Python – How to Open a File by Regular Expression? : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
In Python, you can use the glob
module’s glob.glob(pattern)
function to find all the pathnames matching a specified pattern
(e.g., '*.py'
) according to the rules used by the Unix shell, which includes functionality for wildcard matching that can be used similarly to regular expressions for filename matching.
Here’s a minimal example that filters out all Python files from my Desktop *.py
:
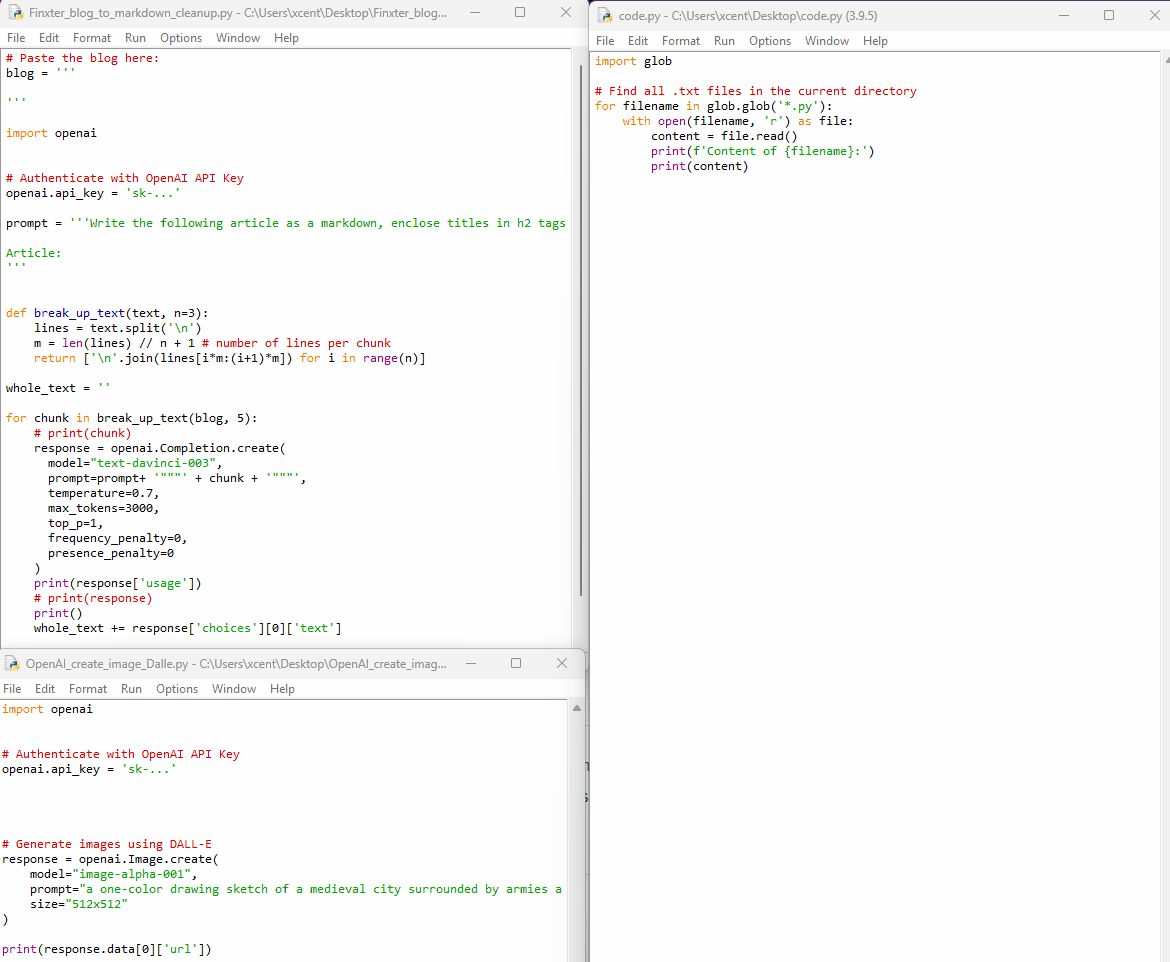
import glob # Find all .txt files in the current directory for filename in glob.glob('*.py'): with open(filename, 'r') as file: content = file.read() print(f'Content of {filename}:') print(content)
If you need more advanced pattern matching, you can use the re
module along with os.listdir()
or os.scandir()
to filter filenames using regular expressions.
Here’s a minimal example of a file that reads itself:

import re import os # Regular expression pattern to match filenames pattern = re.compile(r'co.*\.py') # List all files in the current directory for filename in os.listdir('.'): if pattern.match(filename): with open(filename, 'r') as file: content = file.read() print(f'Content of {filename}:') print(content)
Thanks for reading! If you want to keep improving your coding skills and stay tuned with the recent developments in OpenAI API, Llama, and AI + Python, feel free to join my free email newsletter by downloading this cheat sheet:
The post Python – How to Open a File by Regular Expression? appeared first on Be on the Right Side of Change.
October 06, 2023 at 04:39PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
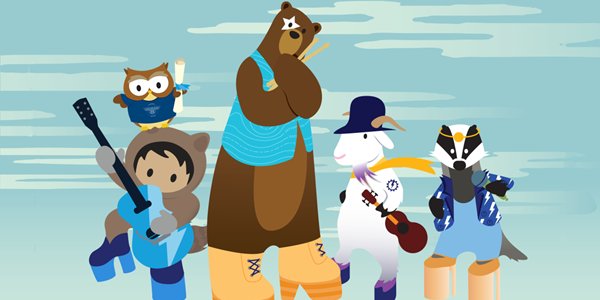
Post a Comment