Python Integer to Hex — The Ultimate Guide : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Working with different number systems and their representations is a common practice in the world of programming. One such conversion involves changing integer values into their corresponding hexadecimal representations. In Python, this transformation can be achieved with ease by utilizing built-in functions and string formatting techniques.
Hexadecimal, also known as base-16, is a number system that employs sixteen distinct symbols made up of 0-9 digits and the letters A-F. It is particularly useful in computing and digital electronics since it represents large numbers concisely and aligns well with the byte size (8 bits) commonly used in computer systems.
In this article, we’ll explore various methods for converting integers to their hex equivalents in Python.
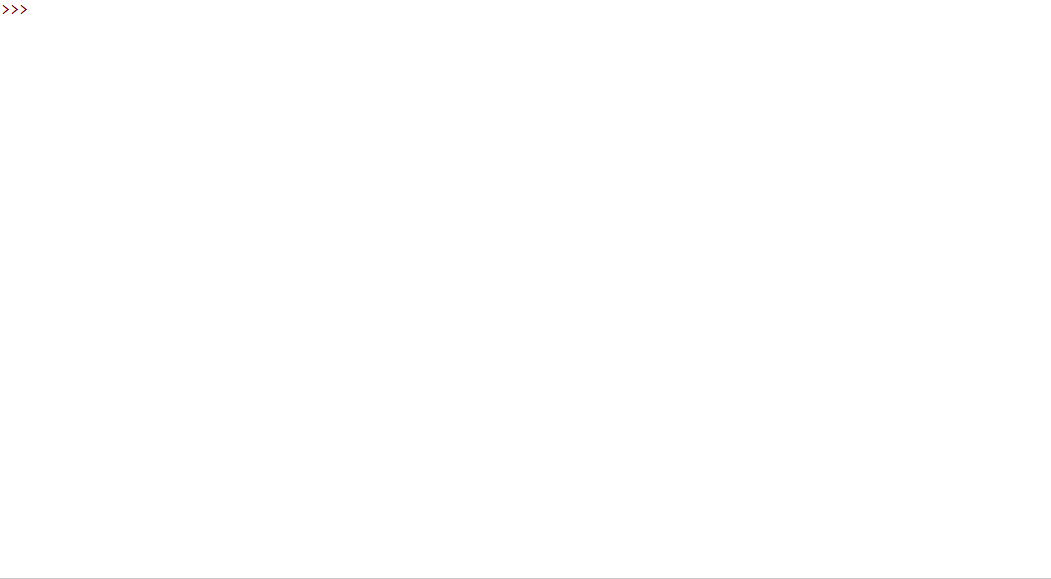
Python provides several ways to convert an integer to its corresponding hexadecimal representation, including the built-in hex()
function and string formatting. By mastering these techniques, you can easily handle various use cases involving hexadecimal number manipulations.
Understanding Integers and Hexadecimal Representation
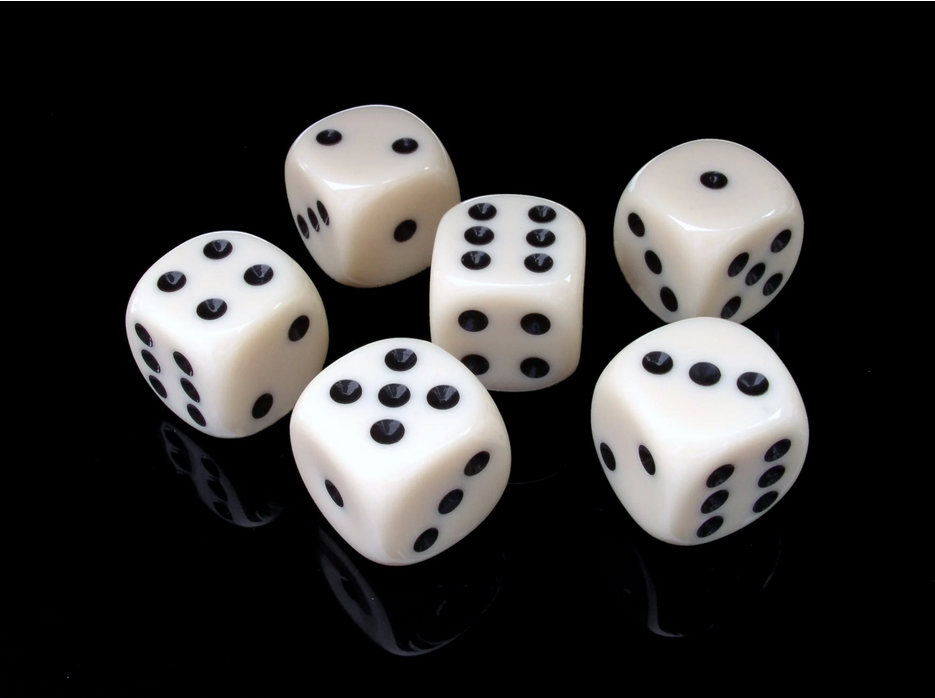
Integer Basics
An integer is a whole number that can be either positive, negative, or zero. In Python, integers are numbers without decimals and are represented using the built-in int
data type. They can be used for various mathematical operations such as addition, subtraction, multiplication, and division.
Here’s an example of Python code with integers:
x = 5 y = -3 result = x + y print(result) # Output: 2
Hexadecimal Basics
Hexadecimal is a number system that uses base 16 instead of base 10, like the decimal system. It is more convenient to represent binary data, as each hexadecimal digit can represent four or two binary digits (a byte). The 16 symbols used in hexadecimal are 0-9 and A-F, where A stands for 10, B for 11, up to F for 15.
When working with hexadecimals in Python, the hex()
function can be used to convert an integer to a lowercase hexadecimal string prefixed with '0x'
for positive integers and '-0x'
for negative integers.
For example:
integer_number = 255 hex_string = hex(integer_number) print(hex_string) # Output: '0xff'
Converting back to an integer from a hexadecimal string can be done using the int()
function with the base parameter set to 16:
hexadecimal_string = '0xff' integer_value = int(hexadecimal_string, 16) print(integer_value) # Output: 255
Converting Integers to Hexadecimal in Python
In this section, we will explore different methods to convert integers to hexadecimal in Python. We will look at three common ways to achieve this:
- Using the
hex()
Function - Using
format()
Function - Using F-Strings
Using the hex() Function

The hex()
function is a built-in Python function that converts an integer to its corresponding hexadecimal representation. The syntax of the function is:
hex(N)
Where N
is the integer to be converted to hexadecimal. The function returns the hexadecimal string with a '0x'
prefix. For instance,
int_number = 42 hex_string = hex(int_number) print(hex_string)
The output would be: 0x2a
.
You can watch my explainer video on this function right here on the blog:

Using format() Function
You can also use the format()
function to convert an integer to a hexadecimal string. The syntax for this method is:
format(N, 'X')
Where N is the integer to be converted, and ‘X’ specifies uppercase hexadecimal representation. You can use ‘x’ for lowercase hexadecimal representation.
For example:
int_number = 42 hex_string = format(int_number, 'X') print(hex_string)
The output would be: 2A
.
Using F-Strings
Another method to convert integers to their hexadecimal representations is to use f-strings, introduced in Python 3.6. The syntax for using f-strings is as follows:
f"{N:X}"
Where N
is the integer to be converted, and 'X'
specifies uppercase and 'x'
specifies lowercase hexadecimal representation.
Here’s an example:
int_number = 42 hex_string = f"{int_number:X}" print(hex_string)
The output would be: 2A
.
In this section, we explored three different methods for converting integers to hexadecimal in Python. You can choose the method that best suits your needs and is compatible with your version of Python.
Handling Negative Integers and Zero

Converting negative integers and zero to their corresponding hexadecimal representation in Python can be achieved using the hex()
function, which produces a string output prefixed with '0x'
for positive integers and '-0x'
for negative integers. The hex()
function can handle the conversion of zero as well.
Representing negative numbers in hexadecimal relies on the two’s complement format. However, Python’s built-in hex()
function cannot directly provide the two’s complement representation. Consequently, additional steps are required.
Below is a brief procedure to obtain the two’s complement representation for negative integers:
- Specify the desired bit length for the representation (e.g., 8 bits, 16 bits, or 32 bits).
- Calculate the two’s complement value by adding the negative integer to 2 raised to the power of the specified bit length.
- Convert the resulting integer to its corresponding hexadecimal representation.
Here is an example of converting a negative integer to its two’s complement hexadecimal representation using a defined function:
def twos_complement_hex(number, bit_length): if number < 0: complement = (1 << bit_length) + number else: complement = number return hex(complement)
This function handles the conversion of negative integers to their corresponding two’s complement hexadecimal values based on the specified bit length.
For example, twos_complement_hex(-10, 8)
would produce the output ‘0xf6
‘. Use this function to handle the conversion of negative integers and zero to their hexadecimal representation in Python.
Examples and Use Cases
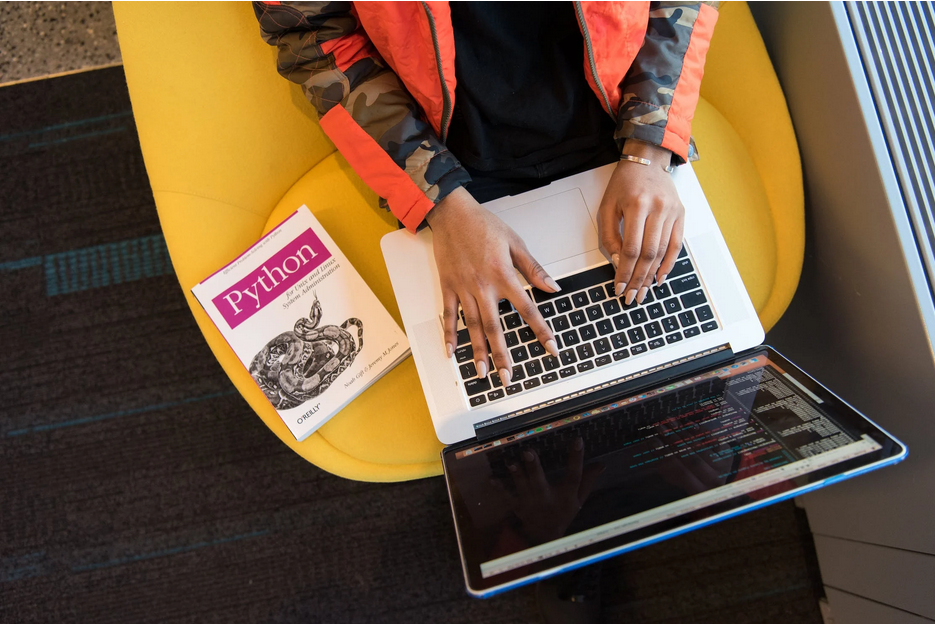
Converting integers to hexadecimal values in Python is common in various programming scenarios. This can be useful for debugging, memory allocation, and more tasks.
Let’s take a look at a few examples and use cases of the Python hex() function and other alternatives.
Using the hex() function:
integer_value = 42 hex_value = hex(integer_value) print(hex_value) # Output: 0x2a
This example demonstrates how to use the built-in hex()
function in Python to convert an integer value to a lowercase hexadecimal string prefixed with '0x'
.
Formatting with f-strings:
integer_value = 42 hex_value = f"{integer_value:x}" print(hex_value) # Output: 2a
For Python >= 3.6, you can use f-string formatting to convert integers to hexadecimal strings without the '0x'
prefix, as shown in this Stack Overflow discussion.
Using the format() function for uppercase hexadecimal:
integer_value = 42 hex_value = format(integer_value, 'X') print(hex_value) # Output: 2A
In this example from Tutorial Kart, the format()
function is utilized to convert an integer to an uppercase hexadecimal string without the '0x'
prefix.
Considerations for Large Numbers and Performance
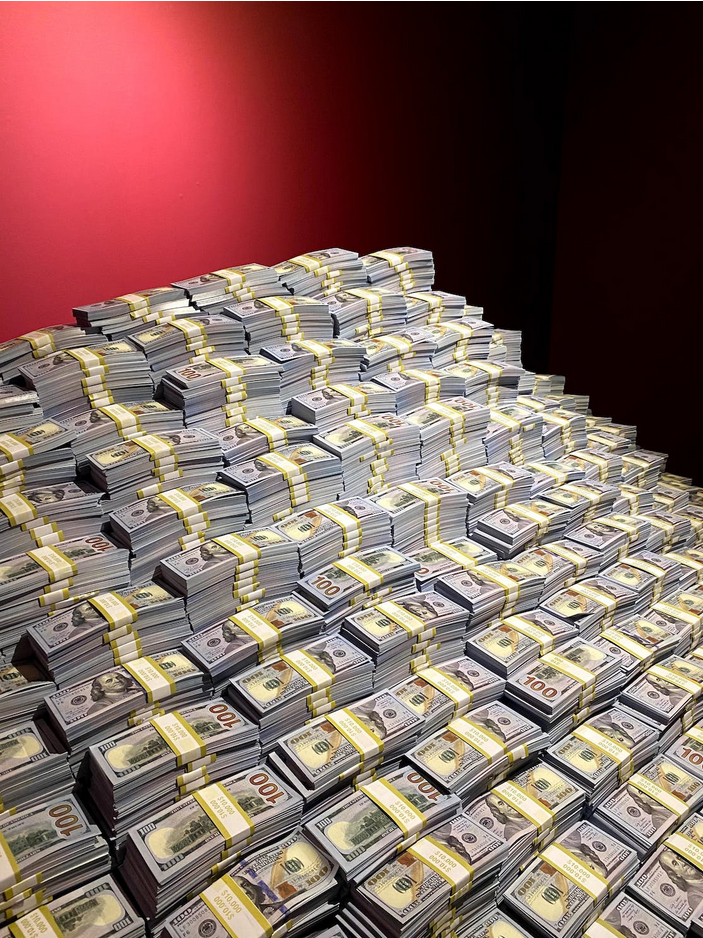
Handling large integers in Python can introduce specific challenges related to performance and representation. When converting large integers to hexadecimal, the choice of method and efficient implementation can greatly impact the execution time and memory usage of the code.
Python’s built-in hex()
function can be used to convert integers to hexadecimal strings, even for large numbers. This function returns a lowercase hexadecimal string prefixed with “0x” (source). However, it is important to note that the time required for conversion will also increase with the increasing size of integers.
In some cases, it might be beneficial to consider alternative representations for large integers, such as using hexadecimal strings directly.
In such cases, the int()
function can be particularly handy as it accepts base values from 2 to 36 (source). For example:
hex_value = "1A2B3C4D" integer_value = int(hex_value, 16) # Converts the hexadecimal string to an integer
When working with large numbers, evaluating the balance between readability and performance is important.
For instance, printing or parsing of very large integers might introduce bugs or performance issues and might require special treatment, as seen in some Python bugfix releases (source).
To optimize performance, it is recommended to use native Python functions and libraries or to explore third-party libraries for more efficient implementations suited for large numbers, as well as to profile your code to identify potential bottlenecks and resource-intensive operations.
Recommended: 7 Performance Tuning Tips
Python Int to Hex Without 0x
Converting integers to hexadecimal values in Python is commonly done with the built-in hex()
function.
However, this function returns a hexadecimal string with the "0x"
prefix, which might not be desired in certain situations. In this section, we will discuss a few methods to obtain a hexadecimal representation of an integer without the "0x"
prefix.
One simple method to remove the “0x
” prefix is to use string slicing. By starting with index 2 of the hexadecimal string returned by the hex()
function, we effectively skip the “0x
” part.
Here’s an example:
integer_value = 42 hex_string = hex(integer_value)[2:] print(hex_string) # Output: "2a"
Another approach is to utilize f-strings, which support the Format Specification Mini-Language in Python 3. This allows for direct control over the format of the output, including the display of hexadecimal numbers without the “0x
“.
An example using f-strings is as follows:
integer_value = 42 hex_string = f"{integer_value:x}" print(hex_string) # Output: "2a"
Both of these methods provide clean and efficient ways of converting integers to hexadecimal strings without the “0x
” prefix, making them suitable for various use cases in Python programming.
Python Int to Hex 2 Digit
In Python, converting an integer to a 2-digit hexadecimal number is a common task. There are a couple of ways you can achieve this conversion. Let’s explore some methods for generating 2-digit hex numbers from integers.
One way to generate a 2-digit hexadecimal number is by using the built-in Python function hex()
. However, this method might not always return a 2-digit hex number. To obtain the desired result, you can use the format()
function:
my_integer = 42 hex_string = format(my_integer, '02X')
In this example, the format()
function takes two arguments. The first argument is the integer you want to convert, and the second argument is a string specifying the format.
Here, the string '02X'
indicates that we want a 2-digit uppercase hexadecimal number. If you prefer lowercase hexadecimal numbers, replace 'X'
with 'x'
.
Another approach to generate a 2-digit hex number is by using the %-style formatting. This method is considered old-fashioned but still works:
my_integer = 42 hex_string = '%.2X' % my_integer
This code performs the same task as the previous example, but it utilizes %-style formatting instead of the format()
function.
Both methods mentioned above will provide you with a 2-digit hex number from an integer, making your conversions straightforward and convenient in your Python projects.
Python Int to Hex With Padding
When converting integers to hexadecimal strings in Python, it’s common to require padding with zeros to achieve a consistent length. Utilizing Python’s built-in tools like f-strings and the format function, achieving this goal is simple and efficient.
For Python 3.6 and above, you can use f-strings. The syntax is concise and easy to read:
value = 42 padding = 6 hex_string = f"{value:#0{padding}x}"
In this example, value
contains the integer to be converted, and padding
stores the desired length of the output hexadecimal string. The f-string then uses #0{padding}x
to format the value with the desired padding, including the “0x” prefix (source).
If you’re working with older versions of Python, using the .format()
method of strings to achieve the same result is recommended:
value = 42 padding = 8 hex_string = "0x{:0{padding}X}".format(value, padding=padding)
The .format()
method specifies a zero-padded hexadecimal representation of the integer by using {:0{padding}X}
, with padding
as the desired number of characters in the output string (source).
Python Integer to Hex With Leading Zeros
To convert an integer to a hexadecimal string with leading zeros in Python, you can use the format()
function. You’ll need to specify the number of leading zeros you want in the output.
Here’s an example:
# Convert an integer to a hexadecimal string with leading zeros def int_to_hex_with_leading_zeros(number, zeros=8): return format(number, f'0{zeros}x') # Test the function number = 12345 hex_string = int_to_hex_with_leading_zeros(number, 8) print(hex_string)
In this example, the int_to_hex_with_leading_zeros
function converts the given integer to a hexadecimal string with a specified number of leading zeros. The default number of leading zeros is set to 8.
The format()
function formats the number with the specified formatting string, which includes the number of zeros and the 'x'
for hexadecimal. The output for the example will be:
00003039
You can adjust the number of leading zeros by changing the zeros
parameter.
Python Integer to Hex Color
To convert an integer to a hex color string in Python, you can use the following function. It takes an integer and converts it to a 6-digit hexadecimal color string, including the leading '#'
sign.
def int_to_hex_color(number): return "#{:06x}".format(number % (1 << 24)) # Test the function number = 123456 hex_color = int_to_hex_color(number) print(hex_color)
In this example, the int_to_hex_color
function converts the given integer to a 6-digit hexadecimal color string by using the format string "{:06x}"
, which ensures that the output has exactly 6 digits with leading zeros, if necessary. The number is first taken modulo 2^24 (1 << 24) to ensure it’s within the valid color range (0 to 16777215).
The output for the example will be:
#01e240
Keep in mind that if you provide an integer greater than 16777215, it will wrap around and may not give you the desired color.
March 23, 2023 at 07:49PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
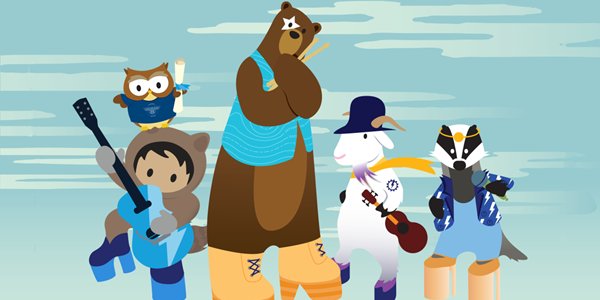
Post a Comment