5 Best Ways to Find Strings of the Same Size in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Imagine you are given a collection of strings and need to identify groups of strings that have the same length. For example, given the list
["hello", "world", "python", "code", "AI"]
, the desired output would be a new list containing [["hello", "world"], ["python"], ["code", "AI"]]
, since “hello” and “world” have 5 characters, “python” has 6, and “code” and “AI” have 4 and 2 characters respectively.
Method 1: Using defaultdict from Collections
This method utilizes the collections.defaultdict
to group strings by their length. It is efficient as it avoids manual checks for key existence and automatically initializes a list for each new key (the length of a string).
Here’s an example:
from collections import defaultdict def group_by_length(words): length_dict = defaultdict(list) for word in words: length_dict[len(word)].append(word) return list(length_dict.values()) words = ["hello", "world", "python", "code", "AI"] print(group_by_length(words))
Output:
[['hello', 'world'], ['python'], ['code', 'AI']]
This snippet starts by importing defaultdict from collections. The group_by_length()
function iterates over the list of words, using the word’s length as a key and appending the word to the corresponding list in the dictionary. Finally, it returns the dictionary’s values as a list of lists, effectively grouping the strings by their size.
Method 2: Using Groupby from itertools
The itertools.groupby
function can group items by their length after sorting the input. This method requires sorted input but is elegant and concise. It works best when the order of the groups is also of interest.
Here’s an example:
from itertools import groupby words = ["code", "AI", "hello", "world", "python"] sorted_words = sorted(words, key=len) grouped_words = [list(group) for _, group in groupby(sorted_words, key=len)] print(grouped_words)
Output:
[['AI'], ['code'], ['hello', 'world'], ['python']]
After sorting the words by length, groupby
is applied with the key function len
to group them. The list comprehension iterates over the groups, creating a list of words for each group. The output is slightly different from Method 1 in terms of ordering as it reflects the sorted nature of the input.
Method 3: Using a Simple For Loop
A straightforward way to group strings by size is using a for loop and a dictionary. This method requires no imports and is easily understood by beginners.
Here’s an example:
words = ["hello", "world", "python", "code", "AI"] grouped_words = {} for word in words: grouped_words.setdefault(len(word), []).append(word) print(list(grouped_words.values()))
Output:
[['hello', 'world'], ['python'], ['code', 'AI']]
This code creates an empty dictionary and iterates through the list of words, using the setdefault
method to append words to lists keyed by their length. The list of lists is then obtained by retrieving the dictionary values.
Method 4: Using List Comprehension and set
This method involves using a set to obtain the unique lengths of the strings and then a list comprehension to group them. It is a more Pythonic and compact approach, making good use of list comprehensions.
Here’s an example:
words = ["hello", "world", "python", "code", "AI"] unique_lengths = set(map(len, words)) grouped_words = [[word for word in words if len(word) == size] for size in unique_lengths] print(grouped_words)
Output:
[['code', 'AI'], ['hello', 'world'], ['python']]
This snippet first maps each word to its length and converts the result to a set, providing unique sizes. It then uses a nested list comprehension to build lists of words for each size.
Bonus One-Liner Method 5: Using a Lambda Function and sorted
This bonus one-liner solution exploits a lambda function within the sorted method to group words by their length in a concise expression, making it very compact but potentially less readable.
Here’s an example:
words = ["hello", "world", "python", "code", "AI"] print(sorted(words, key=len))
Output:
['AI', 'code', 'hello', 'world', 'python']
The one-liner uses sorted with a key set as the length of the strings, so it doesn’t return the groups but rather a list ordered by string lengths. To get the groups, further processing is required (e.g., using Method 2 afterward).
Summary/Discussion
- Method 1: Using defaultdict from Collections. Strengths: Automatically handles key initialization. Weaknesses: Requires an import from collections.
- Method 2: Using Groupby from itertools. Strengths: Elegant and concise when ordered groups are needed. Weaknesses: Requires sorting of the list beforehand.
- Method 3: Using a Simple For Loop. Strengths: Easy to understand and requires no import. Weaknesses: More verbose than other methods.
- Method 4: Using List Comprehension and set. Strengths: Pythonic and compact. Weaknesses: Could be less performance-efficient due to multiple iterations over the list.
- Bonus Method 5: One-Liner Using a Lambda Function and sorted. Strengths: Extremely compact. Weaknesses: Less readable and requires additional processing to group by size.
March 10, 2024 at 04:36AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
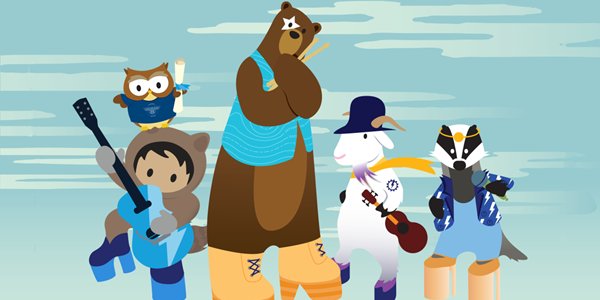
Post a Comment