Python | Split String Reverse : Shubham Sayon
by: Shubham Sayon
blow post content copied from Finxter
click here to view original post
Summary: There are three different ways to split the string and reverse it:
Using reversed() and join()
Using List Slicing
Using numpy.flip()
Minimal Example
# Given string text = "123,45,678" # Method 1: Using reversed() res = " ".join(reversed(text.split(","))) print(res) # Method 2: List slicing print(' '.join(text.split(',')[::-1])) # Method 3: Using numpy import numpy as np res = text.split(",") print(' '.join(list(np.flip(res)))) # OUTPUTS: 678 45 123
Problem Formulation
Problem: Given a string. How will you take a string, split it, reverse it and join it back together again using Python?
Example: Let’s visualize the problem with the help of an example:
input = "red black blue" output = blue black red
Now that we have an overview of our problem, let us dive into the solutions without further ado.
Method 1: Using split() and reversed()
Prerequisites
- Python’s built-in
reversed(sequence)
function returns a reverse iterator over the values of the given sequence, such as a list, a tuple, or a string. The return value of thereversed()
function is not a list but an iterator object for efficiency reasons. - The
split()
method splits the string at a given separator and returns a split list of substrings. It returns a list of the words in the string, usingsep
as the delimiter string.
Approach: You have to split the given string using space as a delimiter and use the reversed()
method to return a reverse value of the elements in the list. The join()
method is used to bind the elements together into a string. It concatenates the elements in an iterable.
Code:
text = "red black blue" res = " ".join(reversed(text.split(" "))) print(res) # blue black red
Related reads:
(i) Python reversed() — A Simple Guide with Video
(ii) Python String split()
(iii) Python String join()
Method 2: Using List Slicing
Prerequisite: Slicing is a concept to carve out a substring from a given string. Use slicing notation s[start:stop:step]
to access every step-th element starting from index start (included) and ending in index stop (excluded). All three arguments are optional, so you can skip them to use the default values (start = 0, stop = len(lst), step = 1
). For example, the expression s[2:4]
from string ‘hello
‘ carves out the slice ‘ll
‘, and the expression s[:3:2]
carves out the slice ‘hl
‘.
Approach: You must use the split()
method to split the string and get a list of substrings. In order to reverse the list, negative list slicing gets used in this case: s[::-1]
, i.e. the default indices do not start = 0
and end = len(s)
but the other way round: start = len(s)-1
and end = -1
. Here, the start index gets still included, and the end index gets still excluded from the slice. Because of that, the default end index is -1 and not 0. The join()
method gets used to concatenate the elements in an iterable.It binds the elements together into a string.
Code:
text = "red black blue" print(' '.join(text.split(' ')[::-1])) # blue black red
Related reads:
(i) Introduction to Slicing in Python
(ii) Python String join()
Method 3: Using numpy.flip()
Approach: numpy.flip()
is a function from the numpy library, which can be used to reverse the order of the elements. The method uses the numpy array along the specified axis, thereby preserving the shape of the array. You have to use the np.flip()
method on the list of split substrings to reverse the elements. Use the join()
method to bind the elements together in a string.
Code:
import numpy as np text = "red black blue" res = text.split(" ") print(' '.join(np.flip(res))) # blue black red
Do you want to become a NumPy master? Check out our interactive puzzle book Coffee Break NumPy and boost your data science skills! (Amazon link opens in new tab.)
Conclusion
Hurrah! We have successfully solved the given problem using as many as three different ways. I hope you enjoyed this article and it helps you in your Python coding journey. Please subscribe and stay tuned for more interesting articles!
December 04, 2022 at 07:30PM
Click here for more details...
=============================
The original post is available in Finxter by Shubham Sayon
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
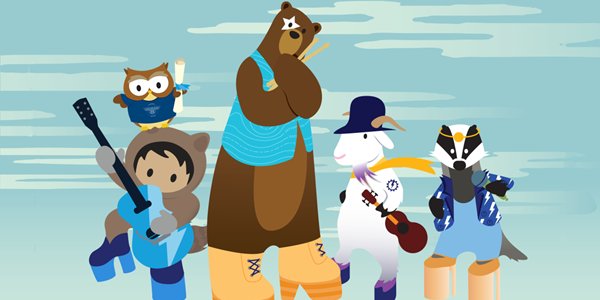
Post a Comment