5 Best Ways to Convert a Single Pandas Series into a String : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with pandas in Python, a common task is to convert a Series object—a one-dimensional array holding any data type—into a single string representation. This can be necessary for data serialization, logging, or simply for generating human-readable reports. For example, given a pandas Series with the values {‘apple’, ‘banana’, ‘cherry’}, the desired output would be a single string like “apple, banana, cherry”.
Method 1: Using Series.str.cat()
The Series.str.cat()
method in pandas is specifically designed for concatenating strings. It works seamlessly with Series objects containing string (object) data. This method is straightforward and can incorporate delimiters, giving you flexibility in formatting the output string.
Here’s an example:
import pandas as pd # Create a simple series fruits_series = pd.Series(['apple', 'banana', 'cherry']) # Use the str.cat() method to concatenate into a string fruits_string = fruits_series.str.cat(sep=', ') print(fruits_string)
Output: apple, banana, cherry
This code snippet creates a pandas Series called fruits_series
containing three fruit names. It then uses the str.cat()
method with a comma followed by a space as the separator to join all the Series elements into a single string, which is printed out.
Method 2: Using join()
Function
The built-in Python function join()
is an effective way to join a sequence of strings into a single string. In the context of pandas, you can apply join()
directly to the values of the Series after converting them to a list, which allows the method to handle non-string data types by converting them to strings automatically during joining.
Here’s an example:
import pandas as pd # Create a simple series fruits_series = pd.Series(['apple', 'banana', 'cherry']) # Use join() on the list of series values fruits_string = ', '.join(fruits_series.astype(str)) print(fruits_string)
Output: apple, banana, cherry
In this example, we first cast our Series fruits_series
to string type to ensure that all elements are strings. We then call the join()
function with a string ‘, ‘ that specifies the separator, and we join all the elements of the series converted to a list. The result is a single string with all elements concatenated, displayed by the print
function.
Method 3: Using Series.apply()
The Series.apply()
method is extremely versatile, allowing you to run a custom function on each element of the Series. This can be used to explicitly convert each element to string and then apply the join()
function to concatenate the results.
Here’s an example:
import pandas as pd # Create a simple series fruits_series = pd.Series(['apple', 'banana', 'cherry']) # Convert each element to string and concatenate fruits_string = ''.join(fruits_series.apply(str)) print(fruits_string)
Output: applebananacherry
This code leverages the apply()
function to cast each item in the Series fruits_series
to a string. Then, the join()
method is used without a separator to merge all elements into a single, continuous string. The print
function outputs the concatenated string.
Method 4: Using Series.aggregate()
or Series.agg()
The aggregate()
(or its alias agg()
) method in pandas allows for the application of a single function, or a list of functions, to the entire Series. When used with a string concatenation function, it effectively converts the Series into a string.
Here’s an example:
import pandas as pd # Create a simple series fruits_series = pd.Series(['apple', 'banana', 'cherry']) # Aggregate using a lambda function to concatenate strings fruits_string = fruits_series.agg(lambda x: ', '.join(x.astype(str))) print(fruits_string)
Output: apple, banana, cherry
The agg()
function is used with a lambda function that converts the entire Series into a list of strings and then joins them with a comma and a space as the separator. The final concatenated string is then printed out.
Bonus One-Liner Method 5: Using +
Operator with astype(str)
Python’s +
operator can be used to concatenate strings. By converting each element of a pandas Series to strings using astype(str)
, the +
operator can be applied iteratively to concatenate the entire Series into a single string.
Here’s an example:
import pandas as pd # Create a simple series fruits_series = pd.Series(['apple', 'banana', 'cherry']) # Convert to string and concatenate with the + operator fruits_string = fruits_series.astype(str).sum() print(fruits_string)
Output: applebananacherry
In this one-liner, we convert fruits_series
to a Series of strings and then simply use the sum()
method, which, for string data, defaults to concatenation with no separator. The final string is outputted on the console.
Summary/Discussion
- Method 1: Using
Series.str.cat()
. This method is specific to string objects within a Series. It’s very flexible and allows inclusion of separators. It requires Series data to be string-typed. - Method 2: Using
join()
Function. This is a Python standard for string joining and ensures automatic conversion to strings for non-string data. However, it involves an extra step of converting the Series to a list. - Method 3: Using
Series.apply()
. This method is good for applying more complex operations to Series elements before concatenating. It may not be as efficient as other methods for simple tasks. - Method 4: Using
Series.aggregate()
orSeries.agg()
. Ideal for when you need to apply multiple operations to a Series. The code can be less intuitive than simpler methods. - Bonus Method 5: Using
+
Operator withastype(str)
. This method is great for quick-and-dirty concatenation without separators, but may lack readability for others examining the code.
March 03, 2024 at 02:36AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
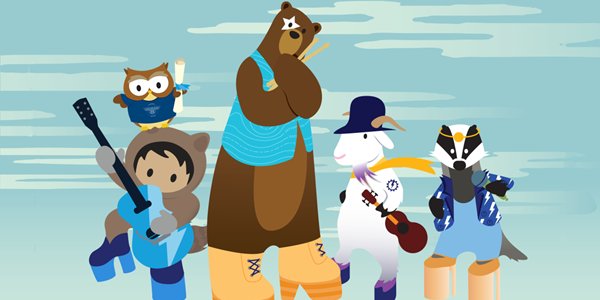
Post a Comment