OpenAI.Error.AuthenticationError Empty Message : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
The openai.error.authenticationerror <empty message>
is an issue that some developers face while working with OpenAI’s API. This error is often an indication of invalid or expired API keys, or even a problem with the underlying platform or model. The error can also appear if you published your API key publicly, e.g., on GitHub.
openai.error.AuthenticationError: <empty message>
To debug the authentication error, you should first verify your API keys and ensure they are entered correctly. Additionally, you should pay attention to the authentication process and maintain API key security by avoiding potential security breaches. In some cases, simply generating another token or a new API key will fix the issue!
Warning: Do not publish your API key publicly (e.g., on GitHub)! According to OpenAI, this can mean that your API key will get deleted automatically causing the issue:
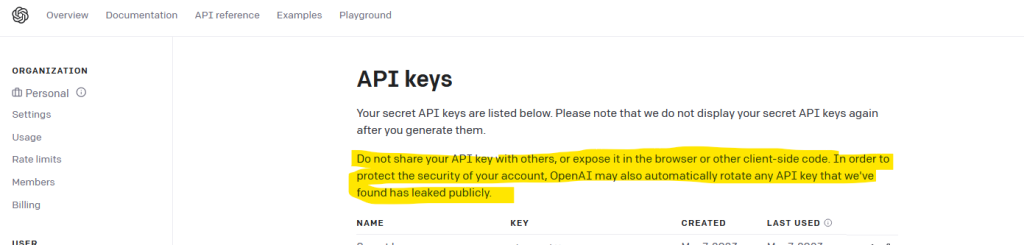
Do not share your API key with others, or expose it in the browser or other client-side code. In order to protect the security of your account, OpenAI may also automatically rotate any API key that we've found has leaked publicly.
You may also want to try the following:
Step 1: Open the .env
file in your project root directory and add your OPENAI_API_KEY=sk-...
Step 2: Use the following Python code to import it:
config = dotenv_values('.env') openai.api_key = config['OPENAI_API_KEY'] os.environ['OPENAI_API_KEY'] = config['OPENAI_API_KEY']
This may already fix it.
Understanding Error: AuthenticationError Empty Message
Common Causes
The openai.error.AuthenticationError
with an empty message can occur for several reasons. Some of the common causes include:
- Invalid or expired API key:
Ensure you are using a valid API key and that it has not expired.
- Typo or formatting error: Double-check your API key or token for any typos or formatting issues.
- Revoked API key:
If your API key has been revoked, you may need to request a new one.
For more details on how to fix authentication errors, refer to the OpenAI Help Center recommendation.
Impact on OpenAI API Usage
Facing an AuthenticationError
while using the OpenAI API can have some consequences, such as:
- Inability to access the API:
You won’t be able to access or use the API without proper authentication.
- Delay in AI processes:
If you were running any processes that depend on the OpenAI API, they may be delayed or halted until the issue is resolved.
- Retry or debugging:
You might need to spend time troubleshooting and resolving the error before being able to use the API again.
Remember to keep your API key safe and secure, as it is crucial for successfully accessing the API and using AI functionalities.
API Key Management
Proper Storage
Proper storage of your OpenAI API key is crucial for maintaining security and preventing unauthorized access to your OpenAI account. It’s important to store your API key in a safe location, such as an environment variable, and avoid hard-coding it within your application code.
Here are some guidelines for storing your OpenAI API key:
- Save your API key in an environment variable. This makes it accessible to your application code without directly including it in the source code.
- Be cautious when sharing your code. Even if you remove the API key from the source code, make sure to double-check your version control history and configuration files.
- Regularly review and update access controls to your API key, removing access for users who no longer require it.
Regeneration of API Key
There may be situations where you need to regenerate your OpenAI API key, such as when you suspect unauthorized access or accidentally expose the key. Regenerating the API key ensures that only authorized users can access your OpenAI services.

To regenerate your API key:
- Visit your OpenAI API Key Dashboard (you’ll need to be logged in to your OpenAI account).
- In the “API Keys” section, locate your existing key and click the “Create new secret key” button.
- Update your application code and any settings that reference the old API key with the newly generated key.
- Verify that your application continues to function correctly after the change.
Remember, regularly reviewing your API key usage and following best practices for key management helps maintain the security of your OpenAI services. Be vigilant and proactive in managing your API keys to ensure a secure and reliable integration with OpenAI.
OpenAI Authentication Process
Code Implementation
The OpenAI API requires an authentication process to secure its services and ensure the correct usage of resources. In Python, the authentication can be done by integrating a valid OpenAI API key in the header of your request or using an SDK (e.g., openai-python
). Make sure to import the required libraries and set the proper credentials before making API calls:
import openai openai.api_key = "your-openai-api-key" # Example API call response = openai.Model.list()
Remember, do not hardcode the API key directly into the code. Instead, store it as an environment variable or use a configuration file for better security .
Possible Issues
In case you encounter the openai.error.AuthenticationError: <empty message>
error, it could mean your API key is invalid, expired, or revoked. Here are some troubleshooting steps you can take:
- Ensure you’re using the correct API key
. Double-check the key and make sure it’s properly integrated into your code.
- Examine the code implementation for formatting errors or typos
. This might cause an incorrect API key to be passed in your requests.
- Verify that your API key has not expired or been revoked. Access your OpenAI account and check the status of your API key. If needed, generate a new one.
- If you still face issues, try reproducing the error using the example code provided in the documentation. For instance, you can use the
openai.Model.list()
method as a test case.
Debugging Authentication Errors
API Key Validation
When facing an openai.error.AuthenticationError
with an empty message, it’s essential to validate your API key . Ensure that you have the correct API key, and it’s not expired or invalidated. Check your OpenAPI dashboard for any potential issues with the credentials.
Python Code Troubleshooting
If the API key seems valid but the error persists, you may need to check the Python code for configurations or import issues .
- Make sure you have installed the necessary libraries, such as
openai
. - Double-check your environment variables, especially for
os
: verify if the API key is correctly stored and accessible. - Inspect your
engine_api_resource
for any discrepancies that could hinder authentication. - Ensure proper usage of the
import
statements in your code for pertinent modules.
Remember, the key to debugging authentication errors is staying confident, knowledgeable, and focused on the issue at hand! Remember to maintain a clear and neutral approach in your debugging attempts.
Precautions in Different OS Platforms
In this section, we will discuss some precautions to take when dealing with the openai.error.authenticationerror empty message
issue on different OS platforms, focusing on Windows and Windows 11.
Windows System Settings
When working with the OpenAI API on a Windows system, it’s important to ensure that your API key is correctly configured. One way to do this is by storing your API key in a separate secrets.json
file, which can help you avoid hardcoding it into your script.
- Make sure that your
secrets.json
file is readable and not corrupted. - Ensure that your system’s environment variables are correctly set up to access the API key.
- Double-check that you have the necessary permissions for accessing your API key.
Dealing with Windows 11
Windows 11 brings several new features, and you may experience some issues when using it with the OpenAI API. Here are some troubleshooting steps specific to Windows 11:
- Check system requirements: Make sure your system meets the minimum requirements to run Windows 11, as it could impact the performance of your app.
- Update Python and Libraries: Always use the latest compatible versions of Python and required libraries like requests or GitPython for optimal performance.
- Review Operating System Changes: Windows 11 has various enhancements and modifications that might affect your code. Ensure your code takes these into account.
- Inspect Firewall Settings: Adjust Windows 11 security settings as needed to allow the proper communication between your app and OpenAI API servers.
Frequently Asked Questions
How can I troubleshoot the AuthenticationError in OpenAI?
When encountering an AuthenticationError, first make sure your API key or token is correct and active. You may need to generate a new key from the API Key dashboard, ensure there are no extra spaces or characters, or use a different key if you have multiple ones. If the problem persists, check out the OpenAI Help Center for more troubleshooting steps.
What causes OpenAI AuthenticationError?
AuthenticationError in OpenAI is often caused by incorrect or inactive API keys. Other possible causes include extra spaces or characters in the key, using an expired key, or an issue on the server-side.
How do I resolve an empty message error in OpenAI?
To resolve an empty message error in OpenAI, first ensure your API key is valid and properly formatted. You can also try using .rstrip()
to remove any potential white spaces or newline characters at the end of the prompt, as mentioned in the OpenAI Developer Forum.
Are there common mistakes with OpenAI API keys?
Yes, common mistakes with OpenAI API keys include having extra spaces or characters, using an expired or inactive key, or accidentally including two keys in your configuration file as described in this GitHub issue.
What are some tips for proper OpenAI key management?
Proper OpenAI key management includes:
- Regularly checking your API Key dashboard to ensure your key is active
- Avoiding sharing your key publicly or with unauthorized users
- Rotating your API keys periodically to minimize security risks
- Ensuring only one API key is used in your configuration file and removing unused keys
How can I avoid the error code 500 in OpenAI?
To avoid error code 500 in OpenAI, review your code to ensure proper syntax and formatting. Check if you’re making too many requests within a short period, which may result in rate limiting. Additionally, monitor the OpenAI API status to ensure there are no ongoing server-side issues. When error code 500 occurs, it’s essential to debug your code and determine the root cause to prevent it from reoccurring in the future.
July 06, 2023 at 05:20PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
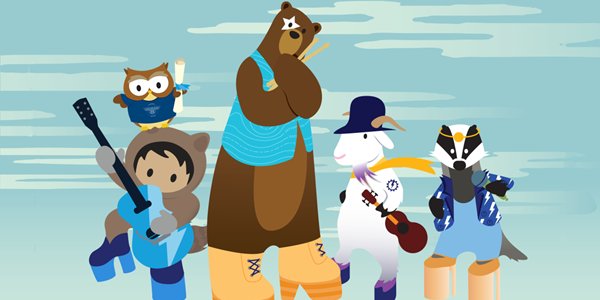
Post a Comment