Build a Hangman Game for the Command Line in Python :
by:
blow post content copied from Real Python
click here to view original post
Many people learn basic language and word skills by playing the game of hangman. First mentioned in print in the late nineteenth century and popularized in international media as the game show Wheel of Fortune, hangman appeals to many people. For beginning programmers who want a challenge or for more experienced coders looking for a bit of fun, writing hangman in Python is a rewarding endeavor.
Throughout this tutorial, you’ll build the hangman game in Python in a series of steps. The game will work as a command-line application. Throughout the process, you’ll also learn the basics of building computer games.
In this tutorial, you’ll:
- Learn the common elements of a computer game
- Track the state of a computer game
- Get and validate the user’s input
- Create a text-based user interface (TUI) for your game
- Figure out when the game is over and who the winner is
To get the most from this tutorial, you should be comfortable working with Python sets and lists. You don’t need to have any previous knowledge about game writing.
All the code that you’ll write in this tutorial is available for download at the link below:
Get Your Game: Click here to download the free code that you’ll write to create a hangman game with Python.
Demo: Command-Line Hangman Game in Python
In this tutorial, you’ll write a hangman game for your command line using Python. Once you’ve run all the steps to build the game, then you’ll end up with a text-based user interface (TUI) that will work something like the following:
Throughout this tutorial, you’ll run through several challenges designed to help you solve specific game-related problems. At the end, you’ll have a hangman game that works like the demo above.
Project Overview
The first step to building a good computer game is to come up with a good design. So, the design for your hangman game should begin with a good description of the game itself. You also need to have a general understanding of the common elements that make up a computer game. These two points are crucial for you to come up with a good design.
Description of the Hangman Game
While many people know the game well, it’s helpful to have a formal description of the game. You can use this description to resolve programming issues later during development. As with many things in life, the exact description of a hangman game could vary from person to person. Here’s a possible description of the game:
- Game setup: The game of hangman is for two or more players, comprising a selecting player and one or more guessing players.
- Word selection: The selecting player selects a word that the guessing players will try to guess.
- The selected word is traditionally represented as a series of underscores for each letter in the word.
- The selecting player also draws a scaffold to hold the hangman illustration.
- Guessing: The guessing players attempt to guess the word by selecting letters one at a time.
- Feedback: The selecting player indicates whether each guessed letter appears in the word.
- If the letter appears, then the selecting player replaces each underscore with the letter as it appears in the word.
- If the letter doesn’t appear, then the selecting player writes the letter in a list of guessed letters. Then, they draw the next piece of the hanged man. To draw the hanged man, they begin with the head, then the torso, the arms, and the legs for a total of six parts.
- Winning conditions: The selecting player wins if the hanged man drawing is complete after six incorrect guesses, in which case the game is over. The guessing players win if they guess the word.
- If the guess is right, the game is over, and the guessing players win.
- If the guess is wrong, the game continues.
A game in progress is shown below. In this game, the word to be guessed is hangman:
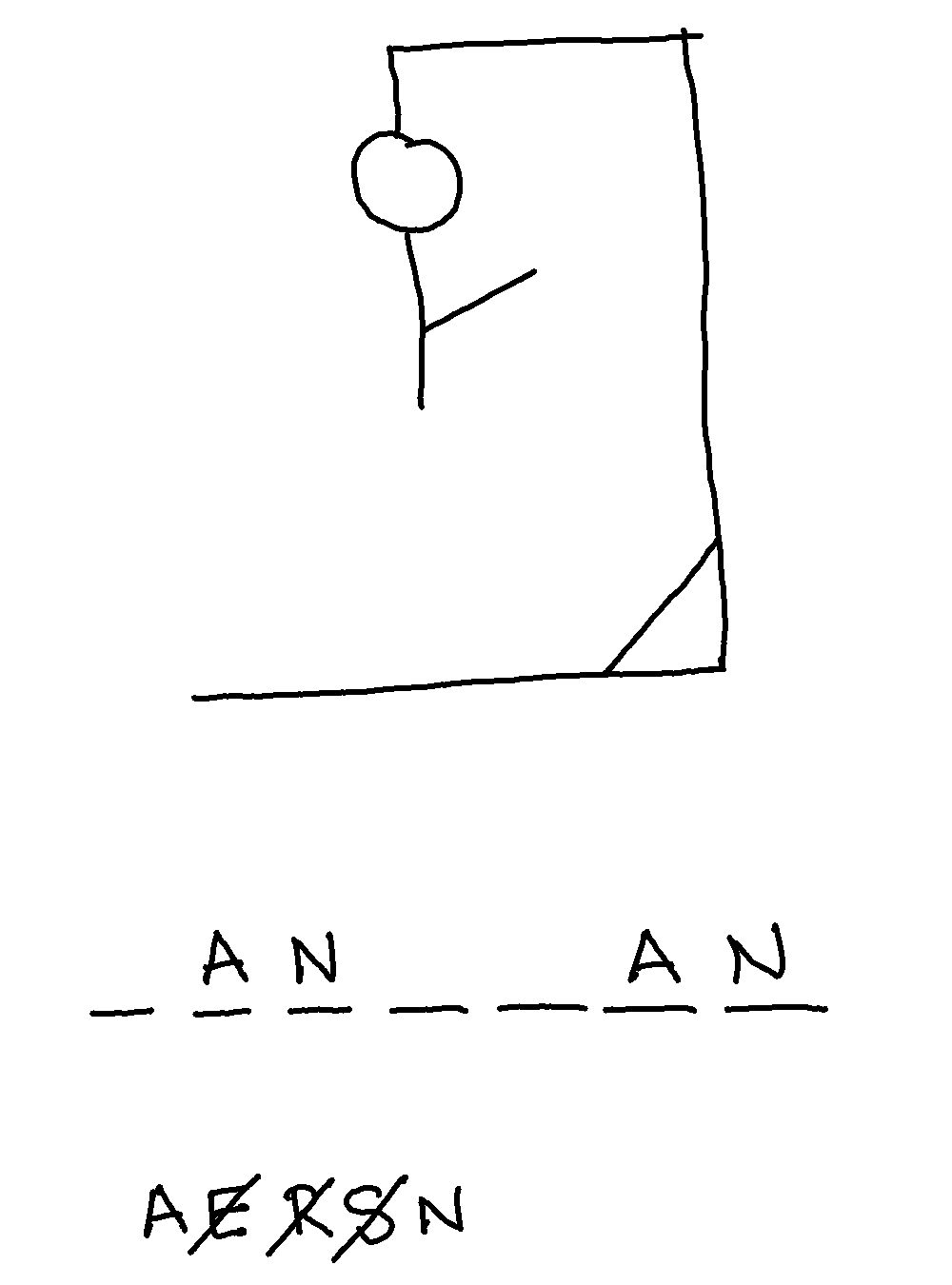
In this tutorial, to write the hangman game in Python, you’ll make a few additional design decisions:
- The game will be between the computer and one human player.
- The computer will act as the selecting player and will select the word to guess, process human input, and handle all output.
- The human player is the guessing player, hereafter simply referred to as the player. When the player knows the word, they continue to guess correct letters until the word is complete.
With this basic understanding of the game and some design decisions for the computer version, you can begin creating the game. First, however, you need to know the common elements of computer games and understand how they interact to produce the desired result.
Common Elements of a Computer Game
Every game, whether it’s a computer game or not, has a set of elements that make it a game. Without these elements, there’s no game:
- Initial setup: You get the game ready to play. This might mean setting the pieces on a chess board, dealing out cards, or rolling dice to see who goes first.
- Gameplay: When people think of the game, this is what they normally think of. The flow of gameplay is controlled by a game loop, which keeps the game moving and doesn’t end until the game is over. The game loop ensures the following happens:
- The user input is gathered and processed.
- The game state is updated based on reactions to the user input. This may include checking for end-of-game conditions.
- Any required output changes are made to reflect the new game state.
- The game loop repeats.
- Game ending: How the game ends depends on the game itself. Whether capturing the enemy king in checkmate, achieving a certain score in a card game, or having your piece cross the line in a board game, certain conditions determine the end of the game and the winner.
All games have a game loop. For example, in a chess game, you first set the pieces on the board and declare the current player. Then the game loop begins:
Read the full article at https://realpython.com/python-hangman/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
November 01, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
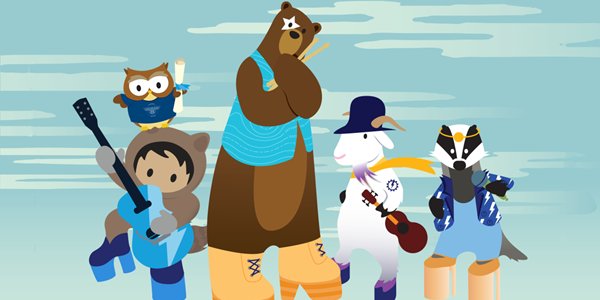
Post a Comment