How to Return a JSON Object From a Function in Python? : Stephen Schwaner
by: Stephen Schwaner
blow post content copied from Finxter
click here to view original post
Return a JSON String Object from the json.dumps() Method
The JSON (JavaScript Object Notation) format is widely used for transmitting structured data, especially between web applications. Fortunately, Python has several libraries for dealing with this data format, including the json
module.
The JSON format can nest data within “object literals” denoted by curly braces. Like Python dictionaries, JSON object literals store data in key-value pairs. Let’s look at an example of converting a Python dictionary to a JSON string object using the json.dumps()
method.
In the example below, we will:
- Import the
json
module - Define a dictionary of
greetings
- Convert (serialize) the dictionary to a JSON string
- Print the JSON string
# import library import json # define dictionary greetings = {"spanish": "hola", "english": "hello", "french": "bonjour"} # convert (serialize) dictionary to JSON string object json_string = json.dumps(greetings) # print result print(json_string)
Result:
{"spanish": "hola", "english": "hello", "french": "bonjour"}
The JSON format can also store data within arrays, denoted by square brackets. JSON arrays are analogous to Python lists.
Let’s look at an example of how to convert a Python array to a JSON string object using json.dumps()
:
# import library import json # define list favorite_foods = ["steak", "lasagne", "collards", "poppy seed muffins"] # convert (serialize) list to JSON string object json_string = json.dumps(favorite_foods) # print result print(json_string)
Result:
["steak", "lasagne", "collards", "poppy seed muffins"]
Like Python, the JSON format allows object literals (dictionaries) and arrays (lists) to be nested within each other:
# import library import json # define dictionary nested_dict = {"spanish": "hola", "english": ["hi", "hello"], "french": "bonjour", "another_dict": {"key": "Wow! A nested dictionary!"}} # convert (serialize) dictionary to JSON string json_string = json.dumps(json_string) # print result print(json_string)
Result:
{"spanish": "hola", "english": ["hi", "hello"], "french": "bonjour", "another_dict": {"key": "Wow! A nested dictionary!"}}
Method Details json.dumps()
One benefit of the JSON format is that it is human-readable. To make its nested structure more obvious to the human eye, the indent
option of json.dumps()
can be utilized.
Let’s write a JSON string object to a file after using the indent
option of the json.dumps()
method. Below, we will specify that we want json.dumps()
to use 2 spaces when indenting:
import json # define dictionary my_dict = {"greetings": {"english": ["hi", "hello"], "spanish": "hola"}, "fruit": ["apples", "pears", "grapefruit"], "integer": 8, "floating": 8.1234, "string": "yarn", "function?": "Nope!", "class?": "Nope!"} # convert (serialize) list to JSON string json_string = json.dumps(my_dict, indent=2) # write JSON string to my_file.JSON with open("my_file.JSON", "w") as file: file.write(json_string)
Resulting file my_file.JSON
:
{
"greetings": {
"english": [
"hi",
"hello"
],
"spanish": "hola"
},
"fruit": [
"apples",
"pears",
"grapefruit"
],
"integer": 8,
"floating": 8.1234,
"string": "yarn",
"function?": "Nope!",
"class?": "Nope!"
}
The previous example also illustrates the python data types that can be JSON serialized by the json.dumps()
method:
- Dictionaries
- Lists
- Integers
- floating point numbers
- Strings
If you try to include a function or class within the list or dictionary that gets passed into the json.dumps()
method, Python will raise an error similar to, “Object of type function is not JSON serializable.
”
Packages such as the jsonpickle
module can be used to serialize more complex datatypes like functions and classes.
Recommended Tutorial: An Introduction to Classes in Python
Return a JSON String From a Web Application Using Flask
Below is another example that utilizes the Python module flask
. The flask
module is used for developing web applications. Let’s say you would like to set up a web page that returns a JSON string containing your favorite books by genre.
To do so, we will:
- Import the
flask
module - Define a
Flask
instance - Define the URL that will return our favorite books
- Map the function that will return our favorite books to the URL
- Use the
jsonify()
method to return a JSON string object
from flask import Flask, jsonify # define Flask instance app = Flask(__name__) # Define URL @app.route('/books') # Map function to URL and define dictionary def return_json(): my_dict = {"Mystery": ["The Hound of the Baskervilles", "And Then There Were None"], "Science Fiction": ["A Canticle for Leibowitz", "That Hideous Strength", "Speaker for the Dead"], "Fantasy": ["The Way of Kings", "Mistborn"], "Biography": "Open"} return jsonify(my_dict) if __name__ == '__main__': app.run()
Run the previous code and open up a web browser. Use the web browser to navigate to http://127.0.0.1:5000/books
.
The result will be:
{"Biography":"Open","Fantasy":["The Way of Kings","Mistborn"],"Mystery":["The Hound of the Baskervilles","And Then There Were None"],"Science Fiction":["A Canticle for Leibowitz","That Hideous Strength","Speaker for the Dead"]}
Receive a JSON Response Using Python Requests
In our last example, we will use the Python requests
module to get real-time data from a website in JSON format.
The requests
module is a simple way to interact with web APIs. It allows the user to make requests to a web API including GET, POST, OPTIONS, HEAD, PUT, PATCH, and DELETE requests. These can all be made using the requests.request()
method. The method also includes keyword arguments for accomplishing tasks such as sending data in the request body or handling authentication.
In the example below, it will suddenly become very important for us to know exactly how many humans are in outer space and what spacecraft they are on. Luckily, http://api.open-notify.org/ has been created to fulfill that need. The steps are quite simple:
- Import the
requests
andjson
modules - Make a GET request using
requests.request()
- Print the returned JSON data
- Print the JSON data to a file with some help from json.dumps()
import requests import json # Make the GET request response = requests.request("GET", "http://api.open-notify.org/astros.json") if response: # print the JSON string containing astronaut names print(f"JSON content is {response.json()}") # write the JSON string to a file with open("astros.JSON", "w") as f: f.write(json.dumps(response.json())) else: print("womp womp")
Interestingly, the output of the response.json()
method is a python dictionary, which cannot be written to a file. As in our first example, the json.dumps()
method comes in handy for serializing this dictionary into a JSON string.
Conclusion
The JSON data format is widely used for passing information to and from web applications. Now that you have read this tutorial, you know how to:
- Return a JSON string object by serializing a python data container using
json.dumps()
- Write a pretty JSON file using the
indent
keyword of thejson.dumps()
method - Create a web application that returns a JSON string object upon receiving a GET request
- Request JSON data from a web application
Enjoy!
December 17, 2022 at 03:29PM
Click here for more details...
=============================
The original post is available in Finxter by Stephen Schwaner
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
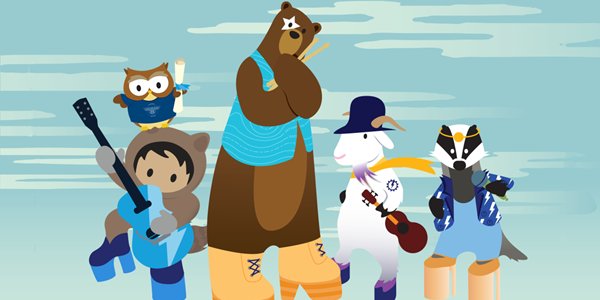
Post a Comment