What's Lazy Evaluation in Python? :
by:
blow post content copied from Real Python
click here to view original post
Being lazy is not always a bad thing. Every line of code you write has at least one expression that Python needs to evaluate. Python lazy evaluation is when Python takes the lazy option and delays working out the value returned by an expression until that value is needed.
An expression in Python is a unit of code that evaluates to a value. Examples of expressions include object names, function calls, expressions with arithmetic operators, literals that create built-in object types such as lists, and more. However, not all statements are expressions. For example, if
statements and for
loop statements don’t return a value.
Python needs to evaluate every expression it encounters to use its value. In this tutorial, you’ll learn about the different ways Python evaluates these expressions. You’ll understand why some expressions are evaluated immediately, while others are evaluated later in the program’s execution. So, what’s lazy evaluation in Python?
Get Your Code: Click here to download the free sample code that shows you how to use lazy evaluation in Python.
Take the Quiz: Test your knowledge with our interactive “What's Lazy Evaluation in Python?” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
What's Lazy Evaluation in Python?
In this quiz, you'll test your understanding of the differences between lazy and eager evaluation in Python. By working through this quiz, you'll revisit how Python optimizes memory use and computational overhead by deciding when to compute values.
In Short: Python Lazy Evaluation Generates Objects Only When Needed
An expression evaluates to a value. However, you can separate the type of evaluation of expressions into two types:
- Eager evaluation
- Lazy evaluation
Eager evaluation refers to those cases when Python evaluates an expression as soon as it encounters it. Here are some examples of expressions that are evaluated eagerly:
1>>> 5 + 10
215
3
4>>> import random
5>>> random.randint(1, 10)
64
7
8>>> [2, 4, 6, 8, 10]
9[2, 4, 6, 8, 10]
10>>> numbers = [2, 4, 6, 8, 10]
11>>> numbers
12[2, 4, 6, 8, 10]
Interactive environments, such as the standard Python REPL used in this example, display the value of an expression when the line only contains the expression. This code section shows a few examples of statements and expressions:
- Lines 1 and 2: The first example includes the addition operator
+
, which Python evaluates as soon as it encounters it. The REPL shows the value15
. - Lines 4 to 6: The second example includes two lines:
- The import statement includes the keyword
import
followed by the name of a module. The module namerandom
is evaluated eagerly. - The function call
random.randint()
is evaluated eagerly, and its value is returned immediately. All standard functions are evaluated eagerly. You’ll learn about generator functions later, which behave differently.
- The import statement includes the keyword
- Lines 8 to 12: The final example has three lines of code:
- The literal to create a list is an expression that’s evaluated eagerly. This expression contains several integer literals, which are themselves expressions evaluated immediately.
- The assignment statement assigns the object created by the list literal to the name
numbers
. This statement is not an expression and doesn’t return a value. However, it includes the list literal on the right-hand side, which is an expression that’s evaluated eagerly. - The final line contains the name
numbers
, which is eagerly evaluated to return the list object.
The list you create in the final example is created in full when you define it. Python needs to allocate memory for the list and all its elements. This memory won’t be freed as long as this list exists in your program. The memory allocation in this example is small and won’t impact the program. However, larger objects require more memory, which can cause performance issues.
Lazy evaluation refers to cases when Python doesn’t work out the values of an expression immediately. Instead, the values are returned at the point when they’re required in the program. Lazy evaluation can also be referred to as call-by-need.
This delay of when the program evaluates an expression delays the use of resources to create the value, which can improve the performance of a program by spreading the time-consuming process across a longer time period. It also prevents values that will not be used in the program from being generated. This can occur when the program terminates or moves to another part of its execution before all the generated values are used.
When large datasets are created using lazily-evaluated expressions, the program doesn’t need to use memory to store the data structure’s contents. The values are only generated when they’re needed.
An example of lazy evaluation occurs within the for
loop when you iterate using range()
:
for index in range(1, 1_000_001):
print(f"This is iteration {index}")
The built-in range()
is the constructor for Python’s range
object. The range
object does not store all of the one million integers it represents. Instead, the for
loop creates a range_iterator
from the range
object, which generates the next number in the sequence when it’s needed. Therefore, the program never needs to have all the values stored in memory at the same time.
Lazy evaluation also allows you to create infinite data structures, such as a live stream of audio or video data that continuously updates with new information, since the program doesn’t need to store all the values in memory at the same time. Infinite data structures are not possible with eager evaluation since they can’t be stored in memory.
There are disadvantages to deferred evaluation. Any errors raised by an expression are also deferred to a later point in the program. This delay can make debugging harder.
The lazy evaluation of the integers represented by range()
in a for
loop is one example of lazy evaluation. You’ll learn about more examples in the following section of this tutorial.
What Are Examples of Lazy Evaluation in Python?
In the previous section, you learned about using range()
in a for
loop, which leads to lazy evaluation of the integers represented by the range
object. There are other expressions in Python that lead to lazy evaluation. In this section, you’ll explore the main ones.
Other Built-In Data Types
Read the full article at https://realpython.com/python-lazy-evaluation/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
April 24, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
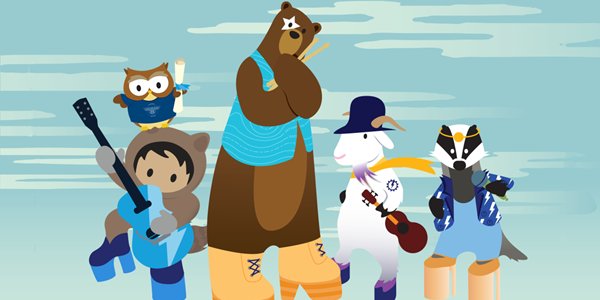
Post a Comment