How to Format Floats Within F-Strings in Python
by:
blow post content copied from Real Python
click here to view original post
You’ll often need to format and round a Python float
to display the results of your calculations neatly within strings. In earlier versions of Python, this was a messy thing to do because you needed to round your numbers first and then use either string concatenation or the old string formatting technique to do this for you.
Since Python 3.6, the literal string interpolation, more commonly known as a formatted string literal or f-string, allows you to customize the content of your strings in a more readable way.
An f-string is a literal string prefixed with a lowercase or uppercase letter f
and contains zero or more replacement fields enclosed within a pair of curly braces {...}
. Each field contains an expression that produces a value. You can calculate the field’s content, but you can also use function calls or even variables.
While most strings have a constant value, f-strings are evaluated at runtime. This makes it possible for you to pass different data into the replacement fields of the same f-string and produce different output. This extensibility of f-strings makes them a great way to embed dynamic content neatly inside strings. However, even though f-strings have largely replaced the earlier methods, they do have their short-comings.
For example, one of the most common attacks performed on a relational database is a SQL injection attack. Often, users provide parameters to SQL queries, and if the query is formed within an f-string, it may be possible to damage a database by passing in rogue commands. F-strings can also be used in a denial-of-service attack by attacking Python’s logging module code.
In older versions of Python, f-strings had a number of other limitations that were only fixed with Python version 3.12. This version is used throughout this tutorial.
Take a look at the example below. It shows you how to embed the result of a calculation within an f-string:
>>> f"One third, expressed as a float is: {1 / 3}"
'One third, expressed as a float is: 0.3333333333333333'
Without any explicit rounding, once an expression has produced its value it’ll be inserted into the string using a default number of decimal places. Here, the result is shown to sixteen decimal places, which, in most cases, is more precision than you’ll ever need. So you’ll likely want to round your final answer to a more practical number of digits.
In this tutorial, you’ll learn how to use a Python f-string to format floats to meet your requirements.
Get Your Code: Click here to download the free sample code and exercise solutions you’ll use for learning how to format floats within f-strings in Python.
Take the Quiz: Test your knowledge with our interactive “Format Floats Within F-Strings” quiz. Upon completion you will receive a score so you can track your learning progress over time:
Interactive Quiz
Format Floats Within F-Strings
In this quiz, you'll test your understanding of how to format floats within f-strings in Python. This knowledge will let you control the precision and appearance of floating-point numbers when you incorporate them into formatted strings.
How to Format and Round a Float Within a Python F-String
To format a float
for neat display within a Python f-string, you can use a format specifier. In its most basic form, this allows you to define the precision, or number of decimal places, the float
will be displayed with.
The code below displays the same calculation as before, only it’s displayed more neatly:
>>> f"One third, rounded to two decimal places is: {1 / 3:.2f}"
'One third, rounded to two decimal places is: 0.33'
To use Python’s format specifiers in a replacement field, you separate them from the expression with a colon (:
). As you can see, your float
has been rounded to two decimal places. You achieved this by adding the format specifier .2f
into the replacement field. The 2
is the precision, while the lowercase f
is an example of a presentation type. You’ll see more of these later.
Note: When you use a format specifier, you don’t actually change the underlying number. You only improve its display.
Python’s f-strings also have their own mini-language that allows you to format your output in a variety of different ways. Although this tutorial will focus on rounding, this is certainly not the only thing you can use them for. As you’ll see later, their mini-language is also used in other string formatting techniques.
In addition to displaying the result of calculations, the precision part of a format specifier can also be applied directly to variables and the return values of function calls:
>>> def total_price(cost):
... return cost * 1.2
...
>>> cost_price = 1000
>>> tax = 0.2
>>> f"£{1000:,.2f} + £{cost_price * tax:,.2f} = £{total_price(cost_price):,.2f}"
'£1,000.00 + £200.00 = £1,200.00'
This time, you’ve used multiple replacement fields in the same string. The first one formats a literal number, the second formats the result of a calculation, while the third formats the return value from a function call. Also, by inserting a comma (,
) before the decimal point (.
) in the format specifier, you add a thousands separator to your final output.
In everyday use, you display numbers with a fixed amount of decimals, but when performing scientific or engineering calculations, you may prefer to format them using significant figures. Your results are then assumed to be accurate to the number of significant figures you display them with.
If you want to round numbers to significant figures, you use the lowercase letter g
in the format specifier. You can also use an uppercase G
, but this automatically switches the format to scientific notation for large numbers.
Suppose you have a circle with a radius of 10.203 meters. To work out the area, you could use this code:
Read the full article at https://realpython.com/how-to-python-f-string-format-float/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
April 17, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
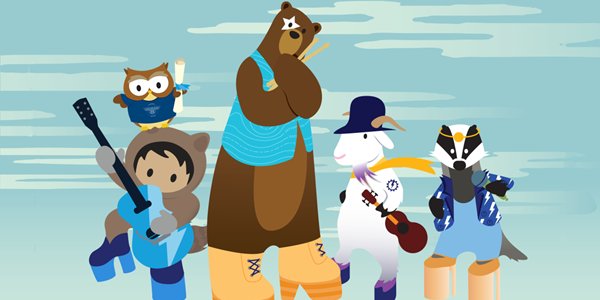
Post a Comment