How to Iterate Through a Dictionary in Python
by:
blow post content copied from Real Python
click here to view original post
Dictionaries are one of the most important and useful built-in data structures in Python. They’re everywhere and are a fundamental part of the language itself. In your code, you’ll use dictionaries to solve many programming problems that may require iterating through the dictionary at hand. In this tutorial, you’ll dive deep into how to iterate through a dictionary in Python.
Solid knowledge of dictionary iteration will help you write better, more robust code. In your journey through dictionary iteration, you’ll write several examples that will help you grasp the different ways to traverse a dictionary by iterating over its keys, values, and items.
In this tutorial, you’ll:
- Get to know some of the main features of dictionaries
- Iterate through a dictionary in Python by using different techniques and tools
- Transform your dictionaries while iterating through them in Python
- Explore other tools and techniques that facilitate dictionary iteration
To get the most out of this tutorial, you should have a basic understanding of Python dictionaries, know how to use Python for
loops, and be familiar with comprehensions. Knowing other tools like the built-in map()
and filter()
functions and the itertools
and collections
modules is also a plus.
Get Your Code: Click here to download the sample code that shows you how to iterate through a dictionary with Python.
Take the Quiz: Test your knowledge with our interactive “Python Dictionary Iteration” quiz. Upon completion you will receive a score so you can track your learning progress over time:
Getting Started With Python Dictionaries
Dictionaries are a cornerstone of Python. Many aspects of the language are built around dictionaries. Modules, classes, objects, globals()
, and locals()
are all examples of how dictionaries are deeply wired into Python’s implementation.
Here’s how the Python official documentation defines a dictionary:
An associative array, where arbitrary keys are mapped to values. The keys can be any object with
__hash__()
and__eq__()
methods. (Source)
There are a couple of points to notice in this definition:
- Dictionaries map keys to values and store them in an array or collection. The key-value pairs are commonly known as items.
- Dictionary keys must be of a hashable type, which means that they must have a hash value that never changes during the key’s lifetime.
Unlike sequences, which are iterables that support element access using integer indices, dictionaries are indexed by keys. This means that you can access the values stored in a dictionary using the associated key rather than an integer index.
The keys in a dictionary are much like a set
, which is a collection of hashable and unique objects. Because the keys need to be hashable, you can’t use mutable objects as dictionary keys.
On the other hand, dictionary values can be of any Python type, whether they’re hashable or not. There are literally no restrictions for values. You can use anything as a value in a Python dictionary.
Note: The concepts and topics that you’ll learn about in this section and throughout this tutorial refer to the CPython implementation of Python. Other implementations, such as PyPy, IronPython, and Jython, could exhibit different dictionary behaviors and features that are beyond the scope of this tutorial.
Before Python 3.6, dictionaries were unordered data structures. This means that the order of items typically wouldn’t match the insertion order:
>>> # Python 3.5
>>> likes = {"color": "blue", "fruit": "apple", "pet": "dog"}
>>> likes
{'color': 'blue', 'pet': 'dog', 'fruit': 'apple'}
Note how the order of items in the resulting dictionary doesn’t match the order in which you originally inserted the items.
In Python 3.6 and greater, the keys and values of a dictionary retain the same order in which you insert them into the underlying dictionary. From 3.6 onward, dictionaries are compact ordered data structures:
>>> # Python 3.6
>>> likes = {"color": "blue", "fruit": "apple", "pet": "dog"}
>>> likes
{'color': 'blue', 'fruit': 'apple', 'pet': 'dog'}
Keeping the items in order is a pretty useful feature. However, if you work with code that supports older Python versions, then you must not rely on this feature, because it can generate buggy behaviors. With newer versions, it’s completely safe to rely on the feature.
Another important feature of dictionaries is that they’re mutable data types. This means that you can add, delete, and update their items in place as needed. It’s worth noting that this mutability also means that you can’t use a dictionary as a key in another dictionary.
Understanding How to Iterate Through a Dictionary in Python
Read the full article at https://realpython.com/iterate-through-dictionary-python/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
August 28, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
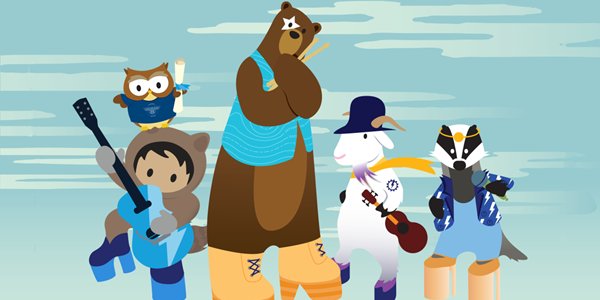
Post a Comment