Creating a Sitemap with Django or How I Created a Blog Web App : Jonathan Okah
by: Jonathan Okah
blow post content copied from Be on the Right Side of Change
click here to view original post
In this series, we will add a sitemap to our blog application. If this is your first time attempting this project, please start from the first part until you get to the previous series to fully understand what we’ll be doing in this project.
What is a sitemap? It is a file that contains the website’s structure, showing among other things, the pages and posts on a website.
If you are a blogger, you understand the importance of search engine optimization and its role in ranking your page in search engines. If your website is not visible in Google or other search engines, few people will know about the existence of your website. This will affect your ability to generate revenue through ads, affiliate marketing or other monetization strategies.
For your website to be visible, search engines like Google send spiders to crawl your website. A sitemap helps these crawlers to find and index your website to identify the most important pages. As you all know, Google is always on the lookout for fresh content.
When a sitemap helped search engines like Google identify new pages on your website and Google saw how frequently you update your website with fresh content, your website will become more visible to internet users. Can you see how important having a sitemap in your blog app is to SEO?
Steps to create a sitemap in the Django blog application
Django makes it possible for developers to generate sitemaps dynamically using its sites and sitemap framework. We will follow five simple steps to create a sitemap in our blog application.
Step 1: Add these to your INSTALLED_APPS
section
INSTALLED_APPS = [ … 'django.contrib.sites', 'django.contrib.sitemaps', ] SITE_ID = 1
We add the sites framework and the sitemaps app to our project’s settings. The SITE_ID
defines how many websites we have in this Django project.
Step 2: Update the database
We do this by running migration on the terminal
python3 manage.py makemigrations python3 manage.py migrate

Go to the admin interface to see the Sites
model added to the database. The domain given for the sitemap is example.com
. We will change this soon.
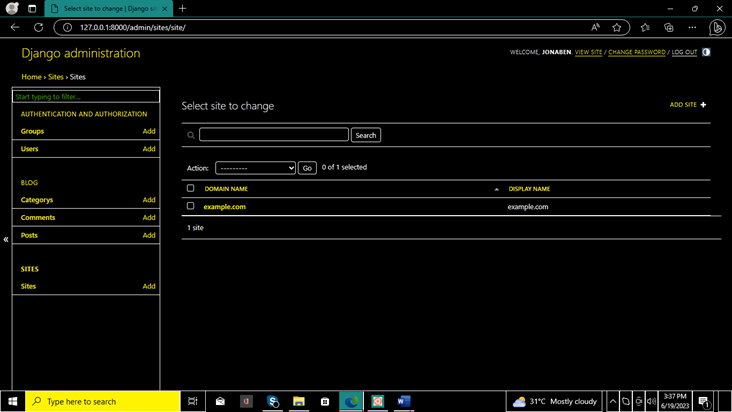
Step 3: Create a Django sitemap.py
file
This will be done in the app-level directory. Creating a separate sitemap file as opposed to using GenericSitemap
class in the urls.py gives us more control and access to its available methods some of which will be used in this project.
from django.contrib.sitemaps import Sitemap from .models import Post class PostSitemap(Sitemap): changefreq = 'weekly' priority = 0.8 protocol = 'http' def items(self): return Post.objects.all() def lastmod(self, obj): return obj.last_modified def location(self, obj): return f'/blog/{obj.slug}'
We create a sitemap class PostSitemap
that extends the Sitemap
class imported at the top. The attributes added are explained as follows:
changefreq
is self-explanatory. A serious blogger should be updating his blog at least twice every week. Hence, we set weekly. Other options include hourly, daily, monthly and yearly.priority
ranks the pages in order of importance to other posts on your website. It ranges from 0 to 1.protocol
refers to the website protocol, which can be eitherhttp
orhttps
. Since our blog is still hosted on the local server, we usehttp
.
The methods are explained as follows:
- The
items()
method returns all the model objects we want to be included in the sitemap. - The
lastmod()
method returns the date a post is created or modified. - The
location()
method returns the location of the URL. We choose slug since it is defined in thePost
model and sets to populate itself based on the post title. Thus, for any post, the URL will now behttp://127.0.0.1:8000. /blog/<title_of_post>
Step 4: Add the sitemap URL
… from .sitemaps import PostSitemap from django.contrib.sitemaps.views import sitemap sitemaps = { 'blog': PostSitemap } urlpatterns = [ … path('sitemap.xml', sitemap, {'sitemaps': sitemaps}, name='django.contrib.sitemaps.views.sitemap'), ]
Step 5: Change the Sites
domain name
Go to the admin interface (see screenshot below), and click on the example.com
.

Change the fields to localhost since we are using the local server. When we deploy the app to a production server, we will use our domain name.

We can now check to see that the sitemap is displayed by starting the local server and navigating to http://127.0.0.1:8000/sitemap.xml

Conclusion
Congratulations on adding a sitemap to your Django blog application. You can now see that a sitemap is nothing but just an XML file but does a lot of work in promoting your website and making it visible in search engines.
Note that a sitemap is not the only requirement for your page to rank in search engines like Google. There are many more things to do which of course, you can find on the Internet. But a sitemap has its role and should not be neglected.
The simple blog website we created is becoming more sophisticated. The source code on my GitHub page has been adjusted. Check it out. In the next series of this project tutorial, we will come back to sitemap once again. Notice we didn’t create sitemap for static pages like About Us and Contact pages. This is because those pages are not yet in our blog application.
Creating those pages in this series will increase your reading time and you may become exhausted especially if you are just starting web development with Django. Take a break and relax. In the next series, we will create a Contact and About Us page. Then, we add them to sitemap for static pages. Stay tuned!
June 25, 2023 at 07:54PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Jonathan Okah
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
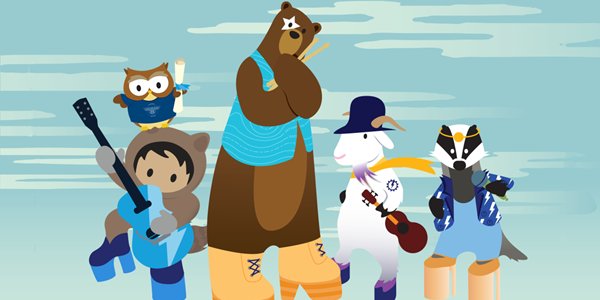
Post a Comment