11 Ways to Create a List of Odd Numbers in Python : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Method 1: Using List Comprehension
To create a list of odd numbers in Python, you can use the list comprehension [x for x in range(10) if x%2==1]
that iterates over all values x
between 0 and 10 (exclusive) and uses the modulo operator x%2
to check if x
is divisible by 2 with remainder, i.e., x%2==1
.
Here’s a minimal example:
odd_numbers = [x for x in range(10) if x%2==1] print(odd_numbers) # [1, 3, 5, 7, 9]
Recommended: List Comprehension in Python — A Helpful Illustrated Guide
Method 2: Using a For-loop and Append Method
This method involves a standard for
-loop and the list’s append()
method. We iterate from 0 to 10, multiply each number by 2 and add 1 to get odd numbers, and append these to the list.
Here’s an example:
odd_numbers = [] for i in range(0, 11): odd_numbers.append(i * 2 + 1) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: Python List
append()
Method
Method 3: Using a For-loop and List’s += Operator
This method is similar to the previous one, but instead of using the append()
method, we use the list’s +=
operator to add new elements.
Here’s an example:
odd_numbers = [] for i in range(0, 11): odd_numbers += [i * 2 + 1] # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: Python In-Place Addition Operator
Method 4: Using a While-loop
This method uses a while
-loop to generate the list of odd numbers. We start with i=0
and increment i
in each iteration until it exceeds 10.
Here’s an example:
odd_numbers = [] i = 0 while i <= 10: odd_numbers.append(i * 2 + 1) i += 1 # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: Python Loops
Method 5: Using List Comprehension (
One-Liner)
List comprehension is a concise way to create lists in Python. It can be used to generate a list of odd numbers by multiplying each number in a range by 2 and adding 1.
Here’s an example:
odd_numbers = [i * 2 + 1 for i in range(0, 11)] # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: List Comprehension in Python — A Helpful Illustrated Guide
Method 6: Using the Map Function (
One-Liner)
The map()
function applies a given function to each item of an iterable (e.g., list) and returns a list of the results. We can use it with a lambda function to generate odd numbers.
Here’s an example:
odd_numbers = list(map(lambda x: x * 2 + 1, range(0, 11))) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: Python
map()
— Finally Mastering the Python Map Function [+Video]
Method 7: Using the Filter Function (
One-Liner)
The filter()
function constructs a list from elements of an iterable for which a function returns True
. Here, we use it with a lambda function to filter odd numbers from a range.
Here’s an example:
odd_numbers = list(filter(lambda x: x % 2 == 1, range(0, 21))) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
Recommended: Python
filter()
Method 8: Using the Range Function with a Step Argument (
One-Liner)
The range()
function can accept a step argument to skip numbers. By starting at 2 and stepping by 2, we can generate a list of odd numbers.
Here’s an example:
odd_numbers = list(range(1, 21, 2)) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
Recommended: Python
range()
Function — A Helpful Illustrated Guide
Method 9: Using the Itertools Module (
One-Liner)
The itertools
module is part of the Python standard library and contains many functions that are useful for efficient iteration. Here, we use itertools.count
to create an infinite sequence of odd numbers, and itertools.islice
to take the first 10.
Here’s an example:
import itertools odd_numbers = list(itertools.islice(itertools.count(1, 2), 10)) print(odd_numbers) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
This code uses the itertools
module in Python to generate a list of the first 10 odd numbers.
Here’s a breakdown of how it works:
itertools.count(1, 2)
: This function generates an infinite sequence starting at 1 and incrementing by 2 each time. This means it will generate the infinite sequence of odd numbers: 1, 3, 5, 7, 9, …itertools.islice(itertools.count(1, 2), 10)
: This function takes two arguments: an iterable and a stop value. It returns a generator that yields items from the iterable, but stops after the specified number of items. In this case, it generates the first 10 odd numbers.list(itertools.islice(itertools.count(1, 2), 10))
: This code converts the generator object returned byitertools.islice()
into a list. The list is assigned to the variableodd_numbers
.print(odd_numbers)
: This line prints the list of the first 10 odd numbers.
The output is: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
, which are the first 10 odd numbers.
Recommended: Iterators, Iterables and Itertools
Method 10: Using Numpy Array (
One-Liner)
Numpy is a powerful library for numerical computations in Python. We can use the numpy function arange()
to generate a sequence of odd numbers.
Here’s an example:
import numpy as np odd_numbers = list(np.arange(1, 21, 2)) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
Recommended: NumPy
arange()
: A Simple Illustrated Guide
Method 11: Using Generator Expression (
One-Liner)
A generator expression is a high-performance, memory–efficient generalization of list comprehensions and generators. In this method, data is not stored in memory all at once but is generated on the fly.
Here’s an example:
odd_numbers = list(i * 2 + 1 for i in range(0, 11)) # Output: [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
Recommended: Python Generator Expressions
Also, you may want to check out our free Finxter email academy — join 150k coders worldwide improving their skills together!
July 16, 2023 at 04:05PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
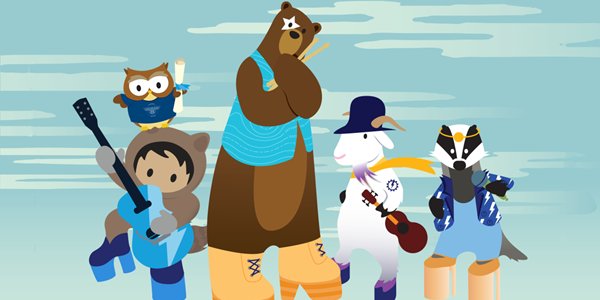
Post a Comment