(Fixed) Python TypeError: String Indices Must Be Integers : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
In Python, the “TypeError: string indices must be integers
” error typically occurs when a string type object is accessed with non-integer indices. This error often arises in situations involving data structures like lists and dictionaries.
Understanding the Error
This error signifies that the code attempts to index a string using a non-integer type, like a string or object. Since strings in Python are arrays of bytes representing Unicode characters, they must be indexed by integers, each pointing to a position in the string.
Solution 1: Correct Indexing
Often, the error arises from incorrectly indexing a string when the intention was to index a list or dictionary.
For instance:
data = {'name': 'Alice', 'age': 30} print(data['name'][0]) # Correct print(data[0]) # TypeError or KeyError
In this example, data[0]
is incorrect since data
is a dictionary, not a list. The right way is to access the dictionary values using their keys.
Solution 2: Data Type Validation
Before indexing, ensure the variable is of the expected type. This can be done using the type()
function or isinstance()
method.
Example:
data = 'Hello' if isinstance(data, str): print(data[0]) else: print("Not a string!")
Solution 3: Using Try-Except Blocks
Handling the error gracefully using a try-except block can prevent the program from crashing:
try: data = 'Hello' print(data['0']) except TypeError: print("Index must be an integer")
Solution 4: Data Parsing and Conversion
In situations involving JSON or similar data formats, ensure the data is correctly parsed and converted into the appropriate type:
import json json_data = '{"name": "Alice", "age": 30}' data = json.loads(json_data) print(data['name']) # Correct
Solution 5: Looping Over Strings
When iterating over a string, it’s common to use a loop. Ensure to use the characters directly or their integer indices:
for i, char in enumerate('Hello'): print(f"Character at {i} is {char}")
November 20, 2023 at 03:42AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
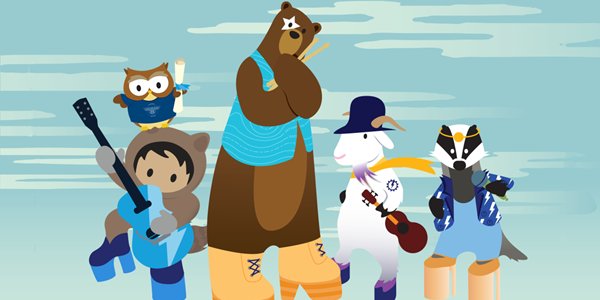
Post a Comment