Python Return NamedTuple From Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
In Python, you can use the collections.namedtuple()
function to create a tuple with named fields.
Here is an example of a function that returns a named tuple:
from collections import namedtuple # Define the named tuple type outside the function Person = namedtuple('Person', ['name', 'age', 'height']) def get_person_info(): # Create an instance of the named tuple person_info = Person(name='John', age=25, height=180) # Return the named tuple return person_info person = get_person_info() print(person.name) print(person.age) print(person.height)
In this example, the get_person_info()
function creates a Person
named tuple with name, age, and height as named fields. The function then creates an instance of this named tuple and returns it.
If the named tuple type definition is not required to be globally accessible, you can define it within the function as well.
Here is an example of this:
from collections import namedtuple def get_person_info(): # Define the named tuple type inside the function Person = namedtuple('Person', ['name', 'age', 'height']) # Create an instance of the named tuple person_info = Person(name='John', age=25, height=180) # Return the named tuple return person_info person = get_person_info() print(person.name) print(person.age) print(person.height)
In both of these examples, the get_person_info()
function returns a named tuple.
Understanding Python NamedTuples
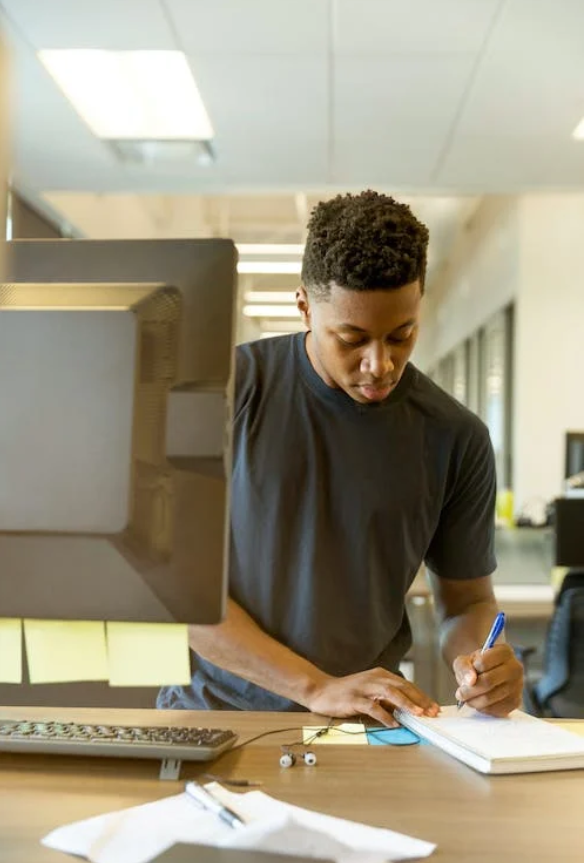
In Python, a namedtuple is a subclass of a tuple that brings additional readability and structure to the basic tuple data structure. Namedtuples are part of the collections
module and provide named fields for each element, making it similar to a dictionary with fixed keys but with the memory efficiency and immutability of tuples.
To create a namedtuple, you need to import the collections
module and use the collections.namedtuple()
function. This function takes two arguments: the name of the namedtuple and a list of field names.
Here’s an example:
import collections Person = collections.namedtuple('Person', ['name', 'age', 'height'])
Now you can create instances of the Person
namedtuple and access the fields using dot notation:
person1 = Person('Alice', 30, 170) print(person1.name) # Output: Alice print(person1.age) # Output: 30
Namedtuples are immutable, which means you cannot change the values once they are assigned. If you need to update any value, you must create a new namedtuple instance. However, you can convert a namedtuple to a dictionary using the _asdict()
method, modify the values, and then convert it back to a namedtuple.
person_dict = person1._asdict() person_dict['age'] = 31 person2 = Person(**person_dict)
Returning namedtuples from functions is a helpful way to provide multiple values without resorting to a simple tuple or a full-fledged class.
For example, consider a function that calculates and returns both the area and perimeter of a rectangle:
def rectangle_properties(length, width): area = length * width perimeter = 2 * (length + width) Rectangle = collections.namedtuple('Rectangle', ['area', 'perimeter']) return Rectangle(area, perimeter) properties = rectangle_properties(4, 2) print(properties.area) # Output: 8 print(properties.perimeter) # Output: 12
In this example, the function returns a namedtuple that clearly states what each value represents, making it more readable and easier to understand.
Creating NamedTuples

NamedTuples, part of the collections
module in Python, are an efficient and readable way to define simple classes that primarily store data. They are similar to tuples but provide names for each element, facilitating a better understanding of the code.
Let’s explore creating NamedTuples using functions, import statements, and naming conventions along with a few examples.
First, you need to import the namedtuple
function from the collections
module:
from collections import namedtuple
To create a namedtuple, you need to define its typename
(class name) and field_names
(attribute names). The namedtuple
function takes two arguments: the typename
as a string and field_names
as an iterable of strings or a single comma-delimited string.
For example, to create a namedtuple representing a point in a 3D space, with x, y, and z coordinates:
Point3D = namedtuple("Point3D", ["x", "y", "z"])
Alternatively, you can provide field names in a comma-delimited string:
Point3D = namedtuple("Point3D", "x, y, z")
With the namedtuple defined, you can now create instances of it, just like any other class:
point1 = Point3D(1, 2, 3) print(point1) # Output: Point3D(x=1, y=2, z=3)
Since the underlying namedtuple is a class, proper naming conventions dictate using a CamelCase class name. Field names should follow the standard lowercase_with_underscores
style.
Returning a namedtuple from a function is straightforward. For example, consider the following function to create a Point3D with random coordinates:
import random from collections import namedtuple Point3D = namedtuple("Point3D", "x, y, z") def random_point_3d(): x = random.randint(1, 10) y = random.randint(1, 10) z = random.randint(1, 10) return Point3D(x, y, z) point2 = random_point_3d() print(point2) # Output: For example, Point3D(x=4, y=7, z=2)
In this example, the random_point_3d
function generates random values for x, y, and z coordinates before creating a new instance of the Point3D namedtuple and returning it.
Working with NamedTuple Methods
In Python, collections.namedtuple
offers a convenient way to create a custom tuple-like object with named fields. NamedTuple provides several useful methods to work with your custom tuple. Let’s explore some of these methods including _fields
, _replace()
, _make()
, and _asdict()
.
_fields
The _fields
attribute lists the field names of a NamedTuple. This attribute can help you keep track of the fields and their order in your custom tuple.
Here’s an example:
from collections import namedtuple Person = namedtuple("Person", "name age") print(Person._fields) # Output: ('name', 'age')
_replace()
You might need to update a field in a NamedTuple, but since tuples are immutable, you can’t directly modify the values. The _replace()
method provides a workaround for this situation by creating a new NamedTuple with the specified field updated.
Here’s a code example:
person_instance = Person("Alice", 30) updated_person = person_instance._replace(age=31) print(updated_person) # Output: Person(name='Alice', age=31)
_make()
The _make()
method allows you to create a new NamedTuple instance from an iterable, such as a list or tuple. This is particularly useful when you want to construct NamedTuples from data stored in other data structures.
Here’s an example:
data = ("Bob", 25) person_from_data = Person._make(data) print(person_from_data) # Output: Person(name='Bob', age=25)
_asdict()
Sometimes, you may want to convert your NamedTuple into a dictionary for easier manipulation and better compatibility with other Python data structures. The _asdict()
method returns an OrderedDict with field names as keys and corresponding values as dictionary values.
Here’s how you can use it:
person_instance = Person("Charlie", 22) person_dict = person_instance._asdict() print(person_dict) # Output: OrderedDict([('name', 'Charlie'), ('age', 22)])
These NamedTuple methods offer concise and clear ways to work with your custom tuple-like object, making your Python code more readable and understandable. Remember to use the appropriate method for each task when working with NamedTuples.
Returning NamedTuples from Functions

Returning namedtuples from functions in Python is a helpful technique for clean and readable code. Namedtuples can be considered as an extension of tuples, where values have assigned names, making it easier to understand and access them. They are immutable and memory efficient, as well as belonging to the collections
module.
To return a namedtuple from a function, first import the namedtuple factory function from the collections
module:
from collections import namedtuple
Then, define your namedtuple structure with the desired field names:
Coordinates = namedtuple("Coordinates", ["x", "y", "z"])
Now, you can create a function that takes in necessary arguments and returns an instance of your namedtuple:
def get_coordinates(x, y, z): return Coordinates(x, y, z)
When calling the function, it will return a namedtuple object with the given values:
coordinates = get_coordinates(4, 2, 9) print(coordinates)
The output will be:
Coordinates(x=4, y=2, z=9)
Accessing namedtuple fields can be done using the dot notation, which is more readable compared to accessing tuple’s elements using index positions:
print(coordinates.x) print(coordinates.y) print(coordinates.z)
The output will be:
4 2 9
Frequently Asked Questions

How to create a namedtuple in a function?
To create a namedtuple in a function, you need to import the namedtuple
function from the collections
module. Then, you can define a namedtuple class and instantiate objects of that class. Here’s an example:
from collections import namedtuple def create_person(): Person = namedtuple('Person', 'name age') person = Person(name='John', age=30) return person john = create_person() print(john.name, john.age)
How to set default values for namedtuples?
To set default values for a namedtuple, you can use the _make
method in combination with a default tuple. Here’s an example:
from collections import namedtuple Person = namedtuple('Person', ['name', 'age']) Person.__new__.__defaults__ = ('No Name', 0) # Set the default values person_with_defaults = Person() print(person_with_defaults)
How to convert a namedtuple to a dictionary?
To convert a namedtuple to a dictionary, simply use the _asdict
method:
from collections import namedtuple Person = namedtuple('Person', 'name age') person = Person(name='John', age=30) person_dict = person._asdict() print(person_dict)
What are the differences between NamedTuple and namedtuple?
NamedTuple
is a class available in the typing
module, whereas namedtuple
is a function available in the collections
module. Although both are used for creating named tuple classes, NamedTuple
provides improved syntax and supports type annotations. Here’s an example using NamedTuple
:
from typing import NamedTuple class Person(NamedTuple): name: str age: int person = Person(name='John', age=30) print(person)
How to create a list of namedtuples?
To create a list of namedtuples, simply instantiate multiple namedtuple objects and append them to a list:
from collections import namedtuple Person = namedtuple('Person', 'name age') persons_data = [('John', 30), ('Jane', 28), ('Mike', 25)] people = [Person(name, age) for name, age in persons_data] print(people)
What are the advantages of using a namedtuple over a dictionary?
Namedtuples come with some advantages over dictionaries, such as:
- Better memory efficiency as namedtuples store data as tuples, which consume less memory than dictionaries.
- Attribute-based access, making the code more readable and clean.
- Immutability, which ensures that the data remains constant and cannot be modified accidentally.
However, dictionaries can be more suitable for specific use cases where you need dynamic keys, mutable data, or non-unique field names.
Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
If your answer is YES!, consider becoming a Python freelance developer! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
July 31, 2023 at 09:46PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
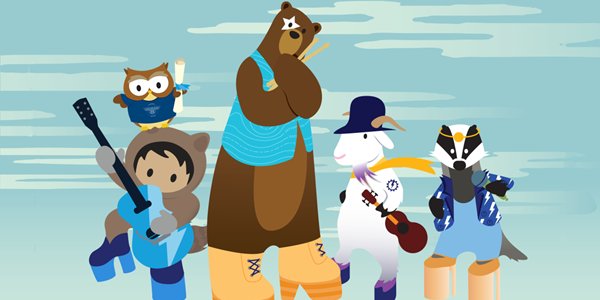
Post a Comment