Python hasattr() : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Python’s built-in hasattr(object, string)
function takes an object
and a string
as an input. It returns True
if one of the object
‘s attributes has the name given by the string
. Otherwise, it returns False
.
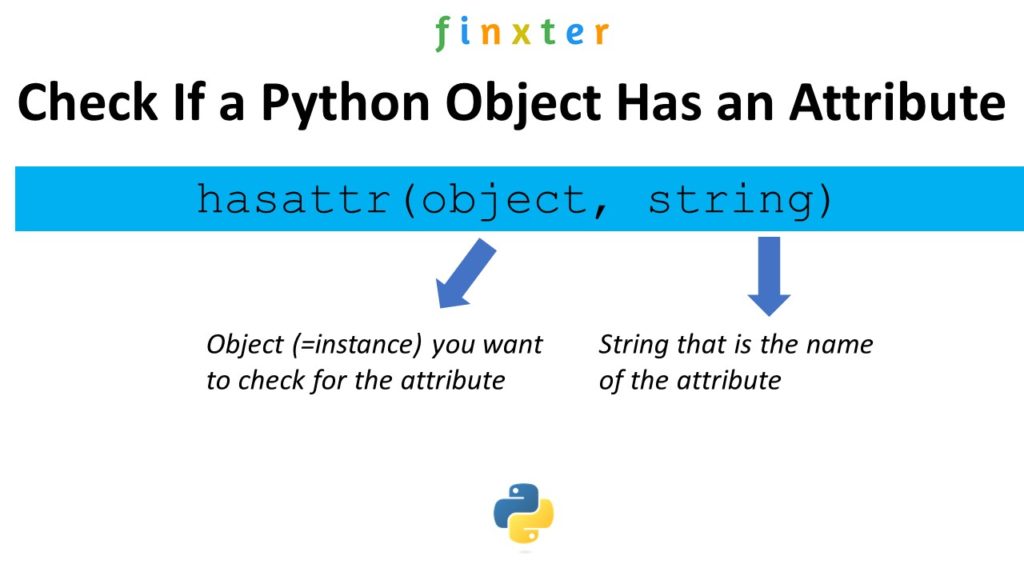
Usage
Learn by example! Here’s an example on how to use the hasattr()
built-in function.
# Define class with one attribute class Car: def __init__(self, brand): self.brand = brand # Create object porsche = Car('porsche') # Check if porsche has attributes print('Porsche has attribute "brand": ', hasattr(porsche, 'brand')) print('Porsche has attribute "color": ', hasattr(porsche, 'color'))
The output of this code snippet is:
Porsche has attribute "brand": True Porsche has attribute "color": False
It has the attribute “brand” but not the attribute “color”.
Video hasattr()

Syntax hasattr()
The hasattr()
object has the following syntax:
Syntax:
hasattr(object, attribute) # Does the object have this attribute?
Arguments | object |
The object from which the attribute value should be drawn. |
attribute |
The attribute name as a string. | |
Return Value | object |
Returns Boolean whether the attribute string is the name of one of the object ‘s attributes. |
Return value from hasattr()
The hasattr(object, attribute)
method returns True
, if the object has the attribute and False
otherwise.
Interactive Shell Exercise: Understanding hasattr()
Consider the following interactive code:
Exercise: Fix the code so that both results of hasattr()
return True
!
Check out my new Python book Python One-Liners (Amazon Link).
If you like one-liners, you’ll LOVE the book. It’ll teach you everything there is to know about a single line of Python code. But it’s also an introduction to computer science, data science, machine learning, and algorithms. The universe in a single line of Python!
The book was released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Publisher Link: https://nostarch.com/pythononeliners
Applications hasattr()
- You can use
hasattr()
to avoid accessing errors when trying to access an attribute of a dynamic object. - You can use
hasattr()
in a ternary operator to conditionally assign a value to a variable such as in:age = object.age if hasattr(object, 'age') else 0
- However, be careful when using
hasattr()
as it always returnFalse
, no matter the error message. Thus, it may overshadow an error different to the error that appears if the attribute doesn’t exist. So, the attribute may indeed exist but if trying to access it causes an error, the result will beFalse
.
Frequently Asked Questions (FAQs)
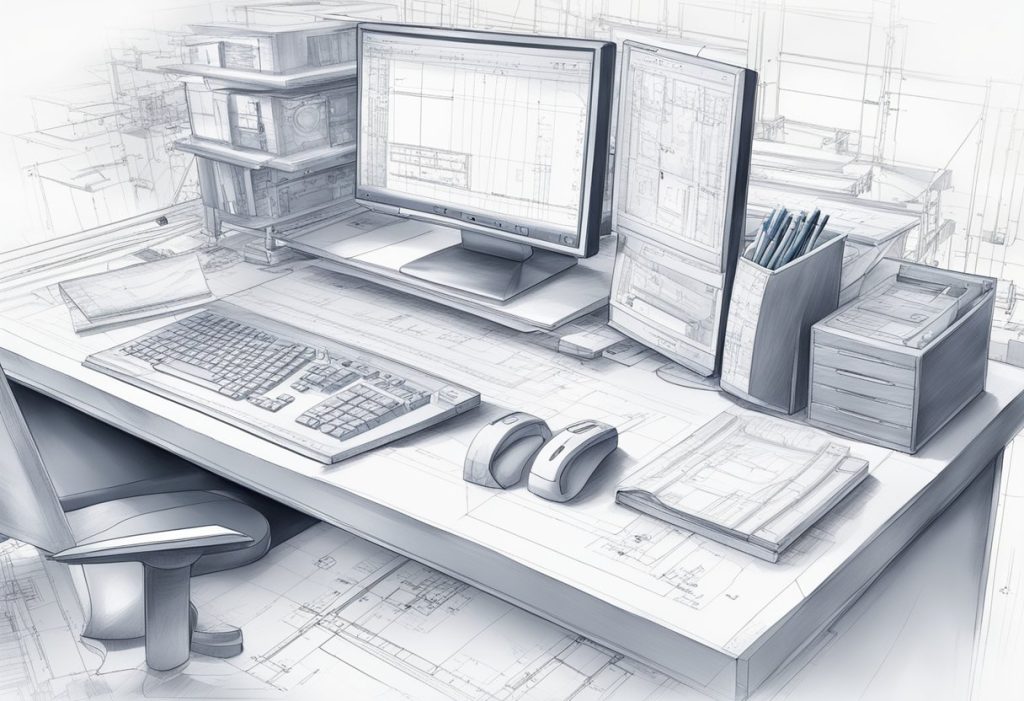
How Does hasattr() Function Check Attributes in Object’s Class and Its Superclasses?
The hasattr()
function in Python is used to determine whether an object has a named attribute. It takes two arguments: the object and a string representing the name of the attribute you are checking for.
Here’s how the process works:
- Local Attributes Check: Initially,
hasattr()
checks whether the specified attribute name exists in the namespace of the object itself. - Inheritance Check (Including Superclasses): If the attribute is not found in the local namespace,
hasattr()
will proceed to check in the namespaces of any superclasses from which the object’s class inherits, following the method resolution order (MRO). This way, it can detect attributes inherited from superclasses. - Descriptor Protocol: If the class has defined
__getattr__()
or__getattribute__()
methods, these methods are invoked as part of the attribute lookup process. If these methods raise anAttributeError
,hasattr()
will returnFalse
; otherwise, it will returnTrue
.
In essence, hasattr()
provides a comprehensive check for attribute presence, encompassing both the local class namespace and inherited namespaces from superclasses.
This behavior ensures that hasattr()
can accurately reflect the attribute lookup process that Python would perform during regular attribute access, making it a reliable tool for introspective operations in your code.
If you want to learn more about namespaces, feel free to read our tutorial and watch the associated video:

Recommended: Understanding Namespaces in Python
What is the hasattr()
function used for in Python?
The hasattr()
function is handy for checking if a particular object has a given named attribute before attempting to access it. This can prevent runtime errors that would occur if you tried to access an attribute that doesn’t exist. Here’s how you’d use it:
class Dog: def __init__(self, name): self.name = name fido = Dog('Fido') # Check if fido has a 'name' attribute print(hasattr(fido, 'name')) # Output: True # Check if fido has a 'bark' attribute print(hasattr(fido, 'bark')) # Output: False
How does hasattr()
differ from getattr()
?
While hasattr()
checks if an object has a certain attribute, getattr()
attempts to retrieve the value of that attribute. If the attribute doesn’t exist, getattr()
will raise an AttributeError
, unless a default value is provided as a third argument. Here’s a comparison:
class Cat: def __init__(self, name): self.name = name whiskers = Cat('Whiskers') # Using hasattr() print(hasattr(whiskers, 'name')) # Output: True print(hasattr(whiskers, 'meow')) # Output: False # Using getattr() print(getattr(whiskers, 'name')) # Output: 'Whiskers' print(getattr(whiskers, 'meow', 'default_value')) # Output: 'default_value'
Can hasattr()
check for method existence?
Absolutely! hasattr()
can be used to check if an object has a specific method. Methods are just attributes that are callable. Here’s a simple example:
class Bird: def chirp(self): print('Chirp chirp!') tweety = Bird() # Check if tweety has a 'chirp' method print(hasattr(tweety, 'chirp')) # Output: True
What happens with hasattr()
if an attribute is set to None
?
Even if an attribute is set to None
, hasattr()
will still return True
, as the attribute does exist on the object—it just has a value of None
. Here’s an illustration:
class Fish: def __init__(self, name=None): self.name = name nemo = Fish() # Check if nemo has a 'name' attribute print(hasattr(nemo, 'name')) # Output: True
Related Functions
- The
getattr()
function returns the value of an attribute. - The
setattr()
function changes the value of an attribute. - The
hasattr()
function checks if an attribute exists. - The
delattr()
function deletes an existing attribute.
Summary
Python’s built-in hasattr(object, string)
function takes an object
and a string
as an input.
- It returns
True
if one of theobject
‘s attributes has the name given bystring
. - It returns
False
otherwise if one of theobject
‘s attributes doesn’t have the name given bystring
.
>>> hasattr('hello', 'count') True >>> hasattr('hello', 'xxx') False
Note that hasattr()
also returns True
if the string is the name of a method rather than an attribute.
I hope you enjoyed the article! To improve your Python education, you may want to join the popular free Finxter Email Academy:
Do you want to boost your Python skills in a fun and easy-to-consume way? Consider the following resources and become a master coder!
Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
If your answer is YES!, consider becoming a Python freelance developer! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
The post Python hasattr() appeared first on Be on the Right Side of Change.
October 23, 2023 at 03:25PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
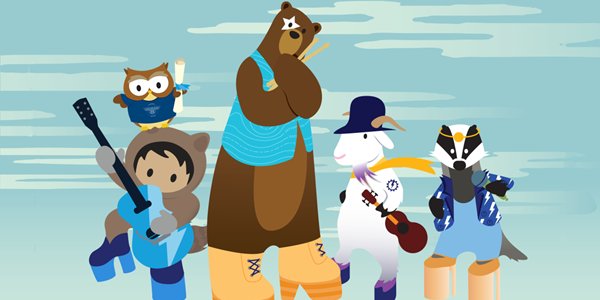
Post a Comment