Python | Split String Get Element : Shubham Sayon
by: Shubham Sayon
blow post content copied from Finxter
click here to view original post
Summary: Use of one of the following ways to split a string to get an element from a string.
- Using split
- Using list comprehension and join
- Using regex
Minimal Example
# Example 1 text = "Welcome to the world of Python!" # Solution res = text.split() print("Split substrings: ", res) print("Required Element: ", res[3]) # Alternate One line formulation (res, ele) = (text.split(), text.split()[3]) print(f'Split substrings: {res}\nRequired Element: {ele}') # Example 2 text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" # Method 1: Using a For Loop li = [] for pair in text.split(','): li.append(pair.split('=')[1]) print(li) print('/'.join(li)) # Method 2: Using List Comprehension and join result = [pair.split('=')[1] for pair in text.split(',')] print(result) print('/'.join(result)) # Method 3: Using regex import re text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" print(re.sub(",.*?=", "/", text[3:]))
Problem Formulation
Problem: Given a string. How will split the string and get the element you want from the split string?
Example 1
Extract the word “world
” from the given string.
# Input text = "Welcome to the world of Python!" # Output Split substrings: ['Welcome', 'to', 'the', 'world', 'of', 'Python!'] Required Element: world
Example 2
# Input text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" # Output ['username', 'group1', 'group2', 'domain1', 'enterprise'] username/group1/group2/domain1/enterprise
This is a more complex scenario wherein you have to extract certain substrings from the given string and then combine the extracted substrings with a “/
” in between to create a new string. Can you solve it?
Solution to Example 1
Method 1: Using split()
Approach: Split the string normally at the occurrence of a whitespace using the split method. To extract the required element from the list use its index within square brackets.
Code:
text = "Welcome to the world of Python!" res = text.split() print("Split substrings: ", res) print("Required Element: ", res[3])
Output:
Split substrings: ['Welcome', 'to', 'the', 'world', 'of', 'Python!'] Required Element: world
One-Liner
You can formulate the above solution in a single line. You can do so by simply packing the required list and the required string into two variables in a single line.
Code:
text = "Welcome to the world of Python!" (res, ele) = (text.split(), text.split()[3]) print(f'Split substrings: {res} \nRequired Element: {ele}')
Output:
Split substrings: ['Welcome', 'to', 'the', 'world', 'of', 'Python!'] Required Element: world
Solutions to Example 2
Method 1: Using a for Loop
Approach: Follow the explanation given below along with the illustrations to understand how to solve the given problem.

- First split the given string using comma “,” as the delimiter and then use a for loop to iterate through each item of the list returned by the split method.
- Go ahead and further split each item into two parts such that the second element of this list will be the word that we actually need in the final string. Store each word required in another list and then combine them to create a string using the
join
method. Use “/” as the joining link/string between each element.
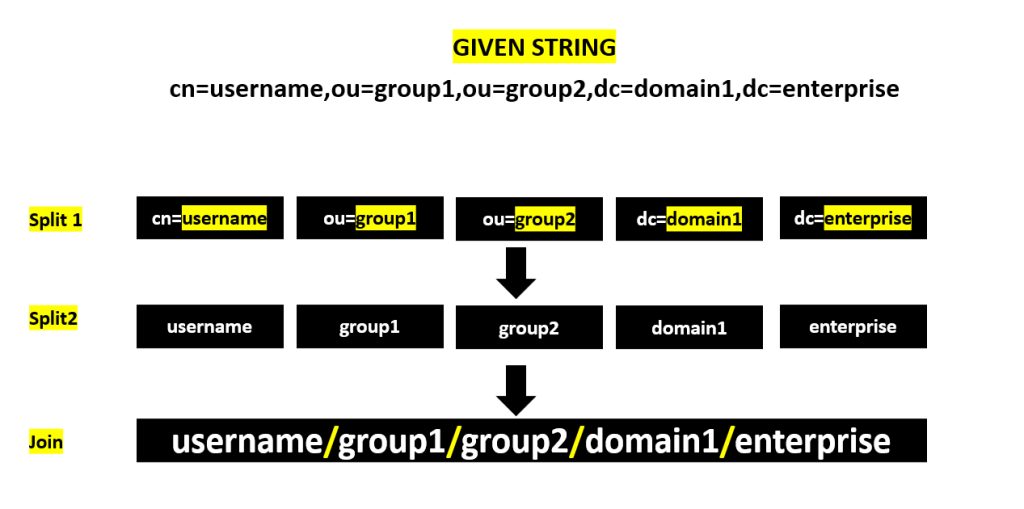
Code:
text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" # Method 1: Using a For Loop li = [] for pair in text.split(','): li.append(pair.split('=')[1]) print(li) print('/'.join(li))
Output:
['username', 'group1', 'group2', 'domain1', 'enterprise'] username/group1/group2/domain1/enterprise
Method 2: Using List Comprehension and Join
You can formulate the above solution in a more compact way by using a list comprehension. Use the list comprehension to split the string twice and store the required substrings in a list. Finally, join the items of list returned by the list comprehension using “/” as the join string within the join()
method.
Code:
result = [pair.split('=')[1] for pair in text.split(',')] print(result) print('/'.join(result))
Output:
['username', 'group1', 'group2', 'domain1', 'enterprise'] username/group1/group2/domain1/enterprise
Method 3: Using regex
Yet another way to solve the problem is to use the sub
method of the regex module. The pattern ,.*?=", "/
allows you to search all items that begin with a comma followed by any number of characters and then followed by an equals sign. Replace/substitute all the matches found with “/” character to deduce the final string.
Code:
import re text = "cn=username,ou=group1,ou=group2,dc=domain1,dc=enterprise" print(re.sub(",.*?=", "/", text[3:]))
Output:
username/group1/group2/domain1/enterprise
Recommended Read: Python Regex Sub
Conclusion
Well! That brings us to the end of this tutorial. We solved two different scenarios pertaining to the same problem. Feel free to opt the one that suits you.
I hope this article helped you. Please subscribe and stay tuned for more interesting articles.
Do you want to master the regex superpower? Check out my new book The Smartest Way to Learn Regular Expressions in Python with the innovative 3-step approach for active learning: (1) study a book chapter, (2) solve a code puzzle, and (3) watch an educational chapter video.
December 02, 2022 at 02:55PM
Click here for more details...
=============================
The original post is available in Finxter by Shubham Sayon
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
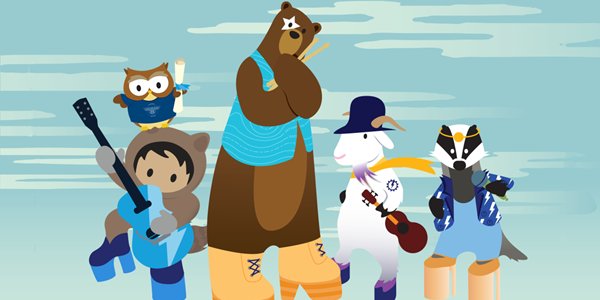
Post a Comment