Python Int to String with Leading Zeros : Chris
by: Chris
blow post content copied from Finxter
click here to view original post
To convert an integer i
to a string with leading zeros so that it consists of 5
characters, use the format string f'{i:05d}'
. The d
flag in this expression defines that the result is a decimal value. The str(i).zfill(5)
accomplishes the same string conversion of an integer with leading zeros.
Challenge: Given an integer number. How to convert it to a string by adding leading zeros so that the string has a fixed number of positions.
Example: For integer 42, you want to fill it up with leading zeros to the following string with 5 characters: '00042'
.
In all methods, we assume that the integer has less than 5 characters.
Method 1: Format String
The first method uses the format string feature in Python 3+. They’re also called replacement fields.
# Integer value to be converted i = 42 # Method 1: Format String s1 = f'{i:05d}' print(s1) # 00042
The code f'{i:05d}'
places the integer i into the newly created string. However, it tells the format language to fill the string to 5
characters with leading '0'
s using the decimal system. This is the most Pythonic way to accomplish this challenge.
Method 2: zfill()
Another readable and Pythonic way to fill the string with leading 0s is the string.zfill()
method.
# Method 2: zfill() s2 = str(i).zfill(5) print(s2) # 00042
The method takes one argument and that is the number of positions of the resulting string. Per default, it fills with 0s.
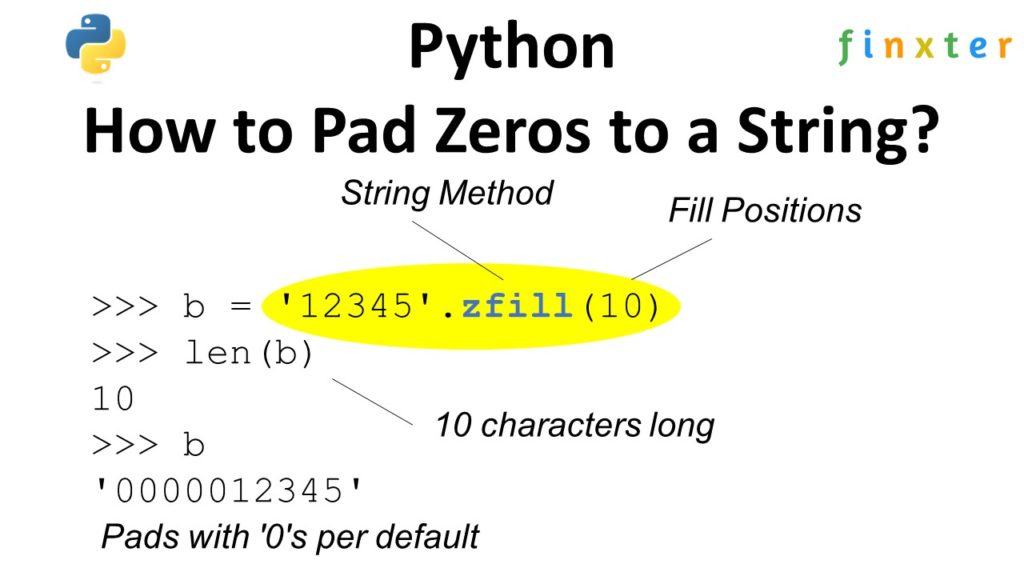
You can check out the following video tutorial from Finxter Adam:

Method 3: List Comprehension
Many Python coders don’t quite get the f-strings and the zfill()
method shown in Methods 2 and 3. If you don’t have time learning them, you can also use a more standard way based on string concatenation and list comprehension.
# Method 3: List Comprehension s3 = str(i) n = len(s3) s3 = '0' * (5-len(s3)) + s3 print(s3)
You first convert the integer to a basic string. Then, you create the prefix of 0
s, you need to fill it up to n=5
characters and concatenate it to the integer’s string representation. The asterisk operator creates a string of 5-len(s3)
zeros here.
Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
If your answer is YES!, consider becoming a Python freelance developer! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
Programmer Humor
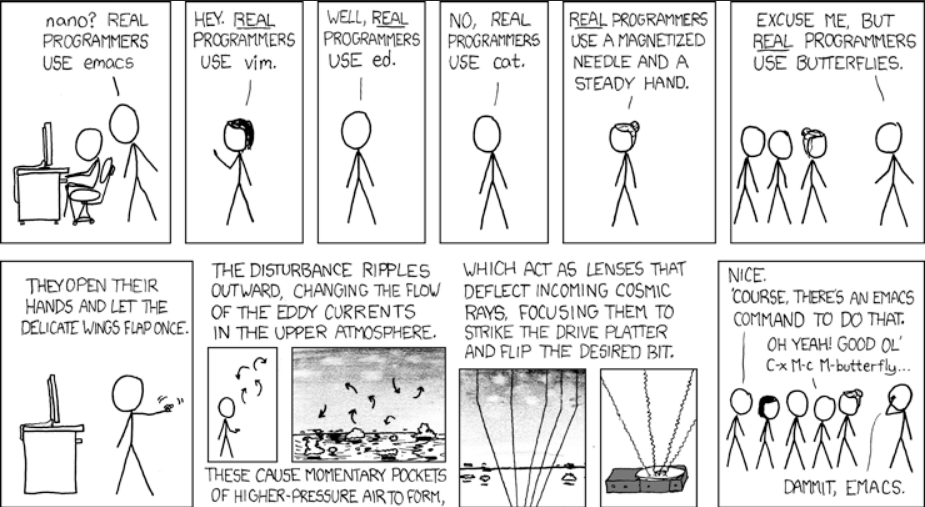
February 25, 2023 at 06:42PM
Click here for more details...
=============================
The original post is available in Finxter by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
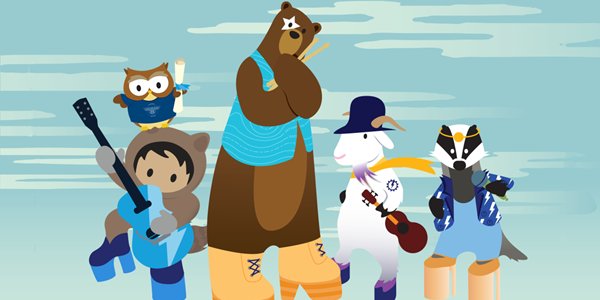
Post a Comment