Python String Formatting: Available Tools and Their Features
by:
blow post content copied from Real Python
click here to view original post
String formatting is the process of applying a proper format to a given value while using this value to create a new string through interpolation. Python has several tools for string interpolation that support many formatting features. In modern Python, you’ll use f-strings or the .format()
method most of the time. However, you’ll see the modulo operator (%
) being used in legacy code.
In this tutorial, you’ll learn how to:
- Format strings using f-strings for eager interpolation
- Use the
.format()
method to format your strings lazily - Work with the modulo operator (
%
) for string formatting - Decide which interpolation and formatting tool to use
To get the most out of this tutorial, you should be familiar with Python’s string data type and the available string interpolation tools. Having a basic knowledge of the string formatting mini-language is also a plus.
Get Your Code: Click here to download the free sample code you’ll use to learn about Python’s string formatting tools.
Take the Quiz: Test your knowledge with our interactive “Python String Formatting: Available Tools and Their Features” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python String Formatting: Available Tools and Their FeaturesTake this quiz to test your understanding of the available tools for string formatting in Python, as well as their strengths and weaknesses. These tools include f-strings, the .format() method, and the modulo operator.
Interpolating and Formatting Strings in Python
String interpolation involves generating strings by inserting other strings or objects into specific places in a base string or template. For example, here’s how you can do some string interpolation using an f-string:
>>> name = "Bob"
>>> f"Hello, {name}!"
'Hello, Bob!'
In this quick example, you first have a Python variable containing a string object, "Bob"
. Then, you create a new string using an f-string. In this string, you insert the content of your name
variable using a replacement field. When you run this last line of code, Python builds a final string, 'Hello, Bob!'
. The insertion of name
into the f-string is an interpolation.
Note: To dive deeper into string interpolation, check out the String Interpolation in Python: Exploring Available Tools tutorial.
When you do string interpolation, you may need to format the interpolated values to produce a well-formatted final string. To do this, you can use different string interpolation tools that support string formatting. In Python, you have these three tools:
- F-strings
- The
str.format()
method - The modulo operator (
%
)
The first two tools support the string formatting mini-language, a feature that allows you to fine-tune your strings. The third tool is a bit old and has fewer formatting options. However, you can use it to do some minimal formatting.
Note: The built-in format()
function is yet another tool that supports the format specification mini-language. This function is typically used for date and number formatting, but you won’t cover it in this tutorial.
In the following sections, you’ll start by learning a bit about the string formatting mini-language. Then, you’ll dive into using this language, f-strings, and the .format()
method to format your strings. Finally, you’ll learn about the formatting capabilities of the modulo operator.
Using F-Strings to Format Strings
Python 3.6 added a string interpolation and formatting tool called formatted string literals, or f-strings for short. As you’ve already learned, f-strings let you embed Python objects and expressions inside your strings. To create an f-string, you must prefix the string with an f
or F
and insert replacement fields in the string literal. Each replacement field must contain a variable, object, or expression:
>>> f"The number is {42}"
'The number is 42'
>>> a = 5
>>> b = 10
>>> f"{a} plus {b} is {a + b}"
'5 plus 10 is 15'
In the first example, you define an f-string that embeds the number 42
directly into the resulting string. In the second example, you insert two variables and an expression into the string.
Formatted string literals are a Python parser feature that converts f-strings into a series of string constants and expressions. These are then joined up to build the final string.
Using the Formatting Mini-Language With F-Strings
When you use f-strings to create strings through interpolation, you need to use replacement fields. In f-strings, you can define a replacement field using curly brackets ({}
) as in the examples below:
>>> debit = 300.00
>>> credit = 450.00
>>> f"Debit: ${debit}, Credit: ${credit}, Balance: ${credit - debit}"
'Debit: $300, Credit: $450.0, Balance: $150.0'
Inside the brackets, you can insert Python objects and expressions. In this example, you’d like the resulting string to display the currency values using a proper format. However, you get a string that shows the currency values with at most one digit on its decimal part.
Read the full article at https://realpython.com/python-string-formatting/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 05, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
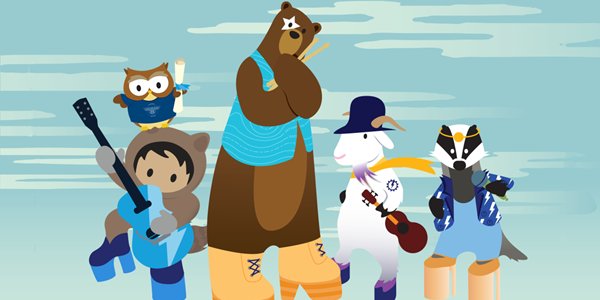
Post a Comment