Python for Beginners: Convert Epoch to Datetime in Python :
by:
blow post content copied from Planet Python
click here to view original post
Most of the software applications log date and time values as UNIX timestamps. While analyzing the logged data, we often need to convert the Unix timestamp to date and time values. In this article, we will discuss different ways to convert UNIX timestamps to datetime in python. We will also discuss how to convert a negative epoch to datetime in Python.
Unix Epoch to Datetime in Python
To convert epoch to datetime in python, we can use the fromtimestamp()
method defined in the datetime module. The fromtimestamp()
method takes the epoch as its input argument and returns the datetime object. You can observe this in the following example.
from datetime import datetime
epoch=123456789
print("The epoch is:")
print(epoch)
datetime_obj=datetime.fromtimestamp(epoch)
print("The datetime object is:")
print(datetime_obj)
Output:
The epoch is:
123456789
The datetime object is:
1973-11-30 03:03:09
In this example, we have converted the epoch 123456789 to datetime value using the fromtimestamp()
method.
The above approach will give you time according to the time zone on your machine. If you want to get the UTC time from the timestamp, you can use the utcfromtimestamp()
instead of the fromtimestamp()
method as shown below.
from datetime import datetime
epoch=123456789
print("The epoch is:")
print(epoch)
datetime_obj=datetime.utcfromtimestamp(epoch)
print("The datetime object is:")
print(datetime_obj)
Output:
The epoch is:
123456789
The datetime object is:
1973-11-29 21:33:09
In this example, you can observe that the datetime output show the time approx 5 hours and 30 minutes before the time in the previous example. However, we have used the same epoch value. This difference is due to the reason that the time zone of my computer is set to +5:30 hours.
Unix Timestamp to Datetime String
To convert the Unix timestamp to a datetime string in python, we will first create a datetime object from the epoch using the fromtimestamp()
method or the utcfromtimestamp()
method. Then, you can use the strftime()
method to convert the datetime object to a string.
The strftime()
method, when invoked on a datetime object, takes the format of the required datetime string as its input argument and returns the output string. You can observe this in the following example.
from datetime import datetime
epoch=123456789
print("The epoch is:")
print(epoch)
datetime_obj=datetime.utcfromtimestamp(epoch)
print("The datetime object is:")
print(datetime_obj)
datetime_string=datetime_obj.strftime( "%d-%m-%Y %H:%M:%S" )
print("The datetime string is:")
print(datetime_string)
Output:
The epoch is:
123456789
The datetime object is:
1973-11-29 21:33:09
The datetime string is:
29-11-1973 21:33:09
In this example, the format specifiers used in the strftime()
method are as follows.
- %d is the placeholder for date.
- %m is the placeholder for month.
- %Y is the placeholder for year.
- %H is the placeholder for hour.
- %M is the placeholder for minutes.
- %S is the placeholder for seconds.
You can also change the position of the placeholders in the string to change the date format.
Datetime to UNIX Timestamp in Python
To convert a datetime object to UNIX timestamp in python, we can use the timestamp()
method. The timestamp()
method, when invoked on a datetime object, returns the UNIX epoch for the given datetime object. You can observe this in the following example.
from datetime import datetime
datetime_obj=datetime.today()
print("The datetime object is:")
print(datetime_obj)
epoch=datetime_obj.timestamp()
print("The epoch is:")
print(epoch)
Output:
The datetime object is:
2023-01-24 00:34:40.582494
The epoch is:
1674500680.582494
In this example, we first obtained the current datetime using the datetime.today()
method. Then, we used the timestamp()
method to convert datetime object to timestamp.
Convert Negative Timestamp to Datetime in Python
The UNIX timestamp or epoch is basically the number of seconds that elapsed after UTC 1st January 1970, 0 hours, 0 minutes, 0 seconds. So, if we represent a date before 1970 using an epoch, the value is negative. For example, the if we represent 31 December 1969 using epoch, it will evaluate to -86400 i.e 24 hours*3600 seconds before 01 Jan 1970. You can observe this in the following example.
from datetime import datetime
epoch=-86400
print("The epoch is:")
print(epoch)
datetime_obj=datetime.fromtimestamp(epoch)
print("The datetime object is:")
print(datetime_obj)
Output:
The epoch is:
-86400
The datetime object is:
1969-12-31 05:30:00
To convert a negative UNIX timestamp to datetime, you can directly pass it to the fromtimestamp()
method as shown above. Here, we have specified the timestamp as -86400. Hence, the fromtimestamp()
method returns the datetime 86400 seconds before the datetime 01 Jan 1970 +5:30.
Again, the above approach will give you time according to the time zone on your machine. If you want to get the UTC time from the timestamp, you can use the utcfromtimestamp()
instead of the fromtimestamp()
method as shown below.
from datetime import datetime
epoch=-86400
print("The epoch is:")
print(epoch)
datetime_obj=datetime.utcfromtimestamp(epoch)
print("The datetime object is:")
print(datetime_obj)
Output:
The epoch is:
-86400
The datetime object is:
1969-12-31 00:00:00
In this example, you can observe that the utcfromtimestamp()
method returns the datetime 1969-12-31 00:00:00 which is exactly 86400 seconds before Jan 1, 1970 00:00:00.
Instead of using the above approach, you can also use the timedelta()
function to convert the negative epoch to datetime.
The timedelta()
function takes the negative epoch as its input argument and returns a timedelta object. After calculating the timedelta object, you can add it to the datetime object representing Jan 1, 1970. This will give you the datetime object for the negative epoch that was given as input. You can observe this in the following example.
from datetime import datetime
from datetime import timedelta
epoch=-86400
print("The epoch is:")
print(epoch)
datetime_obj=datetime(1970,1,1)+timedelta(seconds=epoch)
print("The datetime object is:")
print(datetime_obj)
Output:
The epoch is:
-86400
The datetime object is:
1969-12-31 00:00:00
Conclusion
In this article, we have discussed different ways to convert a UNIX timestamp to datetime in python. We also discussed how to convert a negative epoch to a datetime object in python.
To learn more about python programming, you can read this article on python simplehttpserver. You might also like this article on python with open statement.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Convert Epoch to Datetime in Python appeared first on PythonForBeginners.com.
January 25, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
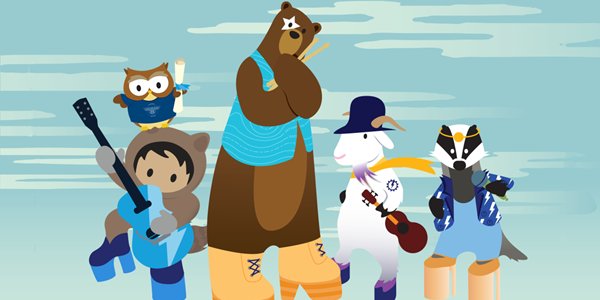
Post a Comment