Python | Split String then Join : Shubham Sayon
by: Shubham Sayon
blow post content copied from Finxter
click here to view original post
Summary: Use one of the following ways to split a string to get an element from a string:
- Using split
- Using list comprehension and join
- Using regex
Minimal Example
text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" li = [] for pair in text.split(','): li.append(pair.split('=')[1]) print(li) print('/'.join(li)) result = [pair.split('=')[1] for pair in text.split(',')] print(result) print('/'.join(result)) import re li = re.split(r",.*?=", text[3:]) res = "/".join(li) print(li) print(res) ''' ===============OUTPUT in each case======================== ['username', 'group1', 'group2', 'domain1', 'enterprise'] username/group1/group2/domain1/enterprise ========================================================== '''
Problem Formulation
Example
Challenge: Extract certain substrings from the given string and then combine the extracted substrings with a “/
” in between to create a new string. Thus, you first have to split the string accordingly and then join them to create a new string.
# Input text = "subdomain=blog, sld=finxter, tld=com" # Output ['blog', 'finxter', 'com'] blog/finxter/com
Method 1: Using a for Loop
Approach: Follow the explanation given below to understand how to solve the given problem.
- First split the given string using comma “,” as the delimiter and then use a for loop to iterate through each item of the list returned by the split method.
- Go ahead and further split each item into two parts such that the second element of this list will be the word that we actually need in the final string.
- Store each word required in another list and then combine them to create a string using the
join
method. Use “/” as the joining link/string between each element.
Code:
text = "subdomain=blog, sld=finxter, tld=com" li = [] for pair in text.split(','): li.append(pair.split('=')[1]) print(li) print('/'.join(li))
Output:
['blog', 'finxter', 'com'] blog/finxter/com
Method 2: Using List Comprehension and Join
You can formulate the above solution in a more compact way by using a list comprehension. Use the list comprehension to split the string twice and store the required substrings in a list. Finally, join the items of list returned by the list comprehension using “/” as the join string within the join()
method.
Code:
text = "subdomain=blog, sld=finxter, tld=com" result = [pair.split('=')[1] for pair in text.split(',')] print(result) print('/'.join(result))
Output:
['blog', 'finxter', 'com'] blog/finxter/com
Method 3: Using regex
- Yet another way to solve the problem is to use the
split
method of the regex module. The pattern,.*?=",
allows you to search and split all items that begin with a comma followed by any number of characters and then followed by an equals sign. In other words, you are simply storing the words that come between a comma and an equals sign in the given string. - Note that the second argument in the split method is an iterable and in this case, we are passing the string as the iterable such that we are considering the index from which the word “
blog
” starts. - Once you have the list containing the split strings, go ahead and join them using the join method with “/” as the joining string.
Code:
text = "subdomain=blog, sld=finxter, tld=com" import re li = re.split(r",.*?=", text[10:]) res = "/".join(li) print(li) print(res)
Output:
['blog', 'finxter', 'com'] blog/finxter/com
Recommended Read: Python Regex Sub
Do you want to master the regex superpower? Check out my new book The Smartest Way to Learn Regular Expressions in Python with the innovative 3-step approach for active learning: (1) study a book chapter, (2) solve a code puzzle, and (3) watch an educational chapter video.
Conclusion
Well! That brings us to the end of this tutorial. We solved the given problem with as many as three different ways. Feel free to opt the one that suits you.
I hope this article helped you. Please subscribe and stay tuned for more interesting tutorials and solutions.
Python Regex Course
Google engineers are regular expression masters. The Google search engine is a massive text-processing engine that extracts value from trillions of webpages.
Facebook engineers are regular expression masters. Social networks like Facebook, WhatsApp, and Instagram connect humans via text messages.
Amazon engineers are regular expression masters. Ecommerce giants ship products based on textual product descriptions. Regular expressions rule the game when text processing meets computer science.
If you want to become a regular expression master too, check out the most comprehensive Python regex course on the planet:
December 03, 2022 at 09:45PM
Click here for more details...
=============================
The original post is available in Finxter by Shubham Sayon
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
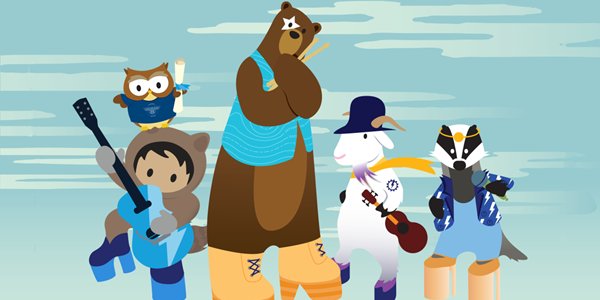
Post a Comment