Build a Maze Solver in Python Using Graphs :
by:
blow post content copied from Real Python
click here to view original post
If you’re up for a little challenge and would like to take your programming skills to the next level, then you’ve come to the right place! In this hands-on tutorial, you’ll practice object-oriented programming, among several other good practices, while building a cool maze solver project in Python.
From reading a maze from a binary file, to visualizing it using scalable vector graphics (SVG), to finding the shortest path from the entrance to the exit, you’ll go step by step through the guided process of building a complete and working project.
In this tutorial, you’ll learn how to:
- Use an object-oriented approach to represent the maze in memory
- Define a specialized binary file format to store the maze on disk
- Transform the maze into a traversable weighted graph
- Use graph search algorithms in the NetworkX library to find the solution
- Visualize the maze and its solution using scalable vector graphics (SVG)
Click the link below to download the complete source code for this project, along with the supporting materials, which include a few sample mazes:
Free Download: Click here to download the source code and supporting materials that you’ll use to build a maze solver in Python.
Demo: Python Maze Solver
At the end of this tutorial, you’ll have a command-line maze solver that can load your maze from a binary file and show its solution in the web browser:
You’ll learn how to build your own mazes like this from scratch and save them on disk. In the meantime, feel free to grab one of the sample mazes from the supporting materials. Now, get ready to dive in!
Project Overview
Take a glimpse at the expected file structure of your project. Once finished, your project’s file and directory tree will look as follows:
maze-solver/
│
├── mazes/
│ ├── labyrinth.maze
│ ├── miniature.maze
│ └── pacman.maze
│
├── src/
│ │
│ └── maze_solver/
│ │
│ ├── graphs/
│ │ ├── __init__.py
│ │ ├── converter.py
│ │ └── solver.py
│ │
│ ├── models/
│ │ ├── __init__.py
│ │ ├── border.py
│ │ ├── edge.py
│ │ ├── maze.py
│ │ ├── role.py
│ │ ├── solution.py
│ │ └── square.py
│ │
│ ├── persistence/
│ │ ├── __init__.py
│ │ ├── file_format.py
│ │ └── serializer.py
│ │
│ ├── view/
│ │ ├── __init__.py
│ │ ├── decomposer.py
│ │ ├── primitives.py
│ │ └── renderer.py
│ │
│ ├── __init__.py
│ └── __main__.py
│
├── pyproject.toml
└── requirements.txt
Yes, that’s a lot of files, but don’t worry! Most of them are fairly short, and some contain only a few lines of code. This helps keep things organized and makes the individual pieces reusable, letting you compose them in new ways. Such granularity also plays an important role in Python projects with larger codebases by avoiding the notorious circular dependency error that you might encounter if various parts of the code were in one big file.
The mazes/
subfolder is home to a few binary files with sample data that you’re going to use in this tutorial. You can get these files, along with the final source code and snapshots of the individual steps, by downloading the supporting materials:
Free Download: Click here to download the source code and supporting materials that you’ll use to build a maze solver in Python.
The src/
subfolder contains your Python modules and packages for the maze solver project. The maze_solver
package consists of several subpackages that group logically related code fragments, including:
graphs
: The traversal and conversion of the maze to a graph representationmodels
: The building blocks of the maze and its solutionpersistence
: A custom binary file format for persistent maze storageview
: The visualization of the graph with scalable vector graphics
You’ll also find the special __main__.py
file, which makes the enclosing package runnable so that you can execute it directly from the command line using Python’s -m
option:
$ python -m maze_solver /path/to/sample.maze
When launched like this, the package reads the specified file with your maze. After solving the maze, it renders the solution into an SVG format embedded in a temporary HTML file. The file gets automatically opened in your default web browser. You can also run the same Python code using a shortcut command:
$ solve /path/to/sample.maze
It’ll work as long the solve
command isn’t already taken or aliased by another program.
Finally, pyproject.toml
provides your project’s configuration, metadata, and dependencies defined in the TOML format. The project only depends on one external library, which you’ll use to find the shortest path in the maze represented as a graph.
Next up, you’ll review a list of relevant resources that might become your savior in case you get stuck at any point. Also, remember the supporting materials, which contain a snapshot of each finished step. Along the way, you can compare your progress to the relevant step to ensure that you’re on the right track.
Read the full article at https://realpython.com/python-maze-solver/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
March 29, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
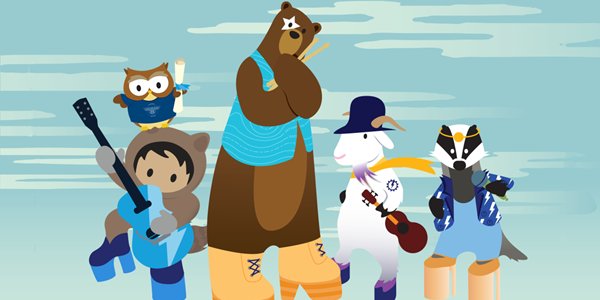
Post a Comment