5 Best Ways to Implement a Queue in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Imagine you need a structure to manage objects in a first-in, first-out (FIFO) order. You want to be able to add items to the back of the queue and remove items from the front, much like customers waiting in line at a store. This article presents five different ways to implement this queue structure using Python, paying attention to simplicity, performance, and Pythonic practices.
Method 1: Using a List
This method involves using a regular Python list to implement the queue. With a list, elements can simply be appended to the end using append()
and popped from the beginning using pop(0)
. This approach is simple and intuitive but not the most efficient due to the high cost of popping elements from the beginning of a list, which is an O(n) operation.
Here’s an example:
queue = [] def enqueue(queue, item): queue.append(item) def dequeue(queue): if len(queue) == 0: raise IndexError("dequeue from an empty queue") return queue.pop(0) # Sample usage enqueue(queue, "customer1") enqueue(queue, "customer2") print(dequeue(queue)) print(dequeue(queue))
The output of this code snippet would be:
customer1 customer2
This code snippet demonstrates the basic operations of a queue: adding items to the rear end and removing them from the front. However, the pop(0)
operation is inefficient because it shifts all the other elements one space to the left.
Method 2: Using collections.deque
The deque
(double-ended queue) from the collections
module is a high-performance object that supports adding and removing elements from both ends in O(1) time. This feature makes it perfect for implementing an efficient queue in Python. The append()
method can be used to add items to the rear, and popleft()
can be used to remove them from the front.
Here’s an example:
from collections import deque queue = deque() # Enqueue items queue.append('customer1') queue.append('customer2') # Dequeue items print(queue.popleft()) print(queue.popleft())
The output of this code snippet would be:
customer1 customer2
In this snippet, we use deque
to create a more efficient queue because popleft()
removes elements from the start of the queue without incurring the O(n) cost associated with shifting elements, as is the case with a list.
Method 3: Using queue.Queue
The Queue
class from the queue
module is designed for multi-threaded environments but can also be used in a single-threaded context. This class provides thread-safe FIFO queue implementation with built-in locking, but this adds overhead which can be unnecessary in a single-threaded scenario.
Here’s an example:
from queue import Queue queue = Queue() # Enqueue items queue.put('customer1') queue.put('customer2') # Dequeue items print(queue.get()) print(queue.get())
The output of this code snippet would be:
customer1 customer2
This code snippet uses the Queue
class where put()
and get()
serve the enqueue and dequeue operations, respectively. While this implementation is ideal for thread safety, it may be overkill for simpler, single-threaded scenarios.
Method 4: Using a Class with Two Stacks
Implementing a queue using two stacks is an alternative that involves two lists where one serves as the main container for elements, and the other is used for dequeuing operations. New elements are pushed onto the main stack, and when an element needs to be dequeued, elements are transferred to the dequeue stack to access the bottom element, which represents the front of the queue.
Here’s an example:
class Queue: def __init__(self): self.enqueue_stack = [] self.dequeue_stack = [] def enqueue(self, item): self.enqueue_stack.append(item) def dequeue(self): if not self.dequeue_stack: while self.enqueue_stack: self.dequeue_stack.append(self.enqueue_stack.pop()) if not self.dequeue_stack: raise IndexError("dequeue from an empty queue") return self.dequeue_stack.pop() # Sample usage queue = Queue() queue.enqueue('customer1') queue.enqueue('customer2') print(queue.dequeue()) print(queue.dequeue())
The output of this code snippet would be:
customer1 customer2
This code snippet shows a queue implemented with two stacks. While this method preserves the FIFO order and has an average time complexity of O(1), it can be complex and counterintuitive compared to other methods.
Bonus One-Liner Method 5: Using a List Comprehension
While not recommended for real-world applications due to inefficiency, it’s possible to implement a queue with a one-liner list comprehension by treating the list as immutable and constructing a new list with each operation.
Here’s an example:
queue = [] enqueue = lambda item: queue.append(item) dequeue = lambda: queue.pop(0) if queue else None # Sample usage enqueue('customer1') enqueue('customer2') print(dequeue()) print(dequeue())
The output of this code snippet would be:
customer1 customer2
While this one-liner uses Python lambda functions for brevity and appends and pops elements from the list to imitate queue behavior, it is the least efficient of the methods provided due to the O(n) time complexity for each dequeue operation.
Summary/Discussion
- Method 1: Using a List. Simple to understand and implement. Poor performance for large datasets due to O(n) operations.
- Method 2: Using collections.deque. Optimal method for queue operations in most situations, with O(1) operations on both ends of the queue.
- Method 3: Using queue.Queue. Thread-safe, suitable for multi-threaded environments. Overhead from locking makes it inefficient for single-threaded applications.
- Method 4: Using a Class with Two Stacks. Preserves FIFO order with average O(1) time complexity, though can be more complex than other methods.
- Bonus Method 5: Using a List Comprehension. A neat one-liner but highly inefficient for dequeue operations and not recommended for practical use.
March 08, 2024 at 01:54AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
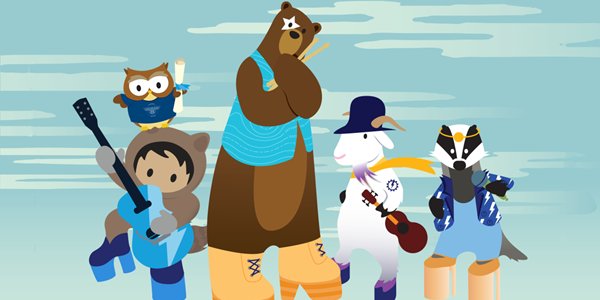
Post a Comment