3, 4, 5] squares = [x**2 if x % 2 == 0 else x for x in numbers]
What is the syntax for Python ternary conditional operator?
The syntax for the ternary conditional operator in Python follows this pattern:
value_if_true if condition else value_if_false
For example:
x = 10 y = 20 result = x if x > y else y
Can you apply ternary operators in function arguments in Python?
Yes, you can apply ternary operators in function arguments in Python. It allows you to conditionally pass different arguments depending on a condition. For instance:
def greet(is_formal): return "Hello, Sir!" if is_formal else "Hi!" print(greet(True))
Are ternary operators faster than if-else in Python?
In most cases, ternary operators and if-else statements have similar performance. Ternary operators can be faster and more concise in some cases, such as when used in list comprehensions. However, the difference in performance is usually negligible and should not be the primary reason for using one over the other.
How to use ternary operator for conditional assignment in Python?
To use a ternary operator for conditional assignment in Python, follow this syntax:
variable = value_if_true if condition else value_if_false
For example, assigning the larger value to a variable:
x = 5 y = 10 larger_value = x if x > y else y
What is the equivalent of the null-conditional operator in Python?
In Python, you can use the ternary operator to achieve similar functionality to a null-conditional operator by checking for None
:
variable = value_if_not_none if variable is not None else default_value
For example, getting the length of a string or returning 0 if the string is None
:
text = None length = len(text) if text is not None else 0
To learn more about the ternary operator, check out our full tutorial on the Finxter blog.
Recommended: Python One Line Ternary
December 04, 2023 at 05:29PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
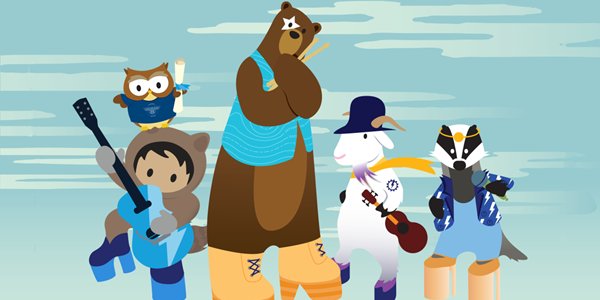
Post a Comment