How to Use Type Hints for Multiple Return Types in Python :
by:
blow post content copied from Real Python
click here to view original post
In Python, type hinting is an optional yet useful feature to make your code easier to read, reason about, and debug. With type hints, you let other developers know the expected data types for variables, function arguments, and return values. As you write code for applications that require greater flexibility, you may need to specify multiple return types to make your code more robust and adaptable to different situations.
You’ll encounter different use cases where you may want to annotate multiple return types within a single function in Python. In other words, the data returned can vary in type. In this tutorial, you’ll walk through examples of how to specify multiple return types for a function that parses a string from an email address to grab the domain name.
In addition, you’ll see examples of how to specify type hints for callback functions or functions that take another function as input. With these examples, you’ll be ready to express type hints in functional programming.
Note: Typically, you want to work with functions that are generous in which type of arguments they accept, while they’re specific about the type of their return value. For example, a function may accept any iterable like a list, tuple, or generator, but always return a list.
If your function can return several different types, then you should first consider whether you can refactor it to have a single return type. In this tutorial, you’ll learn how to deal with those functions that need multiple return types.
To get the most out of this tutorial, you should know the basics of what type hints in Python are and how you use them.
Get Your Code: Click here to get access to the free sample code that shows you how to declare type hints for multiple types in Python.
Use Python’s Type Hints for One Piece of Data of Alternative Types
In this section, you’ll learn how to write type hints for functions that can return one piece of data that could be of different types. The scenarios for considering multiple return types include:
-
Conditional statements: When a function uses conditional statements that return different types of results, you can convey this by specifying alternative return types for your function using type hints.
-
Optional values: A function may sometimes return no value, in which case you can use type hints to signal the occasional absence of a return value.
-
Error handling: When a function encounters an error, you may want to return a specific error object that’s different from the normal results’ return type. Doing so could help other developers handle errors in the code.
-
Flexibility: When designing and writing code, you generally want it to be versatile, flexible, and reusable. This could mean writing functions that can handle a range of data types. Specifying this in type hints helps other developers understand your code’s versatility and its intended uses in different cases.
In the example below, you use type hints in working with conditional statements. Imagine that you’re processing customer data and want to write a function to parse users’ email addresses to extract their usernames.
To represent one piece of data of multiple types using type hints in Python 3.10 or newer, you can use the pipe operator (|
). Here’s how you’d use type hints in a function that typically returns a string containing the username but can also return None
if the corresponding email address is incomplete:
def parse_email(email_address: str) -> str | None:
if "@" in email_address:
username, domain = email_address.split("@")
return username
return None
In the example above, the parse_email()
function has a conditional statement that checks if the email address passed as an argument contains the at sign (@
). If it does, then the function splits on that symbol to extract the elements before and after the at sign, stores them in local variables, and returns the username. If the argument doesn’t contain an at sign, then the return value is None
, indicating an invalid email address.
Note: In practice, the validation rules for email addresses are much more complicated.
So, the return value of this function is either a string containing the username or None
if the email address is incomplete. The type hint for the return value uses the pipe operator (|
) to indicate alternative types of the single value that the function returns. To define the same function in Python versions older than 3.10, you can use an alternative syntax:
from typing import Union
def parse_email(email_address: str) -> Union[str, None]:
if "@" in email_address:
username, domain = email_address.split("@")
return username
return None
This function uses the Union
type from the typing
module to indicate that parse_email()
returns either a string or None
, depending on the input value. Whether you use the old or new syntax, a union type hint can combine more than two data types.
Even when using a modern Python release, you may still prefer the Union
type over the pipe operator if your code needs to run in older Python versions.
Note: One challenge with functions that may return different types is that you need to check the return type when you call the function. In the examples above, you need to test whether you got None
when parsing the email address.
If the return type can be deduced from the argument types, then you can alternatively use @overload
to specify different type signatures.
Now that you know how to define a function that returns a single value of potentially different types, you can turn your attention toward using type hints to declare that a function can return more than one piece of data.
Use Python’s Type Hints for Multiple Pieces of Data of Different Types
Sometimes, a function returns more than one value, and you can communicate this in Python using type hints. You can use a tuple to indicate the types of the individual pieces of data that a function returns at once. In Python 3.9 and later, you can use built-in tuple
data structure. On older versions, you need to use typing.Tuple
in your annotations.
Now, consider a scenario where you want to build on the previous example. You aim to declare a function whose return value incorporates multiple pieces of data of various types. In addition to returning the username obtained from an email address, you want to update the function to return the domain as well.
Here’s how you’d use type hints to indicate that the function returns a tuple with a string for the username and another string for the domain:
def parse_email(email_address: str) -> tuple[str, str] | None:
if "@" in email_address:
username, domain = email_address.split("@")
return username, domain
return None
Read the full article at https://realpython.com/python-type-hints-multiple-types/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
October 30, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
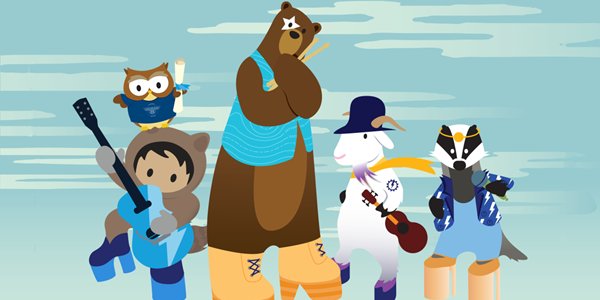
Post a Comment