Bitwise Operators in Python (AND, OR, XOR, NOT, SHIFT) : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Bitwise Operators in Python Overview
Bitwise operators in Python are used to perform bit-level operations on integers. They enable you to manipulate individual bits of data, providing an efficient way of handling binary data. The main bitwise operators in Python include AND, OR, XOR, NOT, and SHIFT.
The AND operator (&
) compares each bit of the first operand to the corresponding bit of the second operand. If both bits are 1, the corresponding result bit is set to 1. Otherwise, the result bit is set to 0:
a = 5 # Binary: 0101 b = 3 # Binary: 0011 c = a & b # Result: 1 (Binary: 0001)
The OR operator (|
) sets the result bit to 1 if at least one of the corresponding bits of the two operands is 1:
c = a | b # Result: 7 (Binary: 0111)
The XOR operator (^
) sets the result bit to 1 if the corresponding bits of the two operands are different. If they are the same, the result bit is set to 0:
c = a ^ b # Result: 6 (Binary: 0110)
The NOT operator (~
) inverts the bits of the operand, changing every 1 to 0 and vice versa:
c = ~a # Result: -6 (Binary representation depends on the integer type)
Shift operators are used to shift the bits of a number left or right, effectively multiplying or dividing the number by powers of 2.
The Left Shift operator (<<
) shifts the bits of the first operand to the left by the number of positions specified by the second operand:
c = a << 2 # Result: 20 (Binary: 10100)
The Right Shift operator (>>
) shifts the bits of the first operand to the right by the number of positions specified by the second operand:
c = a >> 1 # Result: 2 (Binary: 0010)
Using bitwise operators in Python can help optimize your code when working with binary data and allows you to perform operations at the most granular level.
Binary Representation and Conversion
In Python, bitwise operators are used to perform operations on individual bits of integers, which are represented using a binary number system. To perform bitwise operations, Python converts decimal integers into binary and applies the operators on corresponding pairs of bits. The result is then converted back to decimal format. You can perform operations such as AND, OR, XOR, NOT, and SHIFT using bitwise operators.
To convert a decimal integer to binary, you can use Python’s built-in bin()
function. It returns a binary string representation of the given number, with the prefix 0b
. You may also use oct()
for octal representation, which uses the prefix 0o
, and hex()
for hexadecimal representation prefixed with 0x
.
Here’s an example:
decimal_number = 42 binary_number = bin(decimal_number) octal_number = oct(decimal_number) hexadecimal_number = hex(decimal_number) print(binary_number) # Output: 0b101010 print(octal_number) # Output: 0o52 print(hexadecimal_number) # Output: 0x2a
Bitwise operators, such as AND (&
), OR (|
), XOR (^
), NOT (~
), Left Shift (<<
), and Right Shift (>>
), can be used to perform operations on these binary, octal, or hexadecimal representations.
To clarify the usage of bitwise operators, let’s look at an example with the AND operator:
x = 12 # 0b1100 y = 10 # 0b1010 result = x & y # bitwise AND operation print(result) # Output: 8 print(bin(result)) # Output: 0b1000
Remember, bitwise operators work only on integers.
Bitwise AND, OR, and XOR
Bitwise operators in Python are used to perform operations on binary numbers. The most common bitwise operators are AND, OR, and XOR. These operators work on integers, with each bit of the integer undergoing the specified operation.
Bitwise AND in Python is represented by the &
symbol. When doing the bitwise AND operation between two integers, the result will have its bits set to 1 only if both corresponding bits in the input integers are 1. Otherwise, the resulting bits will be 0.
Here’s an example:
a = 5 # 101 (binary) b = 3 # 011 (binary) result = a & b # 001 (binary), which is 1 in decimal

Full Guide: Python Bitwise AND Operator
Bitwise OR is represented by the |
symbol. During a bitwise OR operation, the result’s bits will be set to 1 if either of the corresponding bits in the input integers is 1. If both bits are 0, the resulting bit will also be 0.
a = 5 # 101 (binary) b = 3 # 011 (binary) result = a | b # 111 (binary), which is 7 in decimal

Full Guide: Python Bitwise OR Operator
Bitwise XOR is represented by the ^
symbol. In a bitwise XOR operation, the result’s bits will be set to 1 if the corresponding bits in the input integers are different. If the corresponding bits are the same, the resulting bit will be 0.
a = 5 # 101 (binary) b = 3 # 011 (binary) result = a ^ b # 110 (binary), which is 6 in decimal

Full Guide: Python Bitwise XOR Operator
When working with bitwise operators, it is important to remember that they only work on integers in Python. These operators provide a low-level means of manipulating binary data, which can be useful in various applications such as cryptography, error-detection algorithms, and more.
Bitwise NOT and Two’s Complement
Bitwise NOT, denoted by the ~
symbol in Python, is an operator that inverts all the bits in a given number. It is essential to understand how Python represents negative numbers using a method called two’s complement before diving deeper into the Bitwise NOT operation.
Two’s complement is a binary representation of signed integers, where the most significant bit (MSB) works as a sign bit. A 0
in the MSB means the number is positive, and a 1
means the number is negative. To find the two’s complement of a number, you can use the following steps:
- Invert all the bits in the binary representation of the number.
- Add
1
to the least significant bit (LSB) of the inverted binary representation.
For example, let’s find the two’s complement of -6
. First, represent 6
in binary: 0000 0110
. Next, invert all the bits: 1111 1001
. Finally, add 1
to the LSB: 1111 1010
. Therefore, -6
in two’s complement binary representation is 1111 1010
.
Now, let’s see an example of how the Bitwise NOT works:
a = 6 bitwise_not_a = ~a print(bitwise_not_a)
The output will be -7
. The binary representation of 6
is 0000 0110
. After performing the Bitwise NOT operation, the result is 1111 1001
, which is the two’s complement representation of -7
.
Returning to the topic of two’s complement, let’s see how to obtain the integer value back from its two’s complement representation. For instance, if we have the two’s complement binary representation 1111 1010
, follow these steps:
- Invert all bits in the two’s complement representation:
0000 0101
. - Add
1
to the LSB of the inverted bits:0000 0110
.
Following these steps, we obtain the positive integer 6
. As seen in this example, Python interprets the negative numbers using two’s complement, which is useful when working with bitwise operations, including the Bitwise NOT operator.
Shift Operators and Applications
Shift operators in Python, specifically bitwise left shift and bitwise right shift, are essential tools when working with binary data. They manipulate the bits within an integer, shifting them left or right and filling the empty bits with zeros.
The bitwise left shift (<<
) moves the bits of a number to the left by a specified number of positions:
result = number << shift_distance
For example, if number = 5
(binary 0b101
) and shift_distance = 2
, the resulting value will be 20
(binary 0b10100
). This operation effectively multiplies the original number by 2
raised to the power of the shift distance.
The bitwise right shift (>>
) does the reverse, moving the bits to the right:
result = number >> shift_distance
If number = 5
(binary 0b101
) and shift_distance = 1
, the result will be 2
(binary 0b10
). Bitwise right shift effectively performs integer division by 2
raised to the power of the shift distance.
These shift operators find various applications in areas such as data compression, network protocols, and encryption algorithms. For example, in cryptography, bitwise shifts are used to scramble data to make it harder to decipher.
Here is a simple example of using shift operators in Python to perform basic bit manipulations:
a = 7 # 0b0111 b = a << 2 # 0b0111 -> 0b11100 (equivalent to 7 * 2^2) c = b >> 1 # 0b11100 -> 0b1110 (equivalent to 28 // 2^1)
In this example, a
represents the initial value 7
(binary 0b0111
). b
demonstrates the use of the bitwise left shift to multiply a
by 4, resulting in a value of 28 (binary 0b11100
). Finally, c
applies a bitwise right shift to b
, dividing it by 2 and yielding a value of 14 (binary 0b1110
).
Bitwise Operator Overloading
Python offers the ability to overload bitwise operators, which enables customization of operations for user-defined classes. This is particularly useful if your class needs to perform operations with binary 1, or you need to implement custom logic for AND, OR, XOR, NOT, and SHIFT operators.
Operator overloading is achieved through the implementation of special methods within your class. These methods start and end with double underscores (__
) and correspond to specific bitwise operators. For example, a class can define the __and__
method to implement custom behavior for the bitwise AND operator (&).
Here’s a simple example of how to overload the bitwise AND operator for a custom class:
class BitCounter: def __init__(self, value): self.value = value def __and__(self, other): if isinstance(other, BitCounter): return BitCounter(self.value & other.value) return NotImplemented a = BitCounter(5) # Binary: 0101 b = BitCounter(3) # Binary: 0011 c = a & b # Binary: 0001 (1 in decimal)
In this example, we created a BitCounter
class that takes an integer value and overloads the bitwise AND operator. We first check if the other object is a BitCounter
instance; if so, we apply the operator to self.value
and other.value
. Otherwise, we return NotImplemented
.
To overload other bitwise operators, you would implement similarly named special methods within your class:
- Bitwise OR:
__or__
- Bitwise XOR:
__xor__
- Bitwise NOT:
__invert__
- Left SHIFT:
__lshift__
- Right SHIFT:
__rshift__
Frequently Asked Questions
How do I use bitwise AND operator in Python?
In Python, the bitwise AND operator is represented by an ampersand (&
). It operates on two numbers, comparing each bit of the first number to the corresponding bit of the second number. If both bits are 1, the corresponding result bit is set to 1; otherwise, it’s set to 0. Here’s an example:
a = 5 # Binary: 0101 b = 3 # Binary: 0011 result = a & b # Result: 0001 (Decimal: 1)
What is the Python syntax for bitwise OR operation?
The bitwise OR operator in Python is represented by the pipe symbol (|
). It compares each bit of the first number to the corresponding bit of the second number. If either bit is 1, the corresponding result bit is set to 1. Otherwise, it’s set to 0. Here’s an example:
a = 5 # Binary: 0101 b = 3 # Binary: 0011 result = a | b # Result: 0111 (Decimal: 7)
How can I perform bitwise XOR on a list in Python?
To apply a bitwise XOR operation on a list of numbers in Python, you can use the reduce()
function from the functools
module, along with the ^
operator. Here’s an example:
from functools import reduce nums = [1, 2, 3] result = reduce(lambda x, y: x ^ y, nums) # Result: 0
The reduce()
function successively applies the XOR operation to each pair of elements in the list.
How do I use bitwise NOT operator in Python?
The bitwise NOT operator in Python is represented by the tilde symbol (~
). It inverts each bit of the given number. If the bit is 1, it becomes 0; if it’s 0, it becomes 1. Keep in mind that Python uses two’s complement for integers, and the result will be a negative number. Here’s an example:
a = 5 # Binary: 0101 result = ~a # Result: -6 (Binary: 1010)
What are the differences between logical and bitwise operators in Python?
Logical operators (such as and
, or
, not
) in Python work with boolean values (True
and False
), while bitwise operators (like &
, |
, ^
, ~
, <<
, >>
) work with the individual bits of integers. Logical operators are mainly used for control flow and decision-making in the code, while bitwise operators are used for low-level numerical operations, bit manipulation, and binary data processing.
How can I perform shift operations in Python?
Python provides two bitwise shift operators: left shift <<
and right shift >>
. Left shift moves the bits of the number to the left, adding 0’s on the right, effectively multiplying the number by 2 raised to the power of the desired shifts. Right shift moves the bits to the right, reducing the number by dividing it by 2 raised to the power of the desired shifts. Here’s an example:
a = 5 # Binary: 0101 left_shift_result = a << 2 # Result: 20 (Binary: 10100) right_shift_result = a >> 1 # Result: 2 (Binary: 10)
If you want a more detailed introduction with videos for each bitwise operator (!), check out my blog tutorial here:
Recommended: Python Bitwise Operators [Full Guide + Videos]
Also, subscribe to our free email academy to help you stay on the right side of change by downloading our popular cheat sheets:
The post Bitwise Operators in Python (AND, OR, XOR, NOT, SHIFT) appeared first on Be on the Right Side of Change.
August 23, 2023 at 02:40PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
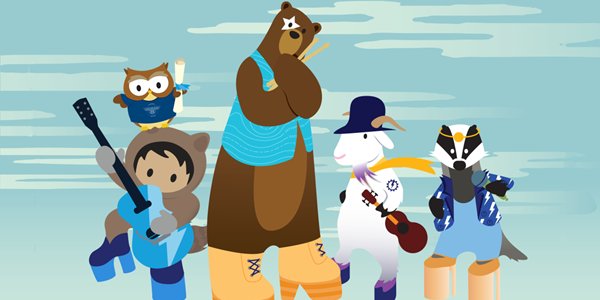
Post a Comment