✅ Solved – Python SyntaxError Unexpected EOF While Parsing : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Unexpected EOF means your Python interpreter reached the end of your program before all code is executed. In most cases, this error occurs if you don’t correctly declare or finish a for or while loop. Or you don’t close parentheses or (curly) brackets in a code block or dictionary definition.
In other words, some syntactical elements are missing! For example, a dictionary isn’t closed:
income = { 'alice': 121000, 'bob': 90000, 'carl': 423000
Another example of the SyntaxError Unexpected EOF while parsing is given here where the closing bracket of a list is missing:
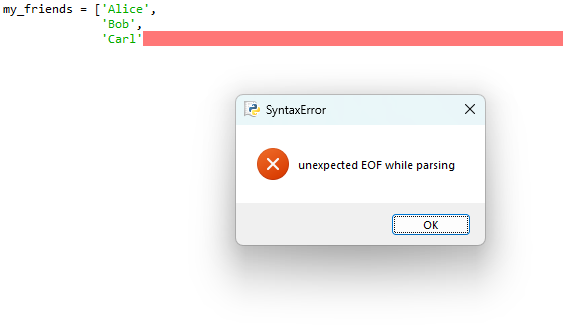
my_friends = ['Alice', 'Bob', 'Carl'
The “SyntaxError: unexpected EOF while parsing
” occurs when the Python interpreter reaches the end of your source code before all the code has been executed. It usually stems from a mistake in the structure or syntax of your code.
Missing or unclosed parentheses, brackets, braces, or quotes are common causes of the “unexpected EOF while parsing
” error.
Info: The
"EOF"
in this error message stands for “End of File”, which means the Python interpreter has reached the end of your code before executing all the lines. In such cases, the interpreter cannot parse the code correctly, resulting in the unexpected EOF while parsing error.
There are a few primary reasons for encountering the unexpected EOF error:
- Missing closing parentheses or brackets: If you forget to close an opening parenthesis, square bracket, or curly bracket, the interpreter will not be able to identify the end of the code block. As a result, an unexpected EOF error will be triggered.
# Example of incorrect code data = [1, 2, 3 for item in data: print(item) # Example of correct code data = [1, 2, 3] for item in data: print(item)
- Incorrect indentation: Python relies on indentation to organize code blocks. If your code is improperly indented, it can cause an unexpected EOF error as the interpreter attempts to parse the complete code block.
# Example of incorrect code if x > 10: print("x is greater than 10") # Example of correct code if x > 10: print("x is greater than 10")
- Incomplete code structures: If you start a control structure, such as a loop or a conditional statement, but fail to provide a proper body for it, the interpreter will reach the end of the file while still expecting more code.
# Example of incorrect code for i in range(5): # Example of correct code for i in range(5): print(i)
A common cause of syntax errors in Python is improper indentation or unbalanced parentheses, brackets, or braces. To resolve these issues, you should make sure your code follows proper indentation rules and that all opening and closing delimiters (parentheses, brackets, braces) are correctly paired and positioned.
For example, when dealing with loops and conditions:
for i in range(5): if i % 2 == 0: print("Even number:", i) else: print("Odd number:", i)
Ensure that the logic within the for
loop and if
statement is indented consistently. This allows Python to correctly understand the code block’s scope.
Additionally, ensuring that all opening and closing parentheses are balanced is essential when working with functions or more complex expressions:
result = ((3 * (5 + 4) - 7) / 2)
In this expression, the parentheses are balanced and ordered properly, allowing for the correct evaluation of the calculation. However, when missing the final closing parenthesis, Python raises this SyntaxError unexpected EOF while parsing
:

Frequently Asked Questions

What causes a SyntaxError: unexpected EOF while parsing in Python?
Unexpected EOF (End of File) errors in Python occur when the parser encounters an incomplete code construct or when the source code ends before all code constructs have been properly closed. The error indicates that there’s a mistake with the syntax or structure of your code, such as a missing closing parenthesis, bracket, or quotation mark, or an indentation issue.
How to fix the SyntaxError: unexpected EOF while parsing error in Python code?
To fix the unexpected EOF error in Python, you need to identify the code construct with incorrect syntax and add any missing elements to make the syntax correct. Carefully go through your code to check for any missing or improperly placed parentheses, brackets, or quotation marks. Also, ensure that your code is properly indented. The Python interpreter’s error message typically gives you an idea about the line of code where the issue has been encountered.
Are there specific programming constructs that can trigger an unexpected EOF error in Python?
Certain programming constructs in Python, including loops, conditionals, and function or class definitions, are more prone to cause unexpected EOF errors if not closed or indented properly. Misplaced parentheses, brackets, or quotation marks can also lead to this error.
What are common debugging techniques for resolving unexpected EOF errors in Python?
Some common debugging techniques for resolving unexpected EOF errors in Python include:
- Inspecting the line of code mentioned in the error message and looking for any syntax issues
- Making sure all code constructs, such as loops and conditionals, are appropriately closed and indented
- Checking for any unclosed parentheses, brackets, or quotation marks
- Walking through your code construct by construct to rule out errors.
How does Python handle unterminated string literals and missing parentheses in relation to unexpected EOF errors?
Unterminated string literals and missing parentheses often result in unexpected EOF errors in Python. If you forget to close a string literal with a matching quotation mark, Python will consider the rest of the code as part of the string, leading to an EOF error. In the case of unbalanced parentheses, Python will continue searching for the closing character until the end of the file, causing the error.
Can IDEs or code editors help in identifying and correcting SyntaxError: unexpected EOF while parsing issues?
Yes, IDEs (Integrated Development Environments) or code editors with Python support can help you identify and correct SyntaxError: unexpected EOF while parsing issues. These tools often have built-in syntax highlighting, code completion, and error detection features that can assist in identifying the source of the issue and suggesting potential fixes as you type your code. Examples of such IDEs and code editors include PyCharm, Visual Studio Code, and Sublime Text.
Recommended: PyCharm – A Simple Illustrated Guide
November 30, 2023 at 05:50PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
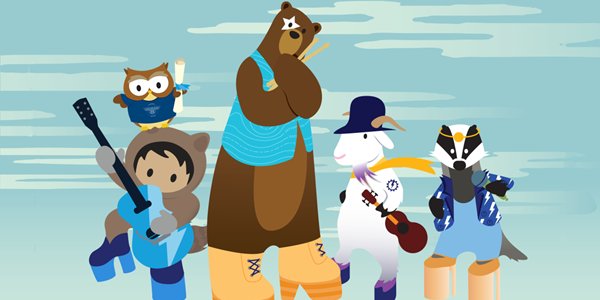
Post a Comment