Python for Beginners: Extract Unique Characters From String in Python :
by:
blow post content copied from Planet Python
click here to view original post
Strings are extensively used for text analysis in Python. This article discusses how to extract unique characters from a string in Python.
Extract Unique Characters From String in Python Using for Loop
To extract unique characters from a string using for loop, we will first define an empty list to store the output characters. Then, we will iterate through the string characters using a for loop. While iteration, we will check if the current character is present in the list or not. If yes, we will move to the next character. Otherwise, we will add the current character to the list using the append()
method. The append()
method, when invoked on a list, takes the character as its input argument and appends it to the end of the list.
After execution of the for loop, we will get all the unique characters of the string in our list. You can observe this in the following example.
myStr="Python For Beginners"
print("The input string is:",myStr)
output=[]
for character in myStr:
if character not in output:
output.append(character)
print("The output is:",output)
Output:
The input string is: Python For Beginners
The output is: ['P', 'y', 't', 'h', 'o', 'n', ' ', 'F', 'r', 'B', 'e', 'g', 'i', 's']
In the above example, we have used the string “Python For Beginners”. After execution of the for loop, we get the list of unique characters from the string.
Extract Unique Characters From String in Python Using Sets
Python set data structure is used to store unique immutable objects together. As python strings as well as individual characters are immutable, we can use sets to extract characters from a string in Python.
To extract unique characters from a string in Python, we will first create an empty set. Then, we will iterate through the string characters using a for loop. While iteration, we will add the current character to the set using the add()
method. The add()
method, when invoked on a set, takes the character as its input argument and adds it to the set if it is not already present there.
After execution of the for loop, we will get all the unique characters of the string in our set. You can observe this in the following example.
myStr="Python For Beginners"
print("The input string is:",myStr)
output=set()
for character in myStr:
output.add(character)
print("The output is:",output)
Output:
The input string is: Python For Beginners
The output is: {'F', 'e', 'y', 't', 'h', 'P', 'i', 'g', ' ', 'o', 's', 'r', 'n', 'B'}
Instead of using the for loop and add()
method, you can use the update()
method on a set to extract unique characters from a string in Python. The update()
method, when invoked on a set object, takes an iterable object as its input argument. After execution, it adds all the elements of the iterable object to the set. As a string is an iterable object, you can use the update()
method to extract unique characters from a string.
To extract unique characters from a string using the update()
method, we will first create an empty set using the set()
function. Then, we will invoke the update()
method on the empty set and pass the string as an input argument to the update()
method. After execution, we will get a set containing all the unique characters of the string. You can observe this in the following example.
myStr="Python For Beginners"
print("The input string is:",myStr)
output=set()
output.update(myStr)
print("The output is:",output)
Output:
The input string is: Python For Beginners
The output is: {'F', 'e', 'y', 't', 'h', 'P', 'i', 'g', ' ', 'o', 's', 'r', 'n', 'B'}
You can also directly pass the input string to the set()
function to create a set of unique characters of the string as shown below.
myStr="Python For Beginners"
print("The input string is:",myStr)
output=set(myStr)
print("The output is:",output)
Output:
The input string is: Python For Beginners
The output is: {'F', 'e', 'y', 't', 'h', 'P', 'i', 'g', ' ', 'o', 's', 'r', 'n', 'B'}
Suggested Reading: Create Chat Application in Python
Unique Characters From String in Python Using Counter() Method
With all the unique characters, you can also find the frequency of the characters in a string. You can use the Counter function defined in the collections module for this task.
The Counter()
function takes an iterable object as its input argument and returns a Counter object. The Counter object contains all the unique elements of the iterable object and their frequencies.
To extract all the unique characters from a string with their frequency, we will pass the string as the input to the Counter()
method. After execution, we will get all the unique characters of the string with their frequency. You can observe this in the following example.
from collections import Counter
myStr="Python For Beginners"
print("The input string is:",myStr)
output=Counter(myStr)
print("The output is:",output)
Output:
The input string is: Python For Beginners
The output is: Counter({'n': 3, 'o': 2, ' ': 2, 'r': 2, 'e': 2, 'P': 1, 'y': 1, 't': 1, 'h': 1, 'F': 1, 'B': 1, 'g': 1, 'i': 1, 's': 1})
Conclusion
In this article, we have discussed different ways to extract unique characters from a string in Python.
To learn more about python programming, you can read this article on string manipulation. You might also like this article on python simplehttpserver.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Extract Unique Characters From String in Python appeared first on PythonForBeginners.com.
January 02, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
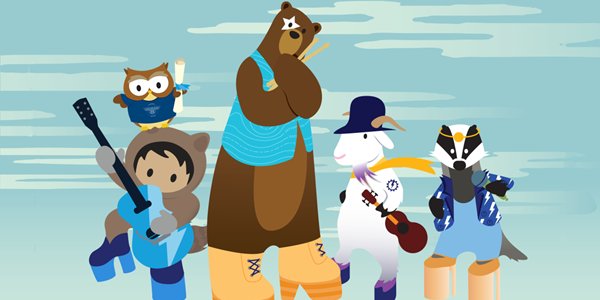
Post a Comment