Real Python: Iterators and Iterables in Python: Run Efficient Iterations :
by:
blow post content copied from Planet Python
click here to view original post
Python’s iterators and iterables are two different but related tools that come in handy when you need to iterate over a data stream or container. Iterators power and control the iteration process, while iterables typically hold data that you want to iterate over one value at a time.
Iterators and iterables are fundamental components of Python programming, and you’ll have to deal with them in almost all your programs. Learning how they work and how to create them is key for you as a Python developer.
In this tutorial, you’ll learn how to:
- Create iterators using the iterator protocol in Python
- Understand the differences between iterators and iterables
- Work with iterators and iterables in your Python code
- Use generator functions and the
yield
statement to create generator iterators - Build your own iterables using different techniques, such as the iterable protocol
- Use the
asyncio
module and theawait
andasync
keywords to create asynchronous iterators
Before diving deeper into these topics, you should be familiar with some core concepts like loops and iteration, object-oriented programming, inheritance, special methods, and asynchronous programming in Python.
Free Sample Code: Click here to download the free sample code that shows you how to use and create iterators and iterables for more efficient data processing.
Understanding Iteration in Python
When writing computer programs, you often need to repeat a given piece of code multiple times. To do this, you can follow one of the following approaches:
- Repeating the target code as many times as you need in a sequence
- Putting the target code in a loop that runs as many times as you need
The first approach has a few drawbacks. The most troublesome issue is the repetitive code itself, which is hard to maintain and not scalable. For example, the following code will print a greeting message on your screen three times:
# greeting.py
print("Hello!")
print("Hello!")
print("Hello!")
If you run this script, then you’ll get 'Hello!'
printed on your screen three times. This code works. However, what if you decide to update your code to print 'Hello, World!'
instead of just 'Hello!'
? In that case, you’ll have to update the greeting message three times, which is a maintenance burden.
Now imagine a similar situation but with a larger and more complex piece of code. It can become a nightmare for maintainers.
Using a loop will be a much better way to solve the problem and avoid the maintainability issue. Loops allow you to run a piece of code as often as you need. Consider how you’d write the above example using a while
loop:
>>> times = 0
>>> while times < 3:
... print("Hello!")
... times += 1
...
Hello!
Hello!
Hello!
This while
loop runs as long as the loop-continuation condition (times < 3
) remains true. In each iteration, the loop prints your greeting message and increments the control variable, times
. Now, if you decide to update your message, then you just have to modify one line, which makes your code way more maintainable.
Python’s while
loop supports what’s known as indefinite iteration, which means executing the same block of code over and over again, a potentially undefined number of times.
You’ll also find a different but similar type of iteration known as definite iteration, which means going through the same code a predefined number of times. This kind of iteration is especially useful when you need to iterate over the items of a data stream one by one in a loop.
To run an iteration like this, you typically use a for
loop in Python:
>>> numbers = [1, 2, 3, 4, 5]
>>> for number in numbers:
... print(number)
...
1
2
3
4
5
In this example, the numbers
list represents your stream of data, which you’ll generically refer to as an iterable because you can iterate over it, as you’ll learn later in this tutorial. The loop goes over each value in numbers
and prints it to your screen.
When you use a while
or for
loop to repeat a piece of code several times, you’re actually running an iteration. That’s the name given to the process itself.
In Python, if your iteration process requires going through the values or items in a data collection one item at a time, then you’ll need another piece to complete the puzzle. You’ll need an iterator.
Getting to Know Python Iterators
Iterators were added to Python 2.2 through PEP 234. They were a significant addition to the language because they unified the iteration process and abstracted it away from the actual implementation of collection or container data types. This abstraction allows iteration over unordered collections, such as sets, ensuring every element is visited exactly once.
Read the full article at https://realpython.com/python-iterators-iterables/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
March 01, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
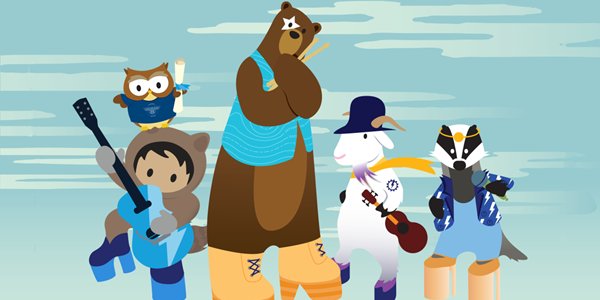
Post a Comment