Checking Julia Version & Installation Quickstart : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Julia is a high-level, high-performance programming language for technical computing, with an easy-to-understand syntax, widely used for various applications, including data science, machine learning, and scientific research.
There are multiple ways to check the version, such as using built-in command-line options or referencing special variables within the programming environment.
Julia Version Overview
To check the version of Julia running on your system, open a terminal or command prompt and enter the following command:
Check Julia Version with this Command:![]()
julia -version
This will display information about the current Julia installation, including the version number. For example, if you see julia version 1.7.1
, it means you are using version 1.7.1 of the Julia programming language.
Check Julia Version in your program:![]()
println(VERSION)
Another way to check the Julia version is by accessing the VERSION
variable within the Julia REPL (Read-Eval-Print Loop). Simply type VERSION
and press enter, and you will see the version number displayed as a VersionNumber
object. This usually includes the major, minor, and patch version details.

TLDR. By using the julia -version
command or the VERSION
variable within the Julia REPL, you can quickly and easily identify your Julia installation’s version and then consult the official documentation for any relevant updates or improvements.
Installation and Setup
In this section, we will guide you through the process of installing and setting up Julia for your specific operating system.
Windows Setup
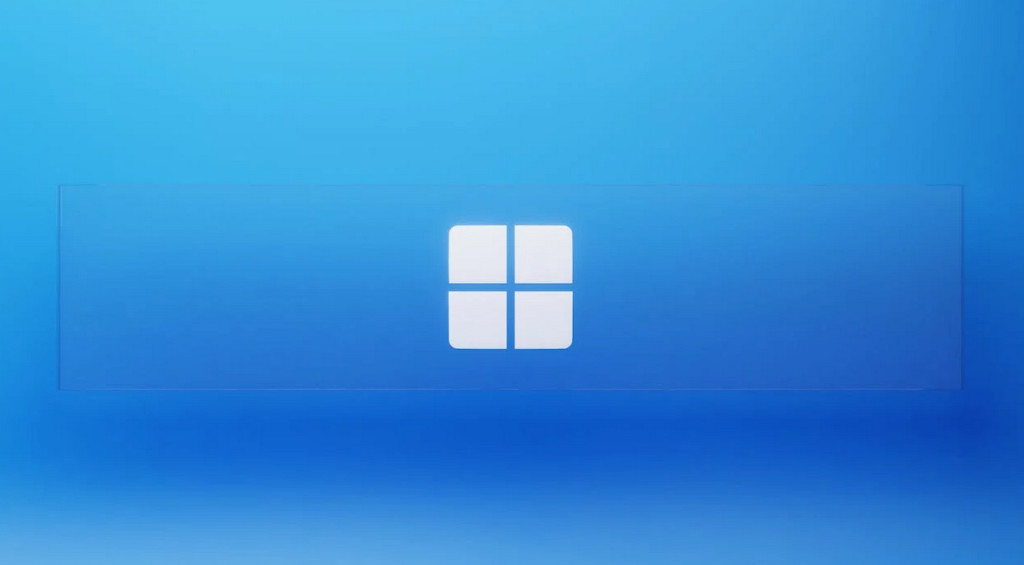
Julia is available for Windows 7 and later, supporting both 32-bit and 64-bit versions. To install Julia, follow these steps:
- Download the Windows Julia installer from the official downloads page.
- Run the installer and follow the on-screen instructions to complete the installation.
- After installation, we highly recommend using a modern terminal, such as Windows Terminal from the Microsoft Store.
- Launch the terminal and run the
julia
command. This should open the Julia REPL, where you can start coding.
For those who prefer working with an IDE, the Julia extension for Visual Studio Code is a popular choice.
Mac and Linux Setup
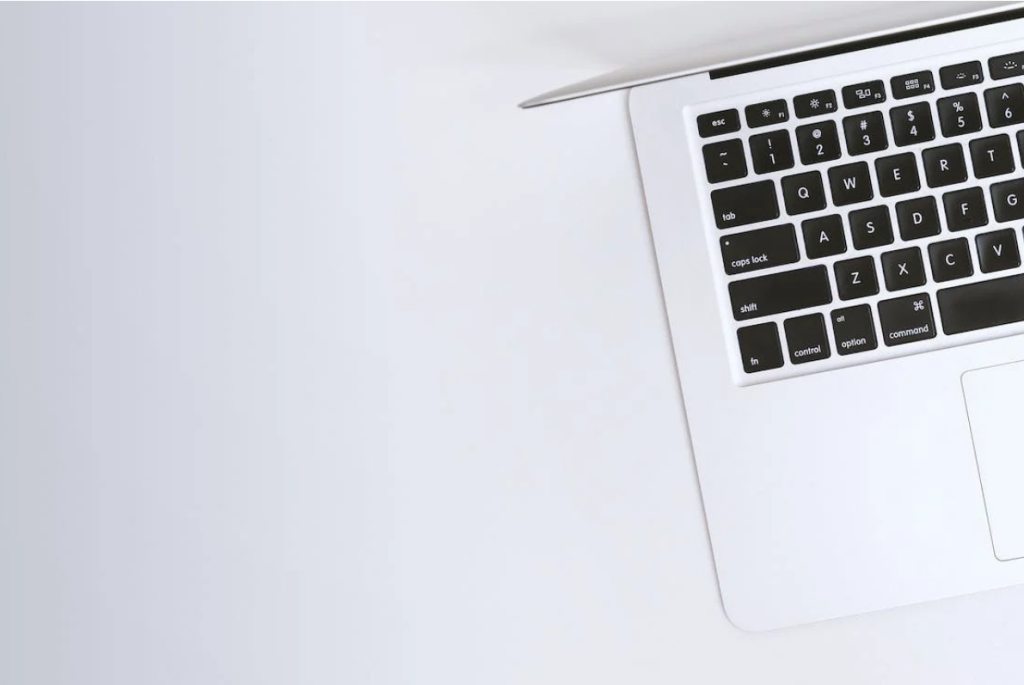
For Mac and Linux users, the installation process is slightly different. Here’s the step-by-step guide for both platforms:
- Visit the Julia downloads page and select the appropriate installer for your operating system.
- For Mac, open the downloaded
.dmg
file and drag the Julia application into the Applications folder. For Linux, extract the downloaded.tar.gz
file to your desired location. - Add the Julia executable to your PATH variable:
- Mac: Add the following line to your
~/.bash_profile
or~/.zshrc
file (depending on your shell):export PATH="/Applications/Julia-X.Y.Z.app/Contents/Resources/julia/bin:$PATH"
ReplaceX.Y.Z
with your installed Julia version. - Linux: Add the following line to your
~/.bashrc
or~/.zshrc
file (depending on your shell):export PATH="/path/to/Julia-X.Y.Z/bin:$PATH"
ReplaceX.Y.Z
with your installed Julia version and/path/to
with the appropriate installation path.
- Mac: Add the following line to your
- Open a new terminal window and run the
julia
command to start the Julia REPL.
The Julia extension for Visual Studio Code is also available for Mac and Linux users, providing a comprehensive IDE experience.
Running Julia Code
Running Julia code can be done in several ways, depending on your environment and preferred workflow. In this section, we will cover three common methods for executing Julia code: REPL, IDEs and Text Editors, and Command Line Execution.
REPL
REPL, or Read-Evaluate-Print Loop, is an interactive programming environment that comes built-in with Julia. It allows you to test, experiment, and run code on-the-fly. To use the REPL:
- Open a terminal or command prompt.
- Type
julia
and hit enter. - Once the Julia REPL is loaded, you can enter your code directly and see the results immediately.
The REPL is a great place to practice writing code, exploring new packages, and testing out functions without the overhead of a full project setup.
IDEs and Text Editors
For more complex projects or multi-file code, using an integrated development environment (IDE) or a text editor with Julia support can help streamline your workflow. Visual Studio Code (VS Code) is a popular option that provides a rich set of features for Julia developers, such as syntax highlighting, autocompletion, and debugging capabilities. To use Julia with VS Code, you need to install the Julia extension.
In VS Code, you can execute portions of your code or entire files using the following commands:
Julia: Execute Code in REPL
: Runs selected code in the REPL.Julia: Execute Code Cell in REPL
: Runs a code cell (marked by# %%
comments) in the REPL.Julia: Execute File in REPL
: Runs the entire file in the REPL.Julia: Run File in New Process
: Runs the file in a new process instead of the REPL.
These commands allow you to test your code as you write it, making it easier to detect errors and optimize performance.
Command Line Execution
Another way to run Julia code is via command line execution, which is particularly useful for running scripts, automating tasks, and working in terminal-based environments. To execute a Julia script from the command line, simply type julia <script_name>.jl
, where <script_name>
is the name of the Julia file you want to run.
Performance and Compatibility
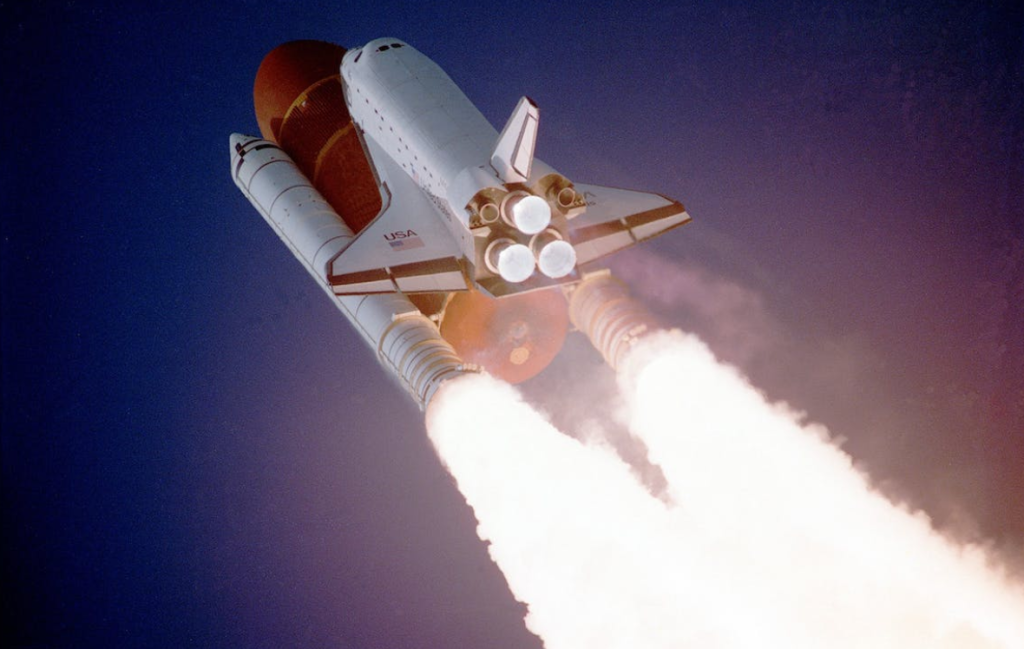
Performance Tips
Julia is a powerful and dynamic language that provides performance comparable to traditional statically-typed languages like C and Fortran. To make the most of this performance, there are a few tips to keep in mind. First, always place performance-critical code inside functions, as this allows the Julia compiler to optimize it better.
It is also essential to understand and utilize Julia’s type system, as it plays a significant role in optimizing the code. Make sure to declare types for your variables to help the compiler generate optimal machine code, leading to improved performance.
Another performance tip is to use in-place operations whenever possible, as they avoid memory allocations and reduce processing overhead.
Integration with Other Languages
One of Julia’s strengths is its ability to integrate with other programming languages for better compatibility and increased functionality. It can easily interoperate with C, R, Python, C++, Lua, Mathematica, Lisp, and Perl, making it a versatile tool for a wide range of projects.
For C and Fortran, Julia provides straightforward syntax and native functions, allowing you to call C and Fortran libraries directly, improving performance and ease of use.
Python is another popular language, and Julia offers seamless integration with Python through the PyCall package. This allows you to call Python functions and libraries from Julia code, providing greater flexibility and access to a wide range of Python packages.
Libraries and Packages
In Julia programming language, libraries and packages play a crucial role in expanding its capabilities and providing support for various tools and functionalities. This section discusses the Standard Library, External Packages, and the process of Creating Your Own Packages.
Standard Library
Julia’s Standard Library contains a wide range of essential modules, which provide support for scientific computing and other core functionalities. Some of the key components include:
- Base: The foundation of Julia’s standard library, containing basic data structures, functions, and essential operators.
- LinearAlgebra: Provides comprehensive support for linear algebra operations, including matrix manipulation and decomposition.
- Random: Functions for generating random numbers and sampling from distributions.
- SharedArrays: Allows creating and manipulating shared memory arrays for efficient parallel processing.
The Standard Library is maintained and distributed with Julia’s core distribution, ensuring consistency and compatibility across different versions of the language.
External Packages
In addition to the Standard Library, Julia has a thriving ecosystem of external packages. These packages are developed and maintained by the community, providing extensive support for various domains, including machine learning, optimization, and statistical analysis. Julia’s package manager, Pkg, facilitates the installation, updating, and removal of these external packages. Some popular external packages include:
- JuMP: A powerful optimization modeling language that makes it easy to define and solve optimization problems.
- DifferentialEquations: A comprehensive suite of solvers for differential equation systems.
- DataFrames: Provides convenient data manipulation tools and data structures for working with large datasets.
To find and explore more packages, you can utilize resources such as JuliaHub or Julia.jl, which offer package search, documentation, and navigation by tags/keywords.
Creating Your Own Packages
Developing your own Julia package can help you share your work with the community or create reusable code components for your projects. Before creating a package, make sure it’s not already available in the ecosystem. Once you have identified a need, follow these general steps:
- Environment: Ensure you have the latest version of Julia and Pkg.
- Initialization: Use the
Pkg.generate()
function to create an initial package structure, which includes the essential files and directories, such asProject.toml
,src
, andtest
. - Implementation: Develop your package’s functionality within the
src
directory, adhering to Julia’s best practices, such as modularizing your code and using appropriate type annotations. - Testing and Documentation: Write tests in the
test
folder and provide clear documentation to help users understand your package’s functions.
After completing these steps, you can register your package with the Julia General registry, making it available for the community to use and contribute.
In conclusion, the extensive availability of libraries and packages in Julia is essential for the language’s support and growth within the scientific computing community, as well as other domains. By understanding the role of the Standard Library, external packages, and creating your own packages, you can make the most of Julia’s powerful ecosystem.
Data Science and Machine Learning
Julia is a versatile programming language that has been gaining traction in the fields of data science and machine learning. Its high-level, dynamic nature allows for fast and efficient numerical analysis while remaining user-friendly, similar to Python.
One of its key advantages is its ability to handle extensive computational workloads, making it a perfect fit for data science applications. Julia’s ecosystem offers various packages for data manipulation, analysis, and visualization, allowing data scientists to perform a wide range of tasks with ease.
Packages like DataFrames.jl, StatsBase.jl, and Plots.jl enable users to work with tabular data, perform necessary statistical functions, and create visually appealing plots, respectively.
Machine learning has also become increasingly important in the world of data science, and Julia is well-equipped to handle this field.
With packages like MLJ.jl and Flux.jl, users can create sophisticated machine learning models and perform complex training tasks. These packages come with a variety of tools to create, train, and validate models, making the machine learning process more streamlined and accessible.
- DataFrames.jl: Data manipulation and representation
- StatsBase.jl: Statistical functions and utilities
- Plots.jl: Data visualization and plotting
- MLJ.jl: Machine Learning in Julia
- Flux.jl: A powerful machine learning library
In terms of visualization, the flexibility of Julia allows for the creation of custom plots and charts suitable for any data processing or analysis task. The Plots.jl package is particularly popular due to its ease of use, customization options, and compatibility with multiple backends such as GR, Plotly, and PyPlot.
Julia’s advantages in data science and machine learning are owed to its impressive performance capabilities. With its just-in-time (JIT) compilation, Julia provides the speed of lower-level languages such as C/C++ while maintaining usability comparable to Python. This potent combination of performance and ease-of-use makes Julia a powerful choice for professionals and hobbyists in data science and machine learning.
Language Features
Types and Unicode
Julia is a high-level, dynamically-typed language with optional typing. This feature allows developers to write efficient and fast code while also maintaining clarity in their programs. Julia’s type inference ensures optimal performance during runtime, as the compiler can generate specialized code for different data types (source).
Additionally, Julia has excellent support for Unicode characters. It can handle strings and character literals containing Unicode characters, making it suitable for internationalization and working with various data sources. Julia’s robust Unicode handling capabilities provide programmers with the flexibility to work with diverse character sets in their programs.
High-Level Constructs
Julia offers a range of high-level constructs that contribute to its simplicity, expressiveness, and power. The language employs multiple dispatch, which allows developers to define function behavior depending on the combination of argument types rather than just a single argument type. This feature makes Julia particularly well-suited for implementing mathematical operations and polymorphic functions (source).
Moreover, Julia integrates imperative, functional, and object-oriented programming paradigms, providing developers with a versatile toolkit for implementing various coding styles. High-level constructs like macros, metaprogramming, and code generation contribute to Julia’s ease of use and extensibility.
In summary, Julia’s features like optional typing, Unicode support, and high-level constructs make it an attractive choice for developers seeking efficiency, flexibility, and expressiveness in a programming language.
Compiling and Building from Source Code
When it comes to installing the Julia programming language, there are two primary methods: downloading precompiled binaries or compiling and building from source code. In this section, we will focus on compiling and building Julia from its source code.
Before you begin, ensure that you have the required resources such as a compatible operating system, the Julia source code, and the necessary software tools. You can download the source code from the official Julia GitHub repository.
First, clone the Julia repository to your local machine using git
. This command downloads the latest version of the source code:
git clone git://github.com/JuliaLang/julia.git
Once you’ve cloned the repository, navigate to the julia directory and run the following command to begin the compiling and building process:
make
This command compiles the source code and builds the Julia executable. Depending on your system, the process might take a considerable amount of time. It’s important to have a stable internet connection, as additional packages might be downloaded during the build process. If you encounter any errors during compilation, it’s helpful to consult the Building Julia (Detailed) guide or seek assistance from the Julia community.
After the build process is complete, you can find the Julia executable in the newly created bin
folder. To run Julia, use the following command:
./julia
Now that you’ve compiled and built Julia from source code, you can use it to execute your code and access the language’s powerful features. It’s important to keep your installation up to date to ensure compatibility and access to the latest language improvements. To update your installation, simply pull the latest version from the Julia repository and re-run the make
command.
Documentation and Learning Resources
The Julia programming language offers a comprehensive set of documentation targeted towards users with varying expertise levels. This documentation covers fundamental topics such as syntax, mathematical operations, and other essential language components. You can find the official Julia documentation in PDF format, which conveniently becomes a single downloadable file for offline use.
Learning resources for Julia can be found at julialang.org/learning/. These resources cater to both beginners and advanced users, ensuring that you have all the necessary tools at your disposal while exploring the language. Julia’s built-in documentation system enables package developers and users to document functions, types, and other objects easily, with the use of docstrings. This encourages a collaborative work environment in which users can easily access and learn from each other’s experiences.
The Julia manual serves as another learning resource, providing a more in-depth look into the intricacies of the language. This manual is ideal for those who prefer a detailed and organized study guide when learning a new programming language. As you gain proficiency in Julia, you may also want to consider exploring Julia’s YouTube channel, where you will find a wealth of tutorials and talks on various topics.
One of the best ways to learn and understand Julia is by engaging in hands-on activities. As a new user, you can start with the Getting Started with Julia guide, which walks you through the installation process and essential programming concepts. Furthermore, you can join the vibrant Julia community by asking questions, seeking advice, and discussing ideas in the Julia forums, where you can interact with other Julia enthusiasts.
Frequently Asked Questions
How can I find out which Julia version is installed?
To find out which Julia version is installed on your system, open the Julia REPL by running julia
in the command line. Upon starting, the REPL will display the installed Julia version right beneath the Julia logo. Alternatively, you can execute the command versioninfo()
in the Julia REPL, which will provide you with comprehensive information, including the Julia version.
What are the steps to update Julia to the latest version?
To update Julia to the latest version, you must first download the latest release from the official Julia website. After downloading, follow the installation instructions for your operating system to install the new version. Note that updating Julia might require you to remove or update your existing packages to ensure compatibility with the new version.
How do I check the version of a specific Julia package?
To check the version of a specific Julia package, open the Julia REPL and enter package mode by typing ]
. In package mode, execute the command status <PackageName>
, replacing <PackageName>
with the name of the package you want to check the version of. The REPL will display the installed version of the package in your active environment.
Where can I download the Julia programming language?
You can download the Julia programming language from the official Julia website. Choose the version appropriate for your operating system and follow the installation instructions provided.
How can I switch between different Julia versions in VSCode?
To switch between different Julia versions in VSCode, open the Julia extension settings by clicking the gear icon in the lower left corner of the interface. Locate the “Julia: Executable Path” setting, and provide the path to the desired Julia executable. You may need to restart VSCode for the changes to take effect.
What should I do to solve the ‘failed to figure out Juliaup channel’ error?
The ‘failed to figure out Juliaup channel’ error might occur when using the Juliaup command-line tool for managing multiple Julia installations. To resolve the error, ensure that the Juliaup binary is up-to-date by running juliaup self update
. If the issue persists, consider reinstalling Juliaup or filing an issue on the Juliaup GitHub repository for further assistance.
June 24, 2023 at 07:33PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
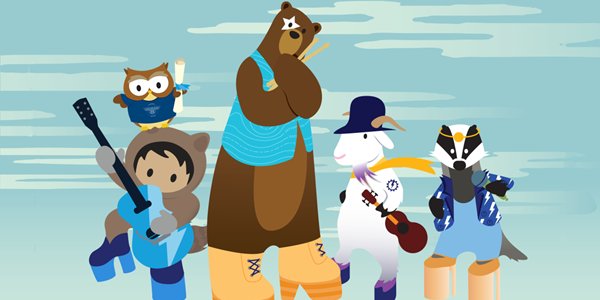
Post a Comment