4 Easy Ways to Download a Video in Python : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Method 1: PyTube
To download a YouTube video using PyTube, install the library with pip install pytube
, import the YouTube
object from pytube, instantiate the YouTube object with your video URL, get the highest resolution stream of the video with youtube.streams.get_highest_resolution()
, and download the video to your desired location using video.download('/path/to/download')
. Ensure to replace '/path/to/download'
with your preferred download directory.

First, you have to install the library. You can do this by running pip install pytube
in your command line.
Recommended: How to Install a Python Library (5 Steps)
Once you’ve done that, you can use the following Python script to download a video:
from pytube import YouTube def download_video_from_youtube(link, path): yt = YouTube(link) video = yt.streams.get_highest_resolution() # download the video video.download(path) # example usage: download_video_from_youtube('https://www.youtube.com/watch?v=dQw4w9WgXcQ', '/path/to/download/directory')
This script takes a YouTube video link and a download path as input. It then uses pytube
to get the video from the link, and downloads the highest resolution stream of that video to the provided download path.
Please note:
Legality Alert! It needs to be said. The legality of downloading videos from YouTube (or other video services) depends on the jurisdiction and the specific terms of service. In many cases, it may be against the terms of service to download and redistribute videos without the explicit permission of the content owner. This script is provided for educational purposes only, and you should not use it to download or distribute videos without permission.
Method 2: URLLib Request
The urllib.request.urlretrieve(video_url, filename)
function can download resources from URLs, and that includes videos. Pass the URL of the resource you want to download as the first argument and the local filename you want to save the downloaded resource to as the second argument. The function will then download the resource at the given URL and save it to the specified filename.

However, it might not always work as expected with video content from platforms like YouTube due to the way such platforms stream and protect their video content.
import urllib.request url_link = 'http://example.com/video.mp4' # replace with your actual video url filename = 'video_name.mp4' urllib.request.urlretrieve(url_link, filename)
Note that urlretrieve
won’t work with all URLs, especially if the file is protected or requires some kind of session or cookie to access or if the server checks the User-Agent and doesn’t like Python’s default User-Agent.
YouTube, for example, uses JavaScript and dynamic page elements to protect its videos from being downloaded, which urlretrieve
can’t handle because it doesn’t execute JavaScript or handle dynamic page elements.
That’s why libraries like youtube_dl
or pytube
are typically used to download videos from YouTube – they can handle the complexity of YouTube’s video serving system.
Method 3: Youtube_DL Library
To download a YouTube video with youtube_dl
in Python, install the library using pip install youtube_dl
and import youtube_dl
in your script using the YoutubeDL
object’s download
method, passing in a list containing your video’s URL. By default, the video will be saved in your current working directory.

Then you can use the following Python code:
import youtube_dl def download_video(url): ydl_opts = {} with youtube_dl.YoutubeDL(ydl_opts) as ydl: ydl.download([url]) # replace 'url' with your video URL download_video('url')
Here’s what this code does:
ydl_opts = {}
is a dictionary of configuration options foryoutube_dl
. In this case, it’s empty, which means we’re using the default options.youtube_dl.YoutubeDL(ydl_opts)
creates aYoutubeDL
object with the given options.ydl.download([url])
downloads the video at the provided URL.
The video will be downloaded to your current working directory. If you want to save it to a specific directory, you can add the 'outtmpl'
key to your ydl_opts
dictionary, like this: 'outtmpl': '/path/to/download/%(title)s-%(id)s.%(ext)s'
.
Again: Please respect YouTube’s terms of service and the rights of content creators when downloading videos.
youtube_dl
is a widely used, reliable library for downloading videos from YouTube and other video platforms. However, because these platforms frequently change their backends, youtube_dl
may occasionally stop working until it is updated by its maintainers.
Method 3: Requests Library
To download a video from a direct URL using the requests
library in Python, first install requests
with pip install requests
. Then, in your script, import requests
, call requests.get(url)
to get the video content, and write it to a file using the write()
function of a file object, opened in binary write mode ('wb'
). Replace 'url'
with your video’s URL and 'filename'
with your preferred file name.
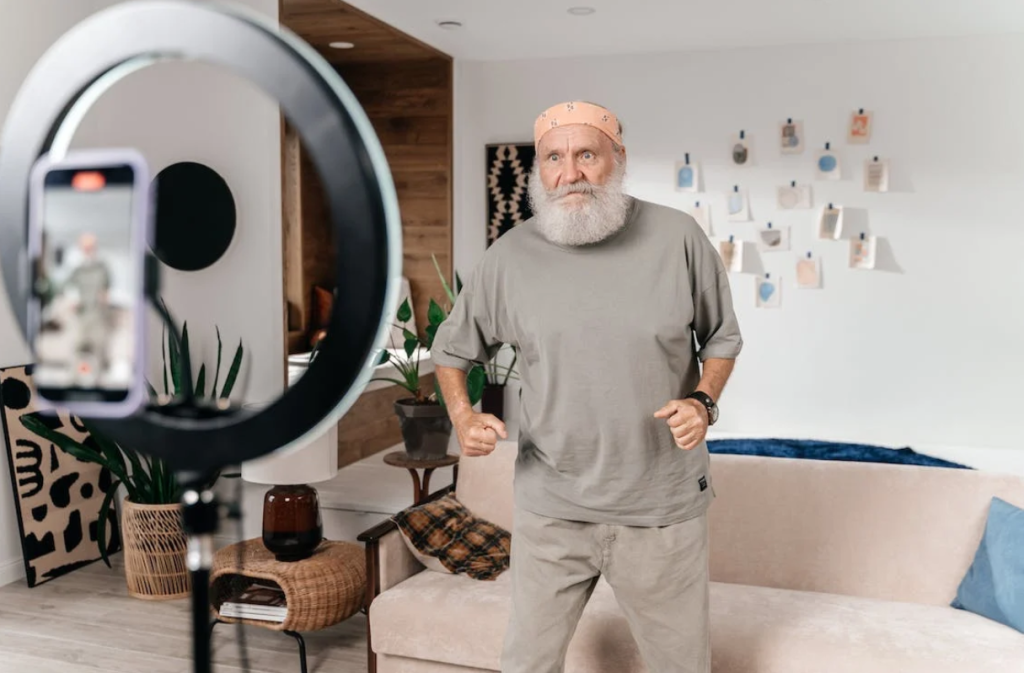
Here’s an example:
import requests def download_video(url, filename): r = requests.get(url) with open(filename, 'wb') as f: f.write(r.content) # replace 'url' and 'filename' download_video('http://example.com/video.mp4', 'video.mp4')
This method doesn’t work for YouTube videos due to the platform’s protection mechanisms. Always respect copyright laws and terms of service.
Method 4: URLLib2
To download a video from a URL (e.g., on Coursera) using Python’s urllib2
library and handle Basic HTTP Authentication, use urlopen()
to retrieve the video content, which is then written into a file. If the server requires authentication, the HTTPPasswordMgrWithDefaultRealm
and HTTPBasicAuthHandler
classes are used to create an opener that includes the username and password.

Here’s an example:
import urllib2 # video link and filename dwn_link = 'https://class.coursera.org/textanalytics-001/lecture/download.mp4?lecture_id=73' file_name = 'trial_video.mp4' # download and save the video rsp = urllib2.urlopen(dwn_link) with open(file_name,'wb') as f: f.write(rsp.read()) # if Basic HTTP Authentication is required password_mgr = urllib2.HTTPPasswordMgrWithDefaultRealm() top_level_url = "http://class.coursera.org/" password_mgr.add_password(None, top_level_url, username, password) handler = urllib2.HTTPBasicAuthHandler(password_mgr) # create "opener" (OpenerDirector instance) opener = urllib2.build_opener(handler) # use the opener to fetch a URL opener.open(dwn_link)
Please note that as of Python 3, urllib2
has been split across several modules in urllib
package, so the above example won’t work directly in Python 3. Also, respect copyright laws and terms of service when downloading videos.
Recommended: Create Your Own YouTube Video Downloader
July 07, 2023 at 09:00PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
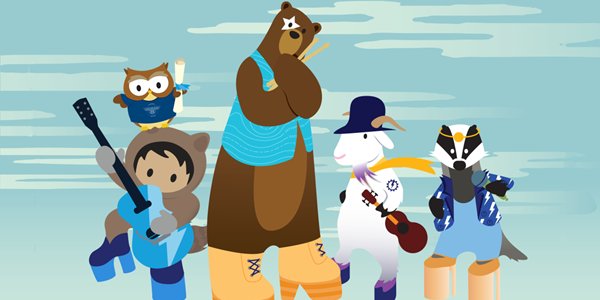
Post a Comment