5 Best Ways to Program to Find Longest Even Value Path of a Binary Tree in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: The challenge is to devise a program that can traverse a binary tree and find the length of the longest path consisting entirely of nodes with even integer values. Given a binary tree, where each node contains an integer, the goal is to compute the maximum length of any descending path where consecutive nodes have even values. For example, if the input binary tree has an even-valued path of length 4, the output should reflect that length.
Method 1: Depth-First Search (DFS) Recursive Approach
This method employs a depth-first search strategy, using recursion to navigate through the tree while tracking the longest sequence of even-valued nodes. The function takes a node and the current length of the path then returns the maximum path length found.
Here’s an example:
class TreeNode: def __init__(self, value=0, left=None, right=None): self.val = value self.left = left self.right = right def longest_even_value_path(root): def dfs(node, length): if not node: return length length = 0 if node.val % 2 else length + 1 return max(dfs(node.left, length), dfs(node.right, length)) return dfs(root, 0) # Example Usage root = TreeNode(2, TreeNode(3, TreeNode(4), TreeNode(6)), TreeNode(2, None, TreeNode(8))) print(longest_even_value_path(root))
Output:
2
The example defines a binary tree with an even-valued path of length 2. The longest_even_value_path
function calculates this by using a nested dfs
function, which checks for node value evenness and recurses down to children, thereby finding the maximum path length.
Method 2: Iterative DFS Using Stack
In this iterative approach, a stack is used to emulate the call stack of a recursive function. Each element in the stack contains a node and the current even value path length up to that node. We iterate until the stack is empty, updating the maximum length as we encounter even-valued nodes.
Here’s an example:
def longest_even_value_path_iterative(root): max_length, stack = 0, [(root, 0)] while stack: node, length = stack.pop() if node: length = 0 if node.val % 2 else length + 1 max_length = max(max_length, length) stack.append((node.left, length)) stack.append((node.right, length)) return max_length # Example usage is the same as in Method 1
Output:
2
This code snippet demonstrates an iterative depth-first search that stores the nodes along with their current even value path lengths in a stack. Each node is processed to update the maximum found length, using an iterative instead of a recursive approach.
Method 3: Breadth-First Search (BFS) Using Queue
Unlike DFS, Breadth-First Search uses a queue to explore nodes level by level. It also maintains the even-valued path length. BFS might be slower due to additional space required but can be more intuitive for some use-cases where the even paths are broad rather than deep.
Here’s an example:
from collections import deque def longest_even_value_path_bfs(root): if not root: return 0 max_length = 0 queue = deque([(root, 0)]) while queue: node, length = queue.popleft() if node: length = 0 if node.val % 2 else length + 1 max_length = max(max_length, length) queue.append((node.left, length)) queue.append((node.right, length)) return max_length # Example usage is the same as in Method 1
Output:
2
By using a queue, this function incrementally processes each level of the binary tree, carrying forth the length of the path on even-valued nodes and updating the running maximum length.
Method 4: Dynamic Programming on Trees
This method applies a dynamic programming approach where each node stores the length of the longest even value path below it. Starting from the leaves and moving up to the root, each node updates its value based on the maximum of its children’s even paths plus one if it’s even.
Here’s an example:
def longest_even_value_path_dp(root): def dp(node): if not node: return 0 left = dp(node.left) if node.val % 2 == 0 else 0 right = dp(node.right) if node.val % 2 == 0 else 0 return max(left, right) + 1 if node.val % 2 == 0 else 0 return dp(root) # Example usage is the same as in Method 1
Output:
2
The dynamic programming method solves subproblems at each node: the longest even value path in its left and right subtrees. It combines these solutions to form a larger solution, avoiding redundant computations.
Bonus One-Liner Method 5: Using Generators
A more Pythonic approach might involve a generator to yield even value path lengths from the binary tree. This method is concise but might be harder to read for those unfamiliar with Python generators.
Here’s an example:
def longest_even_value_path_gen(root): even_val_gen = (length for length in dfs_gen(root, 0) if length % 2 == 0) return max(even_val_gen, default=0) def dfs_gen(node, length): if not node: yield length else: length = length + 1 if node.val % 2 == 0 else 0 yield from dfs_gen(node.left, length) yield from dfs_gen(node.right, length) # Example usage is the same as in Method 1
Output:
2
This one-liner takes advantage of Python generators, which yield even value path lengths from the binary tree, then calculate the maximum.
Summary/Discussion
- Method 1: Depth-First Search (DFS) Recursive Approach. Strengths: Simple, elegant recursive solution. Weaknesses: Stack overflow risk for deep trees, less performant for large trees.
- Method 2: Iterative DFS Using Stack. Strengths: No risk of stack overflow, suitable for large depths. Weaknesses: Less intuitive than recursive solutions.
- Method 3: Breadth-First Search (BFS) Using Queue. Strengths: Intuitive level-by-level approach. Weaknesses: Requires additional space, may take longer for very deep and sparse trees.
- Method 4: Dynamic Programming on Trees. Strengths: Avoids redundant calculations, efficient for compute-intensive tasks. Weaknesses: More complex, potentially more difficult to debug.
- Method 5: Using Generators. Strengths: Concise, Pythonic. Weaknesses: Reduced readability for those not familiar with generators, can be tricky to implement for more complex requirements.
March 10, 2024 at 11:52PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
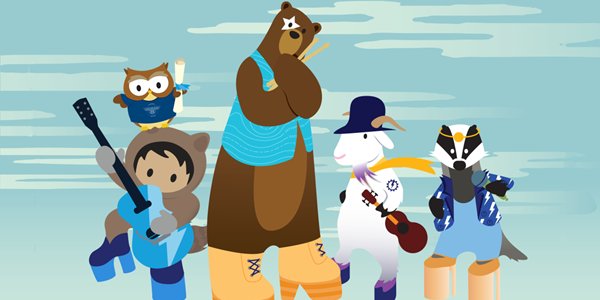
Post a Comment