Real Python: Python's "in" and "not in" Operators: Check for Membership :
by:
blow post content copied from Planet Python
click here to view original post
Python’s in
and not in
operators allow you to quickly determine if a given value is or isn’t part of a collection of values. This type of check is common in programming, and it’s generally known as a membership test in Python. Therefore, these operators are known as membership operators.
In this tutorial, you’ll learn how to:
- Perform membership tests using the
in
andnot in
operators - Use
in
andnot in
with different data types - Work with
operator.contains()
, the equivalent function to thein
operator - Provide support for
in
andnot in
in your own classes
To get the most out of this tutorial, you’ll need basic knowledge of Python, including built-in data types, such as lists, tuples, ranges, strings, sets, and dictionaries. You’ll also need to know about Python generators, comprehensions, and classes.
Source Code: Click here to download the free source code that you’ll use to perform membership tests in Python with in
and not in
.
Getting Started With Membership Tests in Python
Sometimes you need to find out whether a value is present in a collection of values or not. In other words, you need to check if a given value is or is not a member of a collection of values. This kind of check is commonly known as a membership test.
Arguably, the natural way to perform this kind of check is to iterate over the values and compare them with the target value. You can do this with the help of a for
loop and a conditional statement.
Consider the following is_member()
function:
>>> def is_member(value, iterable):
... for item in iterable:
... if value is item or value == item:
... return True
... return False
...
This function takes two arguments, the target value
and a collection of values, which is generically called iterable
. The loop iterates over iterable
while the conditional statement checks if the target value
is equal to the current value. Note that the condition checks for object identity with is
or for value equality with the equality operator (==
). These are slightly different but complementary tests.
If the condition is true, then the function returns True
, breaking out of the loop. This early return short-circuits the loop operation. If the loop finishes without any match, then the function returns False
:
>>> is_member(5, [2, 3, 5, 9, 7])
True
>>> is_member(8, [2, 3, 5, 9, 7])
False
The first call to is_member()
returns True
because the target value, 5
, is a member of the list at hand, [2, 3, 5, 9, 7]
. The second call to the function returns False
because 8
isn’t present in the input list of values.
Membership tests like the ones above are so common and useful in programming that Python has dedicated operators to perform these types of checks. You can get to know the membership operators in the following table:
Operator | Description | Syntax |
---|---|---|
in |
Returns True if the target value is present in a collection of values. Otherwise, it returns False . |
value in collection |
not in |
Returns True if the target value is not present in a given collection of values. Otherwise, it returns False . |
value not in collection |
As with Boolean operators, Python favors readability by using common English words instead of potentially confusing symbols as operators.
Note: Don’t confuse the in
keyword when it works as the membership operator with the in
keyword in the for
loop syntax. They have entirely different meanings. The in
operator checks if a value is in a collection of values, while the in
keyword in a for
loop indicates the iterable that you want to draw from.
Like many other operators, in
and not in
are binary operators. That means you can create expressions by connecting two operands. In this case, those are:
- Left operand: The target value that you want to look for in a collection of values
- Right operand: The collection of values where the target value may be found
The syntax of a membership test looks something like this:
value in collection
value not in collection
In these expressions, value
can be any Python object. Meanwhile, collection
can be any data type that can hold collections of values, including lists, tuples, strings, sets, and dictionaries. It can also be a class that implements the .__contains__()
method or a user-defined class that explicitly supports membership tests or iteration.
If you use the in
and not in
operators correctly, then the expressions that you build with them will always evaluate to a Boolean value. In other words, those expressions will always return either True
or False
. On the other hand, if you try and find a value in something that doesn’t support membership tests, then you’ll get a TypeError
. Later, you’ll learn more about the Python data types that support membership tests.
Because membership operators always evaluate to a Boolean value, Python considers them Boolean operators just like the and
, or
, and not
operators.
Read the full article at https://realpython.com/python-in-operator/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 19, 2022 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
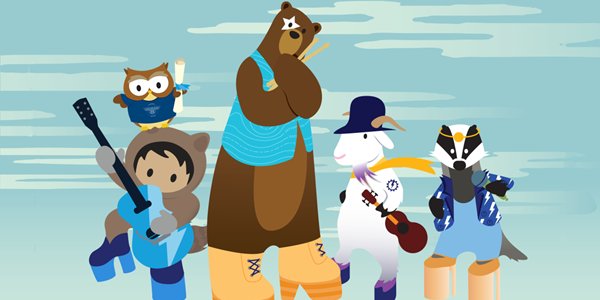
Post a Comment